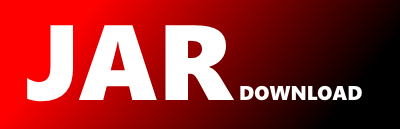
commonMain.aws.sdk.kotlin.services.entityresolution.model.InputSource.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of entityresolution-jvm Show documentation
Show all versions of entityresolution-jvm Show documentation
The AWS SDK for Kotlin client for EntityResolution
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.entityresolution.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* An object containing `InputSourceARN`, `SchemaName`, and `ApplyNormalization`.
*/
public class InputSource private constructor(builder: Builder) {
/**
* Normalizes the attributes defined in the schema in the input data. For example, if an attribute has an `AttributeType` of `PHONE_NUMBER`, and the data in the input table is in a format of 1234567890, Entity Resolution will normalize this field in the output to (123)-456-7890.
*/
public val applyNormalization: kotlin.Boolean? = builder.applyNormalization
/**
* An Glue table ARN for the input source table.
*/
public val inputSourceArn: kotlin.String = requireNotNull(builder.inputSourceArn) { "A non-null value must be provided for inputSourceArn" }
/**
* The name of the schema to be retrieved.
*/
public val schemaName: kotlin.String = requireNotNull(builder.schemaName) { "A non-null value must be provided for schemaName" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.entityresolution.model.InputSource = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("InputSource(")
append("applyNormalization=$applyNormalization,")
append("inputSourceArn=$inputSourceArn,")
append("schemaName=$schemaName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applyNormalization?.hashCode() ?: 0
result = 31 * result + (inputSourceArn.hashCode())
result = 31 * result + (schemaName.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as InputSource
if (applyNormalization != other.applyNormalization) return false
if (inputSourceArn != other.inputSourceArn) return false
if (schemaName != other.schemaName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.entityresolution.model.InputSource = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Normalizes the attributes defined in the schema in the input data. For example, if an attribute has an `AttributeType` of `PHONE_NUMBER`, and the data in the input table is in a format of 1234567890, Entity Resolution will normalize this field in the output to (123)-456-7890.
*/
public var applyNormalization: kotlin.Boolean? = null
/**
* An Glue table ARN for the input source table.
*/
public var inputSourceArn: kotlin.String? = null
/**
* The name of the schema to be retrieved.
*/
public var schemaName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.entityresolution.model.InputSource) : this() {
this.applyNormalization = x.applyNormalization
this.inputSourceArn = x.inputSourceArn
this.schemaName = x.schemaName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.entityresolution.model.InputSource = InputSource(this)
internal fun correctErrors(): Builder {
if (inputSourceArn == null) inputSourceArn = ""
if (schemaName == null) schemaName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy