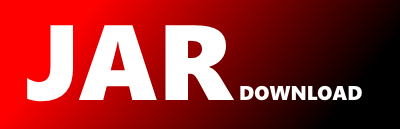
commonMain.aws.sdk.kotlin.services.emrcontainers.model.Endpoint.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.emrcontainers.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* This entity represents the endpoint that is managed by Amazon EMR on EKS.
*/
public class Endpoint private constructor(builder: Builder) {
/**
* The ARN of the endpoint.
*/
public val arn: kotlin.String? = builder.arn
/**
* The certificate ARN of the endpoint. This field is under deprecation and will be removed in future.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public val certificateArn: kotlin.String? = builder.certificateArn
/**
* The certificate generated by emr control plane on customer behalf to secure the managed endpoint.
*/
public val certificateAuthority: aws.sdk.kotlin.services.emrcontainers.model.Certificate? = builder.certificateAuthority
/**
* The configuration settings that are used to override existing configurations for endpoints.
*/
public val configurationOverrides: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides? = builder.configurationOverrides
/**
* The date and time when the endpoint was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The execution role ARN of the endpoint.
*/
public val executionRoleArn: kotlin.String? = builder.executionRoleArn
/**
* The reasons why the endpoint has failed.
*/
public val failureReason: aws.sdk.kotlin.services.emrcontainers.model.FailureReason? = builder.failureReason
/**
* The ID of the endpoint.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the endpoint.
*/
public val name: kotlin.String? = builder.name
/**
* The EMR release version to be used for the endpoint.
*/
public val releaseLabel: kotlin.String? = builder.releaseLabel
/**
* The security group configuration of the endpoint.
*/
public val securityGroup: kotlin.String? = builder.securityGroup
/**
* The server URL of the endpoint.
*/
public val serverUrl: kotlin.String? = builder.serverUrl
/**
* The state of the endpoint.
*/
public val state: aws.sdk.kotlin.services.emrcontainers.model.EndpointState? = builder.state
/**
* Additional details of the endpoint state.
*/
public val stateDetails: kotlin.String? = builder.stateDetails
/**
* The subnet IDs of the endpoint.
*/
public val subnetIds: List? = builder.subnetIds
/**
* The tags of the endpoint.
*/
public val tags: Map? = builder.tags
/**
* The type of the endpoint.
*/
public val type: kotlin.String? = builder.type
/**
* The ID of the endpoint's virtual cluster.
*/
public val virtualClusterId: kotlin.String? = builder.virtualClusterId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.emrcontainers.model.Endpoint = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Endpoint(")
append("arn=$arn,")
append("certificateArn=$certificateArn,")
append("certificateAuthority=$certificateAuthority,")
append("configurationOverrides=$configurationOverrides,")
append("createdAt=$createdAt,")
append("executionRoleArn=$executionRoleArn,")
append("failureReason=$failureReason,")
append("id=$id,")
append("name=$name,")
append("releaseLabel=$releaseLabel,")
append("securityGroup=$securityGroup,")
append("serverUrl=$serverUrl,")
append("state=$state,")
append("stateDetails=$stateDetails,")
append("subnetIds=$subnetIds,")
append("tags=$tags,")
append("type=$type,")
append("virtualClusterId=$virtualClusterId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (certificateArn?.hashCode() ?: 0)
result = 31 * result + (certificateAuthority?.hashCode() ?: 0)
result = 31 * result + (configurationOverrides?.hashCode() ?: 0)
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (executionRoleArn?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (releaseLabel?.hashCode() ?: 0)
result = 31 * result + (securityGroup?.hashCode() ?: 0)
result = 31 * result + (serverUrl?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (stateDetails?.hashCode() ?: 0)
result = 31 * result + (subnetIds?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (virtualClusterId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Endpoint
if (arn != other.arn) return false
if (certificateArn != other.certificateArn) return false
if (certificateAuthority != other.certificateAuthority) return false
if (configurationOverrides != other.configurationOverrides) return false
if (createdAt != other.createdAt) return false
if (executionRoleArn != other.executionRoleArn) return false
if (failureReason != other.failureReason) return false
if (id != other.id) return false
if (name != other.name) return false
if (releaseLabel != other.releaseLabel) return false
if (securityGroup != other.securityGroup) return false
if (serverUrl != other.serverUrl) return false
if (state != other.state) return false
if (stateDetails != other.stateDetails) return false
if (subnetIds != other.subnetIds) return false
if (tags != other.tags) return false
if (type != other.type) return false
if (virtualClusterId != other.virtualClusterId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.emrcontainers.model.Endpoint = Builder(this).apply(block).build()
public class Builder {
/**
* The ARN of the endpoint.
*/
public var arn: kotlin.String? = null
/**
* The certificate ARN of the endpoint. This field is under deprecation and will be removed in future.
*/
@Deprecated("No longer recommended for use. See AWS API documentation for more details.")
public var certificateArn: kotlin.String? = null
/**
* The certificate generated by emr control plane on customer behalf to secure the managed endpoint.
*/
public var certificateAuthority: aws.sdk.kotlin.services.emrcontainers.model.Certificate? = null
/**
* The configuration settings that are used to override existing configurations for endpoints.
*/
public var configurationOverrides: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides? = null
/**
* The date and time when the endpoint was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The execution role ARN of the endpoint.
*/
public var executionRoleArn: kotlin.String? = null
/**
* The reasons why the endpoint has failed.
*/
public var failureReason: aws.sdk.kotlin.services.emrcontainers.model.FailureReason? = null
/**
* The ID of the endpoint.
*/
public var id: kotlin.String? = null
/**
* The name of the endpoint.
*/
public var name: kotlin.String? = null
/**
* The EMR release version to be used for the endpoint.
*/
public var releaseLabel: kotlin.String? = null
/**
* The security group configuration of the endpoint.
*/
public var securityGroup: kotlin.String? = null
/**
* The server URL of the endpoint.
*/
public var serverUrl: kotlin.String? = null
/**
* The state of the endpoint.
*/
public var state: aws.sdk.kotlin.services.emrcontainers.model.EndpointState? = null
/**
* Additional details of the endpoint state.
*/
public var stateDetails: kotlin.String? = null
/**
* The subnet IDs of the endpoint.
*/
public var subnetIds: List? = null
/**
* The tags of the endpoint.
*/
public var tags: Map? = null
/**
* The type of the endpoint.
*/
public var type: kotlin.String? = null
/**
* The ID of the endpoint's virtual cluster.
*/
public var virtualClusterId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.emrcontainers.model.Endpoint) : this() {
this.arn = x.arn
this.certificateArn = x.certificateArn
this.certificateAuthority = x.certificateAuthority
this.configurationOverrides = x.configurationOverrides
this.createdAt = x.createdAt
this.executionRoleArn = x.executionRoleArn
this.failureReason = x.failureReason
this.id = x.id
this.name = x.name
this.releaseLabel = x.releaseLabel
this.securityGroup = x.securityGroup
this.serverUrl = x.serverUrl
this.state = x.state
this.stateDetails = x.stateDetails
this.subnetIds = x.subnetIds
this.tags = x.tags
this.type = x.type
this.virtualClusterId = x.virtualClusterId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.emrcontainers.model.Endpoint = Endpoint(this)
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.Certificate] inside the given [block]
*/
public fun certificateAuthority(block: aws.sdk.kotlin.services.emrcontainers.model.Certificate.Builder.() -> kotlin.Unit) {
this.certificateAuthority = aws.sdk.kotlin.services.emrcontainers.model.Certificate.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides] inside the given [block]
*/
public fun configurationOverrides(block: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides.Builder.() -> kotlin.Unit) {
this.configurationOverrides = aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy