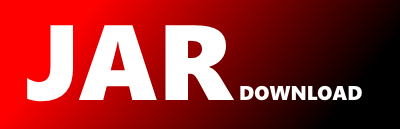
commonMain.aws.sdk.kotlin.services.elastictranscoder.model.CaptionFormat.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elastictranscoder-jvm Show documentation
Show all versions of elastictranscoder-jvm Show documentation
The AWS SDK for Kotlin client for Elastic Transcoder
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.elastictranscoder.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The file format of the output captions. If you leave this value blank, Elastic Transcoder returns an error.
*/
public class CaptionFormat private constructor(builder: Builder) {
/**
* The encryption settings, if any, that you want Elastic Transcoder to apply to your caption formats.
*/
public val encryption: aws.sdk.kotlin.services.elastictranscoder.model.Encryption? = builder.encryption
/**
* The format you specify determines whether Elastic Transcoder generates an embedded or sidecar caption for this output.
* + **Valid Embedded Caption Formats:**
* + **for FLAC**: None
* + **For MP3**: None
* + **For MP4**: mov-text
* + **For MPEG-TS**: None
* + **For ogg**: None
* + **For webm**: None
* + **Valid Sidecar Caption Formats:** Elastic Transcoder supports dfxp (first div element only), scc, srt, and webvtt. If you want ttml or smpte-tt compatible captions, specify dfxp as your output format.
* + **For FMP4**: dfxp
* + **Non-FMP4 outputs**: All sidecar types
* `fmp4` captions have an extension of `.ismt`
*/
public val format: kotlin.String? = builder.format
/**
* The prefix for caption filenames, in the form *description*-`{language}`, where:
* + *description* is a description of the video.
* + `{language}` is a literal value that Elastic Transcoder replaces with the two- or three-letter code for the language of the caption in the output file names.
*
* If you don't include `{language}` in the file name pattern, Elastic Transcoder automatically appends "`{language}`" to the value that you specify for the description. In addition, Elastic Transcoder automatically appends the count to the end of the segment files.
*
* For example, suppose you're transcoding into srt format. When you enter "Sydney-{language}-sunrise", and the language of the captions is English (en), the name of the first caption file is be Sydney-en-sunrise00000.srt.
*/
public val pattern: kotlin.String? = builder.pattern
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.elastictranscoder.model.CaptionFormat = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CaptionFormat(")
append("encryption=$encryption,")
append("format=$format,")
append("pattern=$pattern")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = encryption?.hashCode() ?: 0
result = 31 * result + (format?.hashCode() ?: 0)
result = 31 * result + (pattern?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CaptionFormat
if (encryption != other.encryption) return false
if (format != other.format) return false
if (pattern != other.pattern) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.elastictranscoder.model.CaptionFormat = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The encryption settings, if any, that you want Elastic Transcoder to apply to your caption formats.
*/
public var encryption: aws.sdk.kotlin.services.elastictranscoder.model.Encryption? = null
/**
* The format you specify determines whether Elastic Transcoder generates an embedded or sidecar caption for this output.
* + **Valid Embedded Caption Formats:**
* + **for FLAC**: None
* + **For MP3**: None
* + **For MP4**: mov-text
* + **For MPEG-TS**: None
* + **For ogg**: None
* + **For webm**: None
* + **Valid Sidecar Caption Formats:** Elastic Transcoder supports dfxp (first div element only), scc, srt, and webvtt. If you want ttml or smpte-tt compatible captions, specify dfxp as your output format.
* + **For FMP4**: dfxp
* + **Non-FMP4 outputs**: All sidecar types
* `fmp4` captions have an extension of `.ismt`
*/
public var format: kotlin.String? = null
/**
* The prefix for caption filenames, in the form *description*-`{language}`, where:
* + *description* is a description of the video.
* + `{language}` is a literal value that Elastic Transcoder replaces with the two- or three-letter code for the language of the caption in the output file names.
*
* If you don't include `{language}` in the file name pattern, Elastic Transcoder automatically appends "`{language}`" to the value that you specify for the description. In addition, Elastic Transcoder automatically appends the count to the end of the segment files.
*
* For example, suppose you're transcoding into srt format. When you enter "Sydney-{language}-sunrise", and the language of the captions is English (en), the name of the first caption file is be Sydney-en-sunrise00000.srt.
*/
public var pattern: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.elastictranscoder.model.CaptionFormat) : this() {
this.encryption = x.encryption
this.format = x.format
this.pattern = x.pattern
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.elastictranscoder.model.CaptionFormat = CaptionFormat(this)
/**
* construct an [aws.sdk.kotlin.services.elastictranscoder.model.Encryption] inside the given [block]
*/
public fun encryption(block: aws.sdk.kotlin.services.elastictranscoder.model.Encryption.Builder.() -> kotlin.Unit) {
this.encryption = aws.sdk.kotlin.services.elastictranscoder.model.Encryption.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy