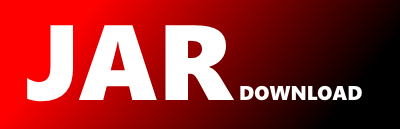
commonMain.aws.sdk.kotlin.services.devicefarm.model.AccountSettings.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.devicefarm.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A container for account-level settings in AWS Device Farm.
*/
public class AccountSettings private constructor(builder: Builder) {
/**
* The AWS account number specified in the `AccountSettings` container.
*/
public val awsAccountNumber: kotlin.String? = builder.awsAccountNumber
/**
* The default number of minutes (at the account level) a test run executes before it times out. The default value is 150 minutes.
*/
public val defaultJobTimeoutMinutes: kotlin.Int? = builder.defaultJobTimeoutMinutes
/**
* The maximum number of minutes a test run executes before it times out.
*/
public val maxJobTimeoutMinutes: kotlin.Int? = builder.maxJobTimeoutMinutes
/**
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an `offering-id:number` pair, where the `offering-id` represents one of the IDs returned by the `ListOfferings` command.
*/
public val maxSlots: Map? = builder.maxSlots
/**
* When set to `true`, for private devices, Device Farm does not sign your app again. For public devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see [Do you modify my app?](http://aws.amazon.com/device-farm/faqs/) in the *AWS Device Farm FAQs*.
*/
public val skipAppResign: kotlin.Boolean? = builder.skipAppResign
/**
* Information about an AWS account's usage of free trial device minutes.
*/
public val trialMinutes: aws.sdk.kotlin.services.devicefarm.model.TrialMinutes? = builder.trialMinutes
/**
* Returns the unmetered devices you have purchased or want to purchase.
*/
public val unmeteredDevices: Map? = builder.unmeteredDevices
/**
* Returns the unmetered remote access devices you have purchased or want to purchase.
*/
public val unmeteredRemoteAccessDevices: Map? = builder.unmeteredRemoteAccessDevices
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.devicefarm.model.AccountSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AccountSettings(")
append("awsAccountNumber=$awsAccountNumber,")
append("defaultJobTimeoutMinutes=$defaultJobTimeoutMinutes,")
append("maxJobTimeoutMinutes=$maxJobTimeoutMinutes,")
append("maxSlots=$maxSlots,")
append("skipAppResign=$skipAppResign,")
append("trialMinutes=$trialMinutes,")
append("unmeteredDevices=$unmeteredDevices,")
append("unmeteredRemoteAccessDevices=$unmeteredRemoteAccessDevices")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = awsAccountNumber?.hashCode() ?: 0
result = 31 * result + (defaultJobTimeoutMinutes ?: 0)
result = 31 * result + (maxJobTimeoutMinutes ?: 0)
result = 31 * result + (maxSlots?.hashCode() ?: 0)
result = 31 * result + (skipAppResign?.hashCode() ?: 0)
result = 31 * result + (trialMinutes?.hashCode() ?: 0)
result = 31 * result + (unmeteredDevices?.hashCode() ?: 0)
result = 31 * result + (unmeteredRemoteAccessDevices?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AccountSettings
if (awsAccountNumber != other.awsAccountNumber) return false
if (defaultJobTimeoutMinutes != other.defaultJobTimeoutMinutes) return false
if (maxJobTimeoutMinutes != other.maxJobTimeoutMinutes) return false
if (maxSlots != other.maxSlots) return false
if (skipAppResign != other.skipAppResign) return false
if (trialMinutes != other.trialMinutes) return false
if (unmeteredDevices != other.unmeteredDevices) return false
if (unmeteredRemoteAccessDevices != other.unmeteredRemoteAccessDevices) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.devicefarm.model.AccountSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The AWS account number specified in the `AccountSettings` container.
*/
public var awsAccountNumber: kotlin.String? = null
/**
* The default number of minutes (at the account level) a test run executes before it times out. The default value is 150 minutes.
*/
public var defaultJobTimeoutMinutes: kotlin.Int? = null
/**
* The maximum number of minutes a test run executes before it times out.
*/
public var maxJobTimeoutMinutes: kotlin.Int? = null
/**
* The maximum number of device slots that the AWS account can purchase. Each maximum is expressed as an `offering-id:number` pair, where the `offering-id` represents one of the IDs returned by the `ListOfferings` command.
*/
public var maxSlots: Map? = null
/**
* When set to `true`, for private devices, Device Farm does not sign your app again. For public devices, Device Farm always signs your apps again.
*
* For more information about how Device Farm re-signs your apps, see [Do you modify my app?](http://aws.amazon.com/device-farm/faqs/) in the *AWS Device Farm FAQs*.
*/
public var skipAppResign: kotlin.Boolean? = null
/**
* Information about an AWS account's usage of free trial device minutes.
*/
public var trialMinutes: aws.sdk.kotlin.services.devicefarm.model.TrialMinutes? = null
/**
* Returns the unmetered devices you have purchased or want to purchase.
*/
public var unmeteredDevices: Map? = null
/**
* Returns the unmetered remote access devices you have purchased or want to purchase.
*/
public var unmeteredRemoteAccessDevices: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.devicefarm.model.AccountSettings) : this() {
this.awsAccountNumber = x.awsAccountNumber
this.defaultJobTimeoutMinutes = x.defaultJobTimeoutMinutes
this.maxJobTimeoutMinutes = x.maxJobTimeoutMinutes
this.maxSlots = x.maxSlots
this.skipAppResign = x.skipAppResign
this.trialMinutes = x.trialMinutes
this.unmeteredDevices = x.unmeteredDevices
this.unmeteredRemoteAccessDevices = x.unmeteredRemoteAccessDevices
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.devicefarm.model.AccountSettings = AccountSettings(this)
/**
* construct an [aws.sdk.kotlin.services.devicefarm.model.TrialMinutes] inside the given [block]
*/
public fun trialMinutes(block: aws.sdk.kotlin.services.devicefarm.model.TrialMinutes.Builder.() -> kotlin.Unit) {
this.trialMinutes = aws.sdk.kotlin.services.devicefarm.model.TrialMinutes.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy