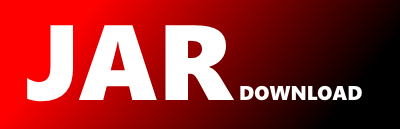
commonMain.aws.sdk.kotlin.services.emrcontainers.model.JobRun.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emrcontainers-jvm Show documentation
Show all versions of emrcontainers-jvm Show documentation
The AWS Kotlin client for EMR containers
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.emrcontainers.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* This entity describes a job run. A job run is a unit of work, such as a Spark jar, PySpark script, or SparkSQL query, that you submit to Amazon EMR on EKS.
*/
public class JobRun private constructor(builder: Builder) {
/**
* The ARN of job run.
*/
public val arn: kotlin.String? = builder.arn
/**
* The client token used to start a job run.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The configuration settings that are used to override default configuration.
*/
public val configurationOverrides: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides? = builder.configurationOverrides
/**
* The date and time when the job run was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The user who created the job run.
*/
public val createdBy: kotlin.String? = builder.createdBy
/**
* The execution role ARN of the job run.
*/
public val executionRoleArn: kotlin.String? = builder.executionRoleArn
/**
* The reasons why the job run has failed.
*/
public val failureReason: aws.sdk.kotlin.services.emrcontainers.model.FailureReason? = builder.failureReason
/**
* The date and time when the job run has finished.
*/
public val finishedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.finishedAt
/**
* The ID of the job run.
*/
public val id: kotlin.String? = builder.id
/**
* Parameters of job driver for the job run.
*/
public val jobDriver: aws.sdk.kotlin.services.emrcontainers.model.JobDriver? = builder.jobDriver
/**
* The name of the job run.
*/
public val name: kotlin.String? = builder.name
/**
* The release version of Amazon EMR.
*/
public val releaseLabel: kotlin.String? = builder.releaseLabel
/**
* The configuration of the retry policy that the job runs on.
*/
public val retryPolicyConfiguration: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyConfiguration? = builder.retryPolicyConfiguration
/**
* The current status of the retry policy executed on the job.
*/
public val retryPolicyExecution: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyExecution? = builder.retryPolicyExecution
/**
* The state of the job run.
*/
public val state: aws.sdk.kotlin.services.emrcontainers.model.JobRunState? = builder.state
/**
* Additional details of the job run state.
*/
public val stateDetails: kotlin.String? = builder.stateDetails
/**
* The assigned tags of the job run.
*/
public val tags: Map? = builder.tags
/**
* The ID of the job run's virtual cluster.
*/
public val virtualClusterId: kotlin.String? = builder.virtualClusterId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.emrcontainers.model.JobRun = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("JobRun(")
append("arn=$arn,")
append("clientToken=$clientToken,")
append("configurationOverrides=$configurationOverrides,")
append("createdAt=$createdAt,")
append("createdBy=$createdBy,")
append("executionRoleArn=$executionRoleArn,")
append("failureReason=$failureReason,")
append("finishedAt=$finishedAt,")
append("id=$id,")
append("jobDriver=$jobDriver,")
append("name=$name,")
append("releaseLabel=$releaseLabel,")
append("retryPolicyConfiguration=$retryPolicyConfiguration,")
append("retryPolicyExecution=$retryPolicyExecution,")
append("state=$state,")
append("stateDetails=$stateDetails,")
append("tags=$tags,")
append("virtualClusterId=$virtualClusterId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (clientToken?.hashCode() ?: 0)
result = 31 * result + (configurationOverrides?.hashCode() ?: 0)
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (createdBy?.hashCode() ?: 0)
result = 31 * result + (executionRoleArn?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (finishedAt?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (jobDriver?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (releaseLabel?.hashCode() ?: 0)
result = 31 * result + (retryPolicyConfiguration?.hashCode() ?: 0)
result = 31 * result + (retryPolicyExecution?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (stateDetails?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (virtualClusterId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as JobRun
if (arn != other.arn) return false
if (clientToken != other.clientToken) return false
if (configurationOverrides != other.configurationOverrides) return false
if (createdAt != other.createdAt) return false
if (createdBy != other.createdBy) return false
if (executionRoleArn != other.executionRoleArn) return false
if (failureReason != other.failureReason) return false
if (finishedAt != other.finishedAt) return false
if (id != other.id) return false
if (jobDriver != other.jobDriver) return false
if (name != other.name) return false
if (releaseLabel != other.releaseLabel) return false
if (retryPolicyConfiguration != other.retryPolicyConfiguration) return false
if (retryPolicyExecution != other.retryPolicyExecution) return false
if (state != other.state) return false
if (stateDetails != other.stateDetails) return false
if (tags != other.tags) return false
if (virtualClusterId != other.virtualClusterId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.emrcontainers.model.JobRun = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of job run.
*/
public var arn: kotlin.String? = null
/**
* The client token used to start a job run.
*/
public var clientToken: kotlin.String? = null
/**
* The configuration settings that are used to override default configuration.
*/
public var configurationOverrides: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides? = null
/**
* The date and time when the job run was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The user who created the job run.
*/
public var createdBy: kotlin.String? = null
/**
* The execution role ARN of the job run.
*/
public var executionRoleArn: kotlin.String? = null
/**
* The reasons why the job run has failed.
*/
public var failureReason: aws.sdk.kotlin.services.emrcontainers.model.FailureReason? = null
/**
* The date and time when the job run has finished.
*/
public var finishedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the job run.
*/
public var id: kotlin.String? = null
/**
* Parameters of job driver for the job run.
*/
public var jobDriver: aws.sdk.kotlin.services.emrcontainers.model.JobDriver? = null
/**
* The name of the job run.
*/
public var name: kotlin.String? = null
/**
* The release version of Amazon EMR.
*/
public var releaseLabel: kotlin.String? = null
/**
* The configuration of the retry policy that the job runs on.
*/
public var retryPolicyConfiguration: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyConfiguration? = null
/**
* The current status of the retry policy executed on the job.
*/
public var retryPolicyExecution: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyExecution? = null
/**
* The state of the job run.
*/
public var state: aws.sdk.kotlin.services.emrcontainers.model.JobRunState? = null
/**
* Additional details of the job run state.
*/
public var stateDetails: kotlin.String? = null
/**
* The assigned tags of the job run.
*/
public var tags: Map? = null
/**
* The ID of the job run's virtual cluster.
*/
public var virtualClusterId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.emrcontainers.model.JobRun) : this() {
this.arn = x.arn
this.clientToken = x.clientToken
this.configurationOverrides = x.configurationOverrides
this.createdAt = x.createdAt
this.createdBy = x.createdBy
this.executionRoleArn = x.executionRoleArn
this.failureReason = x.failureReason
this.finishedAt = x.finishedAt
this.id = x.id
this.jobDriver = x.jobDriver
this.name = x.name
this.releaseLabel = x.releaseLabel
this.retryPolicyConfiguration = x.retryPolicyConfiguration
this.retryPolicyExecution = x.retryPolicyExecution
this.state = x.state
this.stateDetails = x.stateDetails
this.tags = x.tags
this.virtualClusterId = x.virtualClusterId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.emrcontainers.model.JobRun = JobRun(this)
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides] inside the given [block]
*/
public fun configurationOverrides(block: aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides.Builder.() -> kotlin.Unit) {
this.configurationOverrides = aws.sdk.kotlin.services.emrcontainers.model.ConfigurationOverrides.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.JobDriver] inside the given [block]
*/
public fun jobDriver(block: aws.sdk.kotlin.services.emrcontainers.model.JobDriver.Builder.() -> kotlin.Unit) {
this.jobDriver = aws.sdk.kotlin.services.emrcontainers.model.JobDriver.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyConfiguration] inside the given [block]
*/
public fun retryPolicyConfiguration(block: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyConfiguration.Builder.() -> kotlin.Unit) {
this.retryPolicyConfiguration = aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyExecution] inside the given [block]
*/
public fun retryPolicyExecution(block: aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyExecution.Builder.() -> kotlin.Unit) {
this.retryPolicyExecution = aws.sdk.kotlin.services.emrcontainers.model.RetryPolicyExecution.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy