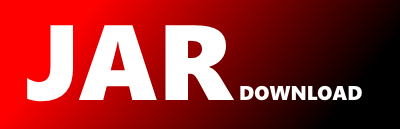
commonMain.aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.devicefarm.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Creates and submits a request to start a remote access session.
*/
public class CreateRemoteAccessSessionRequest private constructor(builder: Builder) {
/**
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the same `clientId` value in each call to `CreateRemoteAccessSession`. This identifier is required only if `remoteDebugEnabled` is set to `true`.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public val clientId: kotlin.String? = builder.clientId
/**
* The configuration information for the remote access session request.
*/
public val configuration: aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionConfiguration? = builder.configuration
/**
* The ARN of the device for which you want to create a remote access session.
*/
public val deviceArn: kotlin.String? = builder.deviceArn
/**
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*/
public val instanceArn: kotlin.String? = builder.instanceArn
/**
* The interaction mode of the remote access session. Valid values are:
* + INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run XCUITest framework-based tests in this mode.
* + NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
* + VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests and watch the screen in this mode.
*/
public val interactionMode: aws.sdk.kotlin.services.devicefarm.model.InteractionMode? = builder.interactionMode
/**
* The name of the remote access session to create.
*/
public val name: kotlin.String? = builder.name
/**
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*/
public val projectArn: kotlin.String? = builder.projectArn
/**
* Set to `true` if you want to access devices remotely for debugging in your remote access session.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public val remoteDebugEnabled: kotlin.Boolean? = builder.remoteDebugEnabled
/**
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public val remoteRecordAppArn: kotlin.String? = builder.remoteRecordAppArn
/**
* Set to `true` to enable remote recording for the remote access session.
*/
public val remoteRecordEnabled: kotlin.Boolean? = builder.remoteRecordEnabled
/**
* When set to `true`, for private devices, Device Farm does not sign your app again. For public devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see [Do you modify my app?](http://aws.amazon.com/device-farm/faqs/)
*/
public val skipAppResign: kotlin.Boolean? = builder.skipAppResign
/**
* Ignored. The public key of the `ssh` key pair you want to use for connecting to remote devices in your remote debugging session. This key is required only if `remoteDebugEnabled` is set to `true`.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public val sshPublicKey: kotlin.String? = builder.sshPublicKey
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateRemoteAccessSessionRequest(")
append("clientId=$clientId,")
append("configuration=$configuration,")
append("deviceArn=$deviceArn,")
append("instanceArn=$instanceArn,")
append("interactionMode=$interactionMode,")
append("name=$name,")
append("projectArn=$projectArn,")
append("remoteDebugEnabled=$remoteDebugEnabled,")
append("remoteRecordAppArn=$remoteRecordAppArn,")
append("remoteRecordEnabled=$remoteRecordEnabled,")
append("skipAppResign=$skipAppResign,")
append("sshPublicKey=$sshPublicKey")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientId?.hashCode() ?: 0
result = 31 * result + (configuration?.hashCode() ?: 0)
result = 31 * result + (deviceArn?.hashCode() ?: 0)
result = 31 * result + (instanceArn?.hashCode() ?: 0)
result = 31 * result + (interactionMode?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (projectArn?.hashCode() ?: 0)
result = 31 * result + (remoteDebugEnabled?.hashCode() ?: 0)
result = 31 * result + (remoteRecordAppArn?.hashCode() ?: 0)
result = 31 * result + (remoteRecordEnabled?.hashCode() ?: 0)
result = 31 * result + (skipAppResign?.hashCode() ?: 0)
result = 31 * result + (sshPublicKey?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateRemoteAccessSessionRequest
if (clientId != other.clientId) return false
if (configuration != other.configuration) return false
if (deviceArn != other.deviceArn) return false
if (instanceArn != other.instanceArn) return false
if (interactionMode != other.interactionMode) return false
if (name != other.name) return false
if (projectArn != other.projectArn) return false
if (remoteDebugEnabled != other.remoteDebugEnabled) return false
if (remoteRecordAppArn != other.remoteRecordAppArn) return false
if (remoteRecordEnabled != other.remoteRecordEnabled) return false
if (skipAppResign != other.skipAppResign) return false
if (sshPublicKey != other.sshPublicKey) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Unique identifier for the client. If you want access to multiple devices on the same client, you should pass the same `clientId` value in each call to `CreateRemoteAccessSession`. This identifier is required only if `remoteDebugEnabled` is set to `true`.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public var clientId: kotlin.String? = null
/**
* The configuration information for the remote access session request.
*/
public var configuration: aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionConfiguration? = null
/**
* The ARN of the device for which you want to create a remote access session.
*/
public var deviceArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the device instance for which you want to create a remote access session.
*/
public var instanceArn: kotlin.String? = null
/**
* The interaction mode of the remote access session. Valid values are:
* + INTERACTIVE: You can interact with the iOS device by viewing, touching, and rotating the screen. You cannot run XCUITest framework-based tests in this mode.
* + NO_VIDEO: You are connected to the device, but cannot interact with it or view the screen. This mode has the fastest test execution speed. You can run XCUITest framework-based tests in this mode.
* + VIDEO_ONLY: You can view the screen, but cannot touch or rotate it. You can run XCUITest framework-based tests and watch the screen in this mode.
*/
public var interactionMode: aws.sdk.kotlin.services.devicefarm.model.InteractionMode? = null
/**
* The name of the remote access session to create.
*/
public var name: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the project for which you want to create a remote access session.
*/
public var projectArn: kotlin.String? = null
/**
* Set to `true` if you want to access devices remotely for debugging in your remote access session.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public var remoteDebugEnabled: kotlin.Boolean? = null
/**
* The Amazon Resource Name (ARN) for the app to be recorded in the remote access session.
*/
public var remoteRecordAppArn: kotlin.String? = null
/**
* Set to `true` to enable remote recording for the remote access session.
*/
public var remoteRecordEnabled: kotlin.Boolean? = null
/**
* When set to `true`, for private devices, Device Farm does not sign your app again. For public devices, Device Farm always signs your apps again.
*
* For more information on how Device Farm modifies your uploads during tests, see [Do you modify my app?](http://aws.amazon.com/device-farm/faqs/)
*/
public var skipAppResign: kotlin.Boolean? = null
/**
* Ignored. The public key of the `ssh` key pair you want to use for connecting to remote devices in your remote debugging session. This key is required only if `remoteDebugEnabled` is set to `true`.
*
* Remote debugging is [no longer supported](https://docs.aws.amazon.com/devicefarm/latest/developerguide/history.html).
*/
public var sshPublicKey: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionRequest) : this() {
this.clientId = x.clientId
this.configuration = x.configuration
this.deviceArn = x.deviceArn
this.instanceArn = x.instanceArn
this.interactionMode = x.interactionMode
this.name = x.name
this.projectArn = x.projectArn
this.remoteDebugEnabled = x.remoteDebugEnabled
this.remoteRecordAppArn = x.remoteRecordAppArn
this.remoteRecordEnabled = x.remoteRecordEnabled
this.skipAppResign = x.skipAppResign
this.sshPublicKey = x.sshPublicKey
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionRequest = CreateRemoteAccessSessionRequest(this)
/**
* construct an [aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionConfiguration] inside the given [block]
*/
public fun configuration(block: aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionConfiguration.Builder.() -> kotlin.Unit) {
this.configuration = aws.sdk.kotlin.services.devicefarm.model.CreateRemoteAccessSessionConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy