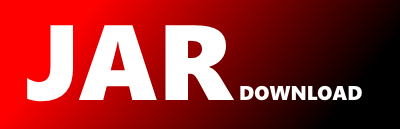
commonMain.aws.sdk.kotlin.services.entityresolution.model.NamespaceRuleBasedProperties.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of entityresolution-jvm Show documentation
Show all versions of entityresolution-jvm Show documentation
The AWS SDK for Kotlin client for EntityResolution
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.entityresolution.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The rule-based properties of an ID namespace. These properties define how the ID namespace can be used in an ID mapping workflow.
*/
public class NamespaceRuleBasedProperties private constructor(builder: Builder) {
/**
* The comparison type. You can either choose `ONE_TO_ONE` or `MANY_TO_MANY` as the `attributeMatchingModel`.
*
* If you choose `MANY_TO_MANY`, the system can match attributes across the sub-types of an attribute type. For example, if the value of the `Email` field of Profile A matches the value of `BusinessEmail` field of Profile B, the two profiles are matched on the `Email` attribute type.
*
* If you choose `ONE_TO_ONE`, the system can only match attributes if the sub-types are an exact match. For example, for the `Email` attribute type, the system will only consider it a match if the value of the `Email` field of Profile A matches the value of the `Email` field of Profile B.
*/
public val attributeMatchingModel: aws.sdk.kotlin.services.entityresolution.model.AttributeMatchingModel? = builder.attributeMatchingModel
/**
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to `ONE_SOURCE_TO_ONE_TARGET`, only one record in the source is matched to one record in the target.
*
* If the value is set to `MANY_SOURCE_TO_ONE_TARGET`, all matching records in the source are matched to one record in the target.
*/
public val recordMatchingModels: List? = builder.recordMatchingModels
/**
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must be compatible.
*/
public val ruleDefinitionTypes: List? = builder.ruleDefinitionTypes
/**
* The rules for the ID namespace.
*/
public val rules: List? = builder.rules
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.entityresolution.model.NamespaceRuleBasedProperties = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NamespaceRuleBasedProperties(")
append("attributeMatchingModel=$attributeMatchingModel,")
append("recordMatchingModels=$recordMatchingModels,")
append("ruleDefinitionTypes=$ruleDefinitionTypes,")
append("rules=$rules")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = attributeMatchingModel?.hashCode() ?: 0
result = 31 * result + (recordMatchingModels?.hashCode() ?: 0)
result = 31 * result + (ruleDefinitionTypes?.hashCode() ?: 0)
result = 31 * result + (rules?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NamespaceRuleBasedProperties
if (attributeMatchingModel != other.attributeMatchingModel) return false
if (recordMatchingModels != other.recordMatchingModels) return false
if (ruleDefinitionTypes != other.ruleDefinitionTypes) return false
if (rules != other.rules) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.entityresolution.model.NamespaceRuleBasedProperties = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The comparison type. You can either choose `ONE_TO_ONE` or `MANY_TO_MANY` as the `attributeMatchingModel`.
*
* If you choose `MANY_TO_MANY`, the system can match attributes across the sub-types of an attribute type. For example, if the value of the `Email` field of Profile A matches the value of `BusinessEmail` field of Profile B, the two profiles are matched on the `Email` attribute type.
*
* If you choose `ONE_TO_ONE`, the system can only match attributes if the sub-types are an exact match. For example, for the `Email` attribute type, the system will only consider it a match if the value of the `Email` field of Profile A matches the value of the `Email` field of Profile B.
*/
public var attributeMatchingModel: aws.sdk.kotlin.services.entityresolution.model.AttributeMatchingModel? = null
/**
* The type of matching record that is allowed to be used in an ID mapping workflow.
*
* If the value is set to `ONE_SOURCE_TO_ONE_TARGET`, only one record in the source is matched to one record in the target.
*
* If the value is set to `MANY_SOURCE_TO_ONE_TARGET`, all matching records in the source are matched to one record in the target.
*/
public var recordMatchingModels: List? = null
/**
* The sets of rules you can use in an ID mapping workflow. The limitations specified for the source and target must be compatible.
*/
public var ruleDefinitionTypes: List? = null
/**
* The rules for the ID namespace.
*/
public var rules: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.entityresolution.model.NamespaceRuleBasedProperties) : this() {
this.attributeMatchingModel = x.attributeMatchingModel
this.recordMatchingModels = x.recordMatchingModels
this.ruleDefinitionTypes = x.ruleDefinitionTypes
this.rules = x.rules
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.entityresolution.model.NamespaceRuleBasedProperties = NamespaceRuleBasedProperties(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy