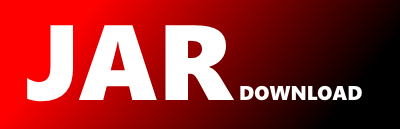
commonMain.aws.sdk.kotlin.services.ecr.model.AuthorizationData.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Kotlin client for ECR
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.ecr.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* An object representing authorization data for an Amazon ECR registry.
*/
public class AuthorizationData private constructor(builder: Builder) {
/**
* A base64-encoded string that contains authorization data for the specified Amazon ECR registry. When the string is decoded, it is presented in the format `user:password` for private registry authentication using `docker login`.
*/
public val authorizationToken: kotlin.String? = builder.authorizationToken
/**
* The Unix time in seconds and milliseconds when the authorization token expires. Authorization tokens are valid for 12 hours.
*/
public val expiresAt: aws.smithy.kotlin.runtime.time.Instant? = builder.expiresAt
/**
* The registry URL to use for this authorization token in a `docker login` command. The Amazon ECR registry URL format is `https://aws_account_id.dkr.ecr.region.amazonaws.com`. For example, `https://012345678910.dkr.ecr.us-east-1.amazonaws.com`..
*/
public val proxyEndpoint: kotlin.String? = builder.proxyEndpoint
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.ecr.model.AuthorizationData = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AuthorizationData(")
append("authorizationToken=$authorizationToken,")
append("expiresAt=$expiresAt,")
append("proxyEndpoint=$proxyEndpoint")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = authorizationToken?.hashCode() ?: 0
result = 31 * result + (expiresAt?.hashCode() ?: 0)
result = 31 * result + (proxyEndpoint?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AuthorizationData
if (authorizationToken != other.authorizationToken) return false
if (expiresAt != other.expiresAt) return false
if (proxyEndpoint != other.proxyEndpoint) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.ecr.model.AuthorizationData = Builder(this).apply(block).build()
public class Builder {
/**
* A base64-encoded string that contains authorization data for the specified Amazon ECR registry. When the string is decoded, it is presented in the format `user:password` for private registry authentication using `docker login`.
*/
public var authorizationToken: kotlin.String? = null
/**
* The Unix time in seconds and milliseconds when the authorization token expires. Authorization tokens are valid for 12 hours.
*/
public var expiresAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The registry URL to use for this authorization token in a `docker login` command. The Amazon ECR registry URL format is `https://aws_account_id.dkr.ecr.region.amazonaws.com`. For example, `https://012345678910.dkr.ecr.us-east-1.amazonaws.com`..
*/
public var proxyEndpoint: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.ecr.model.AuthorizationData) : this() {
this.authorizationToken = x.authorizationToken
this.expiresAt = x.expiresAt
this.proxyEndpoint = x.proxyEndpoint
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.ecr.model.AuthorizationData = AuthorizationData(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy