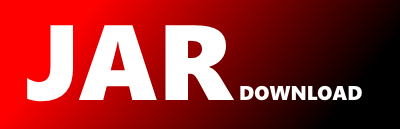
commonMain.aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of entityresolution-jvm Show documentation
Show all versions of entityresolution-jvm Show documentation
The AWS SDK for Kotlin client for EntityResolution
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.entityresolution.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.content.Document
public class GetProviderServiceResponse private constructor(builder: Builder) {
/**
* Specifies whether output data from the provider is anonymized. A value of `TRUE` means the output will be anonymized and you can't relate the data that comes back from the provider to the identifying input. A value of `FALSE` means the output won't be anonymized and you can relate the data that comes back from the provider to your source data.
*/
public val anonymizedOutput: kotlin.Boolean = requireNotNull(builder.anonymizedOutput) { "A non-null value must be provided for anonymizedOutput" }
/**
* Input schema for the provider service.
*/
public val providerComponentSchema: aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema? = builder.providerComponentSchema
/**
* The definition of the provider configuration.
*/
public val providerConfigurationDefinition: aws.smithy.kotlin.runtime.content.Document? = builder.providerConfigurationDefinition
/**
* The required configuration fields to use with the provider service.
*/
public val providerEndpointConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderEndpointConfiguration? = builder.providerEndpointConfiguration
/**
* The definition of the provider entity output.
*/
public val providerEntityOutputDefinition: aws.smithy.kotlin.runtime.content.Document? = builder.providerEntityOutputDefinition
/**
* The provider configuration required for different ID namespace types.
*/
public val providerIdNameSpaceConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration? = builder.providerIdNameSpaceConfiguration
/**
* The Amazon Web Services accounts and the S3 permissions that are required by some providers to create an S3 bucket for intermediate data storage.
*/
public val providerIntermediateDataAccessConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration? = builder.providerIntermediateDataAccessConfiguration
/**
* Provider service job configurations.
*/
public val providerJobConfiguration: aws.smithy.kotlin.runtime.content.Document? = builder.providerJobConfiguration
/**
* The name of the provider. This name is typically the company name.
*/
public val providerName: kotlin.String = requireNotNull(builder.providerName) { "A non-null value must be provided for providerName" }
/**
* The ARN (Amazon Resource Name) that Entity Resolution generated for the provider service.
*/
public val providerServiceArn: kotlin.String = requireNotNull(builder.providerServiceArn) { "A non-null value must be provided for providerServiceArn" }
/**
* The display name of the provider service.
*/
public val providerServiceDisplayName: kotlin.String = requireNotNull(builder.providerServiceDisplayName) { "A non-null value must be provided for providerServiceDisplayName" }
/**
* The name of the product that the provider service provides.
*/
public val providerServiceName: kotlin.String = requireNotNull(builder.providerServiceName) { "A non-null value must be provided for providerServiceName" }
/**
* The type of provider service.
*/
public val providerServiceType: aws.sdk.kotlin.services.entityresolution.model.ServiceType = requireNotNull(builder.providerServiceType) { "A non-null value must be provided for providerServiceType" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetProviderServiceResponse(")
append("anonymizedOutput=$anonymizedOutput,")
append("providerComponentSchema=$providerComponentSchema,")
append("providerConfigurationDefinition=$providerConfigurationDefinition,")
append("providerEndpointConfiguration=$providerEndpointConfiguration,")
append("providerEntityOutputDefinition=$providerEntityOutputDefinition,")
append("providerIdNameSpaceConfiguration=$providerIdNameSpaceConfiguration,")
append("providerIntermediateDataAccessConfiguration=$providerIntermediateDataAccessConfiguration,")
append("providerJobConfiguration=$providerJobConfiguration,")
append("providerName=$providerName,")
append("providerServiceArn=$providerServiceArn,")
append("providerServiceDisplayName=$providerServiceDisplayName,")
append("providerServiceName=$providerServiceName,")
append("providerServiceType=$providerServiceType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = anonymizedOutput.hashCode()
result = 31 * result + (providerComponentSchema?.hashCode() ?: 0)
result = 31 * result + (providerConfigurationDefinition?.hashCode() ?: 0)
result = 31 * result + (providerEndpointConfiguration?.hashCode() ?: 0)
result = 31 * result + (providerEntityOutputDefinition?.hashCode() ?: 0)
result = 31 * result + (providerIdNameSpaceConfiguration?.hashCode() ?: 0)
result = 31 * result + (providerIntermediateDataAccessConfiguration?.hashCode() ?: 0)
result = 31 * result + (providerJobConfiguration?.hashCode() ?: 0)
result = 31 * result + (providerName.hashCode())
result = 31 * result + (providerServiceArn.hashCode())
result = 31 * result + (providerServiceDisplayName.hashCode())
result = 31 * result + (providerServiceName.hashCode())
result = 31 * result + (providerServiceType.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetProviderServiceResponse
if (anonymizedOutput != other.anonymizedOutput) return false
if (providerComponentSchema != other.providerComponentSchema) return false
if (providerConfigurationDefinition != other.providerConfigurationDefinition) return false
if (providerEndpointConfiguration != other.providerEndpointConfiguration) return false
if (providerEntityOutputDefinition != other.providerEntityOutputDefinition) return false
if (providerIdNameSpaceConfiguration != other.providerIdNameSpaceConfiguration) return false
if (providerIntermediateDataAccessConfiguration != other.providerIntermediateDataAccessConfiguration) return false
if (providerJobConfiguration != other.providerJobConfiguration) return false
if (providerName != other.providerName) return false
if (providerServiceArn != other.providerServiceArn) return false
if (providerServiceDisplayName != other.providerServiceDisplayName) return false
if (providerServiceName != other.providerServiceName) return false
if (providerServiceType != other.providerServiceType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Specifies whether output data from the provider is anonymized. A value of `TRUE` means the output will be anonymized and you can't relate the data that comes back from the provider to the identifying input. A value of `FALSE` means the output won't be anonymized and you can relate the data that comes back from the provider to your source data.
*/
public var anonymizedOutput: kotlin.Boolean? = null
/**
* Input schema for the provider service.
*/
public var providerComponentSchema: aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema? = null
/**
* The definition of the provider configuration.
*/
public var providerConfigurationDefinition: aws.smithy.kotlin.runtime.content.Document? = null
/**
* The required configuration fields to use with the provider service.
*/
public var providerEndpointConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderEndpointConfiguration? = null
/**
* The definition of the provider entity output.
*/
public var providerEntityOutputDefinition: aws.smithy.kotlin.runtime.content.Document? = null
/**
* The provider configuration required for different ID namespace types.
*/
public var providerIdNameSpaceConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration? = null
/**
* The Amazon Web Services accounts and the S3 permissions that are required by some providers to create an S3 bucket for intermediate data storage.
*/
public var providerIntermediateDataAccessConfiguration: aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration? = null
/**
* Provider service job configurations.
*/
public var providerJobConfiguration: aws.smithy.kotlin.runtime.content.Document? = null
/**
* The name of the provider. This name is typically the company name.
*/
public var providerName: kotlin.String? = null
/**
* The ARN (Amazon Resource Name) that Entity Resolution generated for the provider service.
*/
public var providerServiceArn: kotlin.String? = null
/**
* The display name of the provider service.
*/
public var providerServiceDisplayName: kotlin.String? = null
/**
* The name of the product that the provider service provides.
*/
public var providerServiceName: kotlin.String? = null
/**
* The type of provider service.
*/
public var providerServiceType: aws.sdk.kotlin.services.entityresolution.model.ServiceType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse) : this() {
this.anonymizedOutput = x.anonymizedOutput
this.providerComponentSchema = x.providerComponentSchema
this.providerConfigurationDefinition = x.providerConfigurationDefinition
this.providerEndpointConfiguration = x.providerEndpointConfiguration
this.providerEntityOutputDefinition = x.providerEntityOutputDefinition
this.providerIdNameSpaceConfiguration = x.providerIdNameSpaceConfiguration
this.providerIntermediateDataAccessConfiguration = x.providerIntermediateDataAccessConfiguration
this.providerJobConfiguration = x.providerJobConfiguration
this.providerName = x.providerName
this.providerServiceArn = x.providerServiceArn
this.providerServiceDisplayName = x.providerServiceDisplayName
this.providerServiceName = x.providerServiceName
this.providerServiceType = x.providerServiceType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse = GetProviderServiceResponse(this)
/**
* construct an [aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema] inside the given [block]
*/
public fun providerComponentSchema(block: aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema.Builder.() -> kotlin.Unit) {
this.providerComponentSchema = aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration] inside the given [block]
*/
public fun providerIdNameSpaceConfiguration(block: aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration.Builder.() -> kotlin.Unit) {
this.providerIdNameSpaceConfiguration = aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration] inside the given [block]
*/
public fun providerIntermediateDataAccessConfiguration(block: aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration.Builder.() -> kotlin.Unit) {
this.providerIntermediateDataAccessConfiguration = aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (anonymizedOutput == null) anonymizedOutput = false
if (providerName == null) providerName = ""
if (providerServiceArn == null) providerServiceArn = ""
if (providerServiceDisplayName == null) providerServiceDisplayName = ""
if (providerServiceName == null) providerServiceName = ""
if (providerServiceType == null) providerServiceType = ServiceType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy