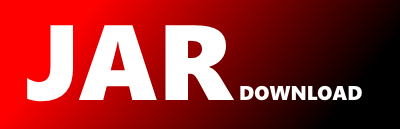
commonMain.aws.sdk.kotlin.services.devicefarm.model.Upload.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.devicefarm.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* An app or a set of one or more tests to upload or that have been uploaded.
*/
public class Upload private constructor(builder: Builder) {
/**
* The upload's ARN.
*/
public val arn: kotlin.String? = builder.arn
/**
* The upload's category. Allowed values include:
* + CURATED: An upload managed by AWS Device Farm.
* + PRIVATE: An upload managed by the AWS Device Farm customer.
*/
public val category: aws.sdk.kotlin.services.devicefarm.model.UploadCategory? = builder.category
/**
* The upload's content type (for example, `application/octet-stream`).
*/
public val contentType: kotlin.String? = builder.contentType
/**
* When the upload was created.
*/
public val created: aws.smithy.kotlin.runtime.time.Instant? = builder.created
/**
* A message about the upload's result.
*/
public val message: kotlin.String? = builder.message
/**
* The upload's metadata. For example, for Android, this contains information that is parsed from the manifest and is displayed in the AWS Device Farm console after the associated app is uploaded.
*/
public val metadata: kotlin.String? = builder.metadata
/**
* The upload's file name.
*/
public val name: kotlin.String? = builder.name
/**
* The upload's status.
*
* Must be one of the following values:
* + FAILED
* + INITIALIZED
* + PROCESSING
* + SUCCEEDED
*/
public val status: aws.sdk.kotlin.services.devicefarm.model.UploadStatus? = builder.status
/**
* The upload's type.
*
* Must be one of the following values:
* + ANDROID_APP
* + IOS_APP
* + WEB_APP
* + EXTERNAL_DATA
* + APPIUM_JAVA_JUNIT_TEST_PACKAGE
* + APPIUM_JAVA_TESTNG_TEST_PACKAGE
* + APPIUM_PYTHON_TEST_PACKAGE
* + APPIUM_NODE_TEST_PACKAGE
* + APPIUM_RUBY_TEST_PACKAGE
* + APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
* + APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
* + APPIUM_WEB_PYTHON_TEST_PACKAGE
* + APPIUM_WEB_NODE_TEST_PACKAGE
* + APPIUM_WEB_RUBY_TEST_PACKAGE
* + INSTRUMENTATION_TEST_PACKAGE
* + XCTEST_TEST_PACKAGE
* + XCTEST_UI_TEST_PACKAGE
* + APPIUM_JAVA_JUNIT_TEST_SPEC
* + APPIUM_JAVA_TESTNG_TEST_SPEC
* + APPIUM_PYTHON_TEST_SPEC
* + APPIUM_NODE_TEST_SPEC
* + APPIUM_RUBY_TEST_SPEC
* + APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
* + APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
* + APPIUM_WEB_PYTHON_TEST_SPEC
* + APPIUM_WEB_NODE_TEST_SPEC
* + APPIUM_WEB_RUBY_TEST_SPEC
* + INSTRUMENTATION_TEST_SPEC
* + XCTEST_UI_TEST_SPEC
*/
public val type: aws.sdk.kotlin.services.devicefarm.model.UploadType? = builder.type
/**
* The presigned Amazon S3 URL that was used to store a file using a PUT request.
*/
public val url: kotlin.String? = builder.url
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.devicefarm.model.Upload = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Upload(")
append("arn=$arn,")
append("category=$category,")
append("contentType=$contentType,")
append("created=$created,")
append("message=$message,")
append("metadata=$metadata,")
append("name=$name,")
append("status=$status,")
append("type=$type,")
append("url=*** Sensitive Data Redacted ***")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (category?.hashCode() ?: 0)
result = 31 * result + (contentType?.hashCode() ?: 0)
result = 31 * result + (created?.hashCode() ?: 0)
result = 31 * result + (message?.hashCode() ?: 0)
result = 31 * result + (metadata?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (url?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Upload
if (arn != other.arn) return false
if (category != other.category) return false
if (contentType != other.contentType) return false
if (created != other.created) return false
if (message != other.message) return false
if (metadata != other.metadata) return false
if (name != other.name) return false
if (status != other.status) return false
if (type != other.type) return false
if (url != other.url) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.devicefarm.model.Upload = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The upload's ARN.
*/
public var arn: kotlin.String? = null
/**
* The upload's category. Allowed values include:
* + CURATED: An upload managed by AWS Device Farm.
* + PRIVATE: An upload managed by the AWS Device Farm customer.
*/
public var category: aws.sdk.kotlin.services.devicefarm.model.UploadCategory? = null
/**
* The upload's content type (for example, `application/octet-stream`).
*/
public var contentType: kotlin.String? = null
/**
* When the upload was created.
*/
public var created: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A message about the upload's result.
*/
public var message: kotlin.String? = null
/**
* The upload's metadata. For example, for Android, this contains information that is parsed from the manifest and is displayed in the AWS Device Farm console after the associated app is uploaded.
*/
public var metadata: kotlin.String? = null
/**
* The upload's file name.
*/
public var name: kotlin.String? = null
/**
* The upload's status.
*
* Must be one of the following values:
* + FAILED
* + INITIALIZED
* + PROCESSING
* + SUCCEEDED
*/
public var status: aws.sdk.kotlin.services.devicefarm.model.UploadStatus? = null
/**
* The upload's type.
*
* Must be one of the following values:
* + ANDROID_APP
* + IOS_APP
* + WEB_APP
* + EXTERNAL_DATA
* + APPIUM_JAVA_JUNIT_TEST_PACKAGE
* + APPIUM_JAVA_TESTNG_TEST_PACKAGE
* + APPIUM_PYTHON_TEST_PACKAGE
* + APPIUM_NODE_TEST_PACKAGE
* + APPIUM_RUBY_TEST_PACKAGE
* + APPIUM_WEB_JAVA_JUNIT_TEST_PACKAGE
* + APPIUM_WEB_JAVA_TESTNG_TEST_PACKAGE
* + APPIUM_WEB_PYTHON_TEST_PACKAGE
* + APPIUM_WEB_NODE_TEST_PACKAGE
* + APPIUM_WEB_RUBY_TEST_PACKAGE
* + INSTRUMENTATION_TEST_PACKAGE
* + XCTEST_TEST_PACKAGE
* + XCTEST_UI_TEST_PACKAGE
* + APPIUM_JAVA_JUNIT_TEST_SPEC
* + APPIUM_JAVA_TESTNG_TEST_SPEC
* + APPIUM_PYTHON_TEST_SPEC
* + APPIUM_NODE_TEST_SPEC
* + APPIUM_RUBY_TEST_SPEC
* + APPIUM_WEB_JAVA_JUNIT_TEST_SPEC
* + APPIUM_WEB_JAVA_TESTNG_TEST_SPEC
* + APPIUM_WEB_PYTHON_TEST_SPEC
* + APPIUM_WEB_NODE_TEST_SPEC
* + APPIUM_WEB_RUBY_TEST_SPEC
* + INSTRUMENTATION_TEST_SPEC
* + XCTEST_UI_TEST_SPEC
*/
public var type: aws.sdk.kotlin.services.devicefarm.model.UploadType? = null
/**
* The presigned Amazon S3 URL that was used to store a file using a PUT request.
*/
public var url: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.devicefarm.model.Upload) : this() {
this.arn = x.arn
this.category = x.category
this.contentType = x.contentType
this.created = x.created
this.message = x.message
this.metadata = x.metadata
this.name = x.name
this.status = x.status
this.type = x.type
this.url = x.url
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.devicefarm.model.Upload = Upload(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy