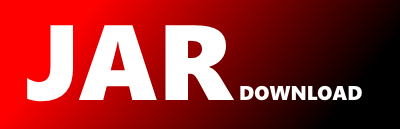
commonMain.aws.sdk.kotlin.services.ecr.model.InvalidLayerPartException.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Kotlin client for ECR
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.ecr.model
import aws.smithy.kotlin.runtime.ServiceErrorMetadata
/**
* The layer part size is not valid, or the first byte specified is not consecutive to the last byte of a previous layer part upload.
*/
public class InvalidLayerPartException private constructor(builder: Builder) : EcrException() {
/**
* The last valid byte received from the layer part upload that is associated with the exception.
*/
public val lastValidByteReceived: kotlin.Long? = builder.lastValidByteReceived
/**
* The error message associated with the exception.
*/
override val message: kotlin.String? = builder.message
/**
* The registry ID associated with the exception.
*/
public val registryId: kotlin.String? = builder.registryId
/**
* The repository name associated with the exception.
*/
public val repositoryName: kotlin.String? = builder.repositoryName
/**
* The upload ID associated with the exception.
*/
public val uploadId: kotlin.String? = builder.uploadId
init {
sdkErrorMetadata.attributes[ServiceErrorMetadata.ErrorType] = ErrorType.Client
}
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.ecr.model.InvalidLayerPartException = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("InvalidLayerPartException(")
append("lastValidByteReceived=$lastValidByteReceived,")
append("message=$message,")
append("registryId=$registryId,")
append("repositoryName=$repositoryName,")
append("uploadId=$uploadId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = lastValidByteReceived?.hashCode() ?: 0
result = 31 * result + (message?.hashCode() ?: 0)
result = 31 * result + (registryId?.hashCode() ?: 0)
result = 31 * result + (repositoryName?.hashCode() ?: 0)
result = 31 * result + (uploadId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as InvalidLayerPartException
if (lastValidByteReceived != other.lastValidByteReceived) return false
if (message != other.message) return false
if (registryId != other.registryId) return false
if (repositoryName != other.repositoryName) return false
if (uploadId != other.uploadId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.ecr.model.InvalidLayerPartException = Builder(this).apply(block).build()
public class Builder {
/**
* The last valid byte received from the layer part upload that is associated with the exception.
*/
public var lastValidByteReceived: kotlin.Long? = null
/**
* The error message associated with the exception.
*/
public var message: kotlin.String? = null
/**
* The registry ID associated with the exception.
*/
public var registryId: kotlin.String? = null
/**
* The repository name associated with the exception.
*/
public var repositoryName: kotlin.String? = null
/**
* The upload ID associated with the exception.
*/
public var uploadId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.ecr.model.InvalidLayerPartException) : this() {
this.lastValidByteReceived = x.lastValidByteReceived
this.message = x.message
this.registryId = x.registryId
this.repositoryName = x.repositoryName
this.uploadId = x.uploadId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.ecr.model.InvalidLayerPartException = InvalidLayerPartException(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy