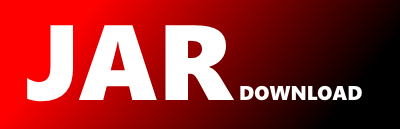
commonMain.aws.sdk.kotlin.services.ecr.model.SetRepositoryPolicyRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecr Show documentation
Show all versions of ecr Show documentation
The AWS Kotlin client for ECR
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.ecr.model
public class SetRepositoryPolicyRequest private constructor(builder: Builder) {
/**
* If the policy you are attempting to set on a repository policy would prevent you from setting another policy in the future, you must force the SetRepositoryPolicy operation. This is intended to prevent accidental repository lock outs.
*/
public val force: kotlin.Boolean = builder.force
/**
* The JSON repository policy text to apply to the repository. For more information, see [Amazon ECR repository policies](https://docs.aws.amazon.com/AmazonECR/latest/userguide/repository-policy-examples.html) in the *Amazon Elastic Container Registry User Guide*.
*/
public val policyText: kotlin.String? = builder.policyText
/**
* The Amazon Web Services account ID associated with the registry that contains the repository. If you do not specify a registry, the default registry is assumed.
*/
public val registryId: kotlin.String? = builder.registryId
/**
* The name of the repository to receive the policy.
*/
public val repositoryName: kotlin.String? = builder.repositoryName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.ecr.model.SetRepositoryPolicyRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SetRepositoryPolicyRequest(")
append("force=$force,")
append("policyText=$policyText,")
append("registryId=$registryId,")
append("repositoryName=$repositoryName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = force.hashCode()
result = 31 * result + (policyText?.hashCode() ?: 0)
result = 31 * result + (registryId?.hashCode() ?: 0)
result = 31 * result + (repositoryName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SetRepositoryPolicyRequest
if (force != other.force) return false
if (policyText != other.policyText) return false
if (registryId != other.registryId) return false
if (repositoryName != other.repositoryName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.ecr.model.SetRepositoryPolicyRequest = Builder(this).apply(block).build()
public class Builder {
/**
* If the policy you are attempting to set on a repository policy would prevent you from setting another policy in the future, you must force the SetRepositoryPolicy operation. This is intended to prevent accidental repository lock outs.
*/
public var force: kotlin.Boolean = false
/**
* The JSON repository policy text to apply to the repository. For more information, see [Amazon ECR repository policies](https://docs.aws.amazon.com/AmazonECR/latest/userguide/repository-policy-examples.html) in the *Amazon Elastic Container Registry User Guide*.
*/
public var policyText: kotlin.String? = null
/**
* The Amazon Web Services account ID associated with the registry that contains the repository. If you do not specify a registry, the default registry is assumed.
*/
public var registryId: kotlin.String? = null
/**
* The name of the repository to receive the policy.
*/
public var repositoryName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.ecr.model.SetRepositoryPolicyRequest) : this() {
this.force = x.force
this.policyText = x.policyText
this.registryId = x.registryId
this.repositoryName = x.repositoryName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.ecr.model.SetRepositoryPolicyRequest = SetRepositoryPolicyRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy