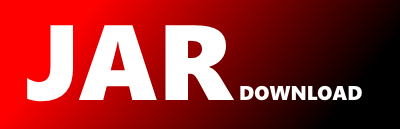
commonMain.aws.sdk.kotlin.services.datazone.model.CreateDomainResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datazone-jvm Show documentation
Show all versions of datazone-jvm Show documentation
The AWS SDK for Kotlin client for DataZone
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.datazone.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateDomainResponse private constructor(builder: Builder) {
/**
* The ARN of the Amazon DataZone domain.
*/
public val arn: kotlin.String? = builder.arn
/**
* The description of the Amazon DataZone domain.
*/
public val description: kotlin.String? = builder.description
/**
* The domain execution role that is created when an Amazon DataZone domain is created. The domain execution role is created in the Amazon Web Services account that houses the Amazon DataZone domain.
*/
public val domainExecutionRole: kotlin.String? = builder.domainExecutionRole
/**
* The identifier of the Amazon DataZone domain.
*/
public val id: kotlin.String = requireNotNull(builder.id) { "A non-null value must be provided for id" }
/**
* The identifier of the Amazon Web Services Key Management Service (KMS) key that is used to encrypt the Amazon DataZone domain, metadata, and reporting data.
*/
public val kmsKeyIdentifier: kotlin.String? = builder.kmsKeyIdentifier
/**
* The name of the Amazon DataZone domain.
*/
public val name: kotlin.String? = builder.name
/**
* The URL of the data portal for this Amazon DataZone domain.
*/
public val portalUrl: kotlin.String? = builder.portalUrl
/**
* The single-sign on configuration of the Amazon DataZone domain.
*/
public val singleSignOn: aws.sdk.kotlin.services.datazone.model.SingleSignOn? = builder.singleSignOn
/**
* The status of the Amazon DataZone domain.
*/
public val status: aws.sdk.kotlin.services.datazone.model.DomainStatus? = builder.status
/**
* The tags specified for the Amazon DataZone domain.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.datazone.model.CreateDomainResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateDomainResponse(")
append("arn=$arn,")
append("description=$description,")
append("domainExecutionRole=$domainExecutionRole,")
append("id=$id,")
append("kmsKeyIdentifier=$kmsKeyIdentifier,")
append("name=$name,")
append("portalUrl=$portalUrl,")
append("singleSignOn=$singleSignOn,")
append("status=$status,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (domainExecutionRole?.hashCode() ?: 0)
result = 31 * result + (id.hashCode())
result = 31 * result + (kmsKeyIdentifier?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (portalUrl?.hashCode() ?: 0)
result = 31 * result + (singleSignOn?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateDomainResponse
if (arn != other.arn) return false
if (description != other.description) return false
if (domainExecutionRole != other.domainExecutionRole) return false
if (id != other.id) return false
if (kmsKeyIdentifier != other.kmsKeyIdentifier) return false
if (name != other.name) return false
if (portalUrl != other.portalUrl) return false
if (singleSignOn != other.singleSignOn) return false
if (status != other.status) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.datazone.model.CreateDomainResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the Amazon DataZone domain.
*/
public var arn: kotlin.String? = null
/**
* The description of the Amazon DataZone domain.
*/
public var description: kotlin.String? = null
/**
* The domain execution role that is created when an Amazon DataZone domain is created. The domain execution role is created in the Amazon Web Services account that houses the Amazon DataZone domain.
*/
public var domainExecutionRole: kotlin.String? = null
/**
* The identifier of the Amazon DataZone domain.
*/
public var id: kotlin.String? = null
/**
* The identifier of the Amazon Web Services Key Management Service (KMS) key that is used to encrypt the Amazon DataZone domain, metadata, and reporting data.
*/
public var kmsKeyIdentifier: kotlin.String? = null
/**
* The name of the Amazon DataZone domain.
*/
public var name: kotlin.String? = null
/**
* The URL of the data portal for this Amazon DataZone domain.
*/
public var portalUrl: kotlin.String? = null
/**
* The single-sign on configuration of the Amazon DataZone domain.
*/
public var singleSignOn: aws.sdk.kotlin.services.datazone.model.SingleSignOn? = null
/**
* The status of the Amazon DataZone domain.
*/
public var status: aws.sdk.kotlin.services.datazone.model.DomainStatus? = null
/**
* The tags specified for the Amazon DataZone domain.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.datazone.model.CreateDomainResponse) : this() {
this.arn = x.arn
this.description = x.description
this.domainExecutionRole = x.domainExecutionRole
this.id = x.id
this.kmsKeyIdentifier = x.kmsKeyIdentifier
this.name = x.name
this.portalUrl = x.portalUrl
this.singleSignOn = x.singleSignOn
this.status = x.status
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.datazone.model.CreateDomainResponse = CreateDomainResponse(this)
/**
* construct an [aws.sdk.kotlin.services.datazone.model.SingleSignOn] inside the given [block]
*/
public fun singleSignOn(block: aws.sdk.kotlin.services.datazone.model.SingleSignOn.Builder.() -> kotlin.Unit) {
this.singleSignOn = aws.sdk.kotlin.services.datazone.model.SingleSignOn.invoke(block)
}
internal fun correctErrors(): Builder {
if (id == null) id = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy