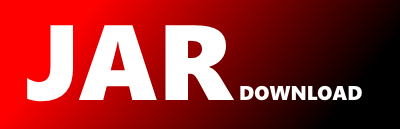
commonMain.aws.sdk.kotlin.services.emrcontainers.model.VirtualCluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emrcontainers-jvm Show documentation
Show all versions of emrcontainers-jvm Show documentation
The AWS Kotlin client for EMR containers
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.emrcontainers.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* This entity describes a virtual cluster. A virtual cluster is a Kubernetes namespace that Amazon EMR is registered with. Amazon EMR uses virtual clusters to run jobs and host endpoints. Multiple virtual clusters can be backed by the same physical cluster. However, each virtual cluster maps to one namespace on an Amazon EKS cluster. Virtual clusters do not create any active resources that contribute to your bill or that require lifecycle management outside the service.
*/
public class VirtualCluster private constructor(builder: Builder) {
/**
* The ARN of the virtual cluster.
*/
public val arn: kotlin.String? = builder.arn
/**
* The container provider of the virtual cluster.
*/
public val containerProvider: aws.sdk.kotlin.services.emrcontainers.model.ContainerProvider? = builder.containerProvider
/**
* The date and time when the virtual cluster is created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The ID of the virtual cluster.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the virtual cluster.
*/
public val name: kotlin.String? = builder.name
/**
* The ID of the security configuration.
*/
public val securityConfigurationId: kotlin.String? = builder.securityConfigurationId
/**
* The state of the virtual cluster.
*/
public val state: aws.sdk.kotlin.services.emrcontainers.model.VirtualClusterState? = builder.state
/**
* The assigned tags of the virtual cluster.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.emrcontainers.model.VirtualCluster = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("VirtualCluster(")
append("arn=$arn,")
append("containerProvider=$containerProvider,")
append("createdAt=$createdAt,")
append("id=$id,")
append("name=$name,")
append("securityConfigurationId=$securityConfigurationId,")
append("state=$state,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (containerProvider?.hashCode() ?: 0)
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (securityConfigurationId?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as VirtualCluster
if (arn != other.arn) return false
if (containerProvider != other.containerProvider) return false
if (createdAt != other.createdAt) return false
if (id != other.id) return false
if (name != other.name) return false
if (securityConfigurationId != other.securityConfigurationId) return false
if (state != other.state) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.emrcontainers.model.VirtualCluster = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ARN of the virtual cluster.
*/
public var arn: kotlin.String? = null
/**
* The container provider of the virtual cluster.
*/
public var containerProvider: aws.sdk.kotlin.services.emrcontainers.model.ContainerProvider? = null
/**
* The date and time when the virtual cluster is created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the virtual cluster.
*/
public var id: kotlin.String? = null
/**
* The name of the virtual cluster.
*/
public var name: kotlin.String? = null
/**
* The ID of the security configuration.
*/
public var securityConfigurationId: kotlin.String? = null
/**
* The state of the virtual cluster.
*/
public var state: aws.sdk.kotlin.services.emrcontainers.model.VirtualClusterState? = null
/**
* The assigned tags of the virtual cluster.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.emrcontainers.model.VirtualCluster) : this() {
this.arn = x.arn
this.containerProvider = x.containerProvider
this.createdAt = x.createdAt
this.id = x.id
this.name = x.name
this.securityConfigurationId = x.securityConfigurationId
this.state = x.state
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.emrcontainers.model.VirtualCluster = VirtualCluster(this)
/**
* construct an [aws.sdk.kotlin.services.emrcontainers.model.ContainerProvider] inside the given [block]
*/
public fun containerProvider(block: aws.sdk.kotlin.services.emrcontainers.model.ContainerProvider.Builder.() -> kotlin.Unit) {
this.containerProvider = aws.sdk.kotlin.services.emrcontainers.model.ContainerProvider.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy