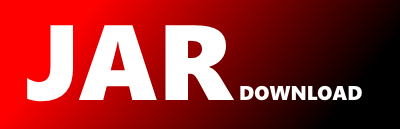
commonMain.aws.sdk.kotlin.services.ecr.model.ImageScanFinding.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Kotlin client for ECR
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.ecr.model
/**
* Contains information about an image scan finding.
*/
public class ImageScanFinding private constructor(builder: Builder) {
/**
* A collection of attributes of the host from which the finding is generated.
*/
public val attributes: List? = builder.attributes
/**
* The description of the finding.
*/
public val description: kotlin.String? = builder.description
/**
* The name associated with the finding, usually a CVE number.
*/
public val name: kotlin.String? = builder.name
/**
* The finding severity.
*/
public val severity: aws.sdk.kotlin.services.ecr.model.FindingSeverity? = builder.severity
/**
* A link containing additional details about the security vulnerability.
*/
public val uri: kotlin.String? = builder.uri
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.ecr.model.ImageScanFinding = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ImageScanFinding(")
append("attributes=$attributes,")
append("description=$description,")
append("name=$name,")
append("severity=$severity,")
append("uri=$uri")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = attributes?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (severity?.hashCode() ?: 0)
result = 31 * result + (uri?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ImageScanFinding
if (attributes != other.attributes) return false
if (description != other.description) return false
if (name != other.name) return false
if (severity != other.severity) return false
if (uri != other.uri) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.ecr.model.ImageScanFinding = Builder(this).apply(block).build()
public class Builder {
/**
* A collection of attributes of the host from which the finding is generated.
*/
public var attributes: List? = null
/**
* The description of the finding.
*/
public var description: kotlin.String? = null
/**
* The name associated with the finding, usually a CVE number.
*/
public var name: kotlin.String? = null
/**
* The finding severity.
*/
public var severity: aws.sdk.kotlin.services.ecr.model.FindingSeverity? = null
/**
* A link containing additional details about the security vulnerability.
*/
public var uri: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.ecr.model.ImageScanFinding) : this() {
this.attributes = x.attributes
this.description = x.description
this.name = x.name
this.severity = x.severity
this.uri = x.uri
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.ecr.model.ImageScanFinding = ImageScanFinding(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy