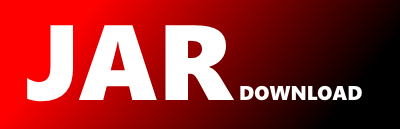
commonMain.aws.sdk.kotlin.services.emrserverless.model.CloudWatchLoggingConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of emrserverless-jvm Show documentation
Show all versions of emrserverless-jvm Show documentation
The AWS SDK for Kotlin client for EMR Serverless
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.emrserverless.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The Amazon CloudWatch configuration for monitoring logs. You can configure your jobs to send log information to CloudWatch.
*/
public class CloudWatchLoggingConfiguration private constructor(builder: Builder) {
/**
* Enables CloudWatch logging.
*/
public val enabled: kotlin.Boolean = requireNotNull(builder.enabled) { "A non-null value must be provided for enabled" }
/**
* The Key Management Service (KMS) key ARN to encrypt the logs that you store in CloudWatch Logs.
*/
public val encryptionKeyArn: kotlin.String? = builder.encryptionKeyArn
/**
* The name of the log group in Amazon CloudWatch Logs where you want to publish your logs.
*/
public val logGroupName: kotlin.String? = builder.logGroupName
/**
* Prefix for the CloudWatch log stream name.
*/
public val logStreamNamePrefix: kotlin.String? = builder.logStreamNamePrefix
/**
* The types of logs that you want to publish to CloudWatch. If you don't specify any log types, driver STDOUT and STDERR logs will be published to CloudWatch Logs by default. For more information including the supported worker types for Hive and Spark, see [Logging for EMR Serverless with CloudWatch](https://docs.aws.amazon.com/emr/latest/EMR-Serverless-UserGuide/logging.html#jobs-log-storage-cw).
* + **Key Valid Values**: `SPARK_DRIVER`, `SPARK_EXECUTOR`, `HIVE_DRIVER`, `TEZ_TASK`
* + **Array Members Valid Values**: `STDOUT`, `STDERR`, `HIVE_LOG`, `TEZ_AM`, `SYSTEM_LOGS`
*/
public val logTypes: Map>? = builder.logTypes
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.emrserverless.model.CloudWatchLoggingConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CloudWatchLoggingConfiguration(")
append("enabled=$enabled,")
append("encryptionKeyArn=$encryptionKeyArn,")
append("logGroupName=$logGroupName,")
append("logStreamNamePrefix=$logStreamNamePrefix,")
append("logTypes=$logTypes")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = enabled.hashCode()
result = 31 * result + (encryptionKeyArn?.hashCode() ?: 0)
result = 31 * result + (logGroupName?.hashCode() ?: 0)
result = 31 * result + (logStreamNamePrefix?.hashCode() ?: 0)
result = 31 * result + (logTypes?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CloudWatchLoggingConfiguration
if (enabled != other.enabled) return false
if (encryptionKeyArn != other.encryptionKeyArn) return false
if (logGroupName != other.logGroupName) return false
if (logStreamNamePrefix != other.logStreamNamePrefix) return false
if (logTypes != other.logTypes) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.emrserverless.model.CloudWatchLoggingConfiguration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Enables CloudWatch logging.
*/
public var enabled: kotlin.Boolean? = null
/**
* The Key Management Service (KMS) key ARN to encrypt the logs that you store in CloudWatch Logs.
*/
public var encryptionKeyArn: kotlin.String? = null
/**
* The name of the log group in Amazon CloudWatch Logs where you want to publish your logs.
*/
public var logGroupName: kotlin.String? = null
/**
* Prefix for the CloudWatch log stream name.
*/
public var logStreamNamePrefix: kotlin.String? = null
/**
* The types of logs that you want to publish to CloudWatch. If you don't specify any log types, driver STDOUT and STDERR logs will be published to CloudWatch Logs by default. For more information including the supported worker types for Hive and Spark, see [Logging for EMR Serverless with CloudWatch](https://docs.aws.amazon.com/emr/latest/EMR-Serverless-UserGuide/logging.html#jobs-log-storage-cw).
* + **Key Valid Values**: `SPARK_DRIVER`, `SPARK_EXECUTOR`, `HIVE_DRIVER`, `TEZ_TASK`
* + **Array Members Valid Values**: `STDOUT`, `STDERR`, `HIVE_LOG`, `TEZ_AM`, `SYSTEM_LOGS`
*/
public var logTypes: Map>? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.emrserverless.model.CloudWatchLoggingConfiguration) : this() {
this.enabled = x.enabled
this.encryptionKeyArn = x.encryptionKeyArn
this.logGroupName = x.logGroupName
this.logStreamNamePrefix = x.logStreamNamePrefix
this.logTypes = x.logTypes
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.emrserverless.model.CloudWatchLoggingConfiguration = CloudWatchLoggingConfiguration(this)
internal fun correctErrors(): Builder {
if (enabled == null) enabled = false
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy