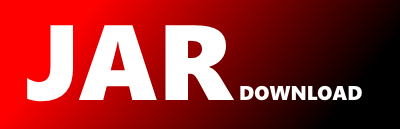
commonMain.aws.sdk.kotlin.services.entityresolution.serde.GetProviderServiceOperationDeserializer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of entityresolution-jvm Show documentation
Show all versions of entityresolution-jvm Show documentation
The AWS SDK for Kotlin client for EntityResolution
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.entityresolution.serde
import aws.sdk.kotlin.services.entityresolution.model.EntityResolutionException
import aws.sdk.kotlin.services.entityresolution.model.GetProviderServiceResponse
import aws.sdk.kotlin.services.entityresolution.model.ProviderComponentSchema
import aws.sdk.kotlin.services.entityresolution.model.ProviderEndpointConfiguration
import aws.sdk.kotlin.services.entityresolution.model.ProviderIdNameSpaceConfiguration
import aws.sdk.kotlin.services.entityresolution.model.ProviderIntermediateDataAccessConfiguration
import aws.sdk.kotlin.services.entityresolution.model.ServiceType
import aws.smithy.kotlin.runtime.awsprotocol.json.RestJsonErrorDeserializer
import aws.smithy.kotlin.runtime.awsprotocol.setAseErrorMetadata
import aws.smithy.kotlin.runtime.awsprotocol.withPayload
import aws.smithy.kotlin.runtime.content.Document
import aws.smithy.kotlin.runtime.http.HttpCall
import aws.smithy.kotlin.runtime.http.isSuccess
import aws.smithy.kotlin.runtime.http.operation.HttpDeserializer
import aws.smithy.kotlin.runtime.operation.ExecutionContext
import aws.smithy.kotlin.runtime.serde.SdkFieldDescriptor
import aws.smithy.kotlin.runtime.serde.SdkObjectDescriptor
import aws.smithy.kotlin.runtime.serde.SerialKind
import aws.smithy.kotlin.runtime.serde.asSdkSerializable
import aws.smithy.kotlin.runtime.serde.deserializeList
import aws.smithy.kotlin.runtime.serde.deserializeMap
import aws.smithy.kotlin.runtime.serde.deserializeStruct
import aws.smithy.kotlin.runtime.serde.field
import aws.smithy.kotlin.runtime.serde.json.JsonDeserializer
import aws.smithy.kotlin.runtime.serde.json.JsonSerialName
import aws.smithy.kotlin.runtime.serde.serializeList
import aws.smithy.kotlin.runtime.serde.serializeMap
import aws.smithy.kotlin.runtime.serde.serializeStruct
internal class GetProviderServiceOperationDeserializer: HttpDeserializer.NonStreaming {
override fun deserialize(context: ExecutionContext, call: HttpCall, payload: ByteArray?): GetProviderServiceResponse {
val response = call.response
if (!response.status.isSuccess()) {
throwGetProviderServiceError(context, call, payload)
}
val builder = GetProviderServiceResponse.Builder()
if (payload != null) {
deserializeGetProviderServiceOperationBody(builder, payload)
}
builder.correctErrors()
return builder.build()
}
}
private fun throwGetProviderServiceError(context: ExecutionContext, call: HttpCall, payload: ByteArray?): kotlin.Nothing {
val wrappedResponse = call.response.withPayload(payload)
val wrappedCall = call.copy(response = wrappedResponse)
val errorDetails = try {
RestJsonErrorDeserializer.deserialize(call.response.headers, payload)
} catch (ex: Exception) {
throw EntityResolutionException("Failed to parse response as 'restJson1' error", ex).also {
setAseErrorMetadata(it, wrappedCall.response, null)
}
}
val ex = when(errorDetails.code) {
"ResourceNotFoundException" -> ResourceNotFoundExceptionDeserializer().deserialize(context, wrappedCall, payload)
"InternalServerException" -> InternalServerExceptionDeserializer().deserialize(context, wrappedCall, payload)
"ValidationException" -> ValidationExceptionDeserializer().deserialize(context, wrappedCall, payload)
"ThrottlingException" -> ThrottlingExceptionDeserializer().deserialize(context, wrappedCall, payload)
"AccessDeniedException" -> AccessDeniedExceptionDeserializer().deserialize(context, wrappedCall, payload)
else -> EntityResolutionException(errorDetails.message)
}
setAseErrorMetadata(ex, wrappedResponse, errorDetails)
throw ex
}
private fun deserializeGetProviderServiceOperationBody(builder: GetProviderServiceResponse.Builder, payload: ByteArray) {
val deserializer = JsonDeserializer(payload)
val ANONYMIZEDOUTPUT_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Boolean, JsonSerialName("anonymizedOutput"))
val PROVIDERCOMPONENTSCHEMA_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("providerComponentSchema"))
val PROVIDERCONFIGURATIONDEFINITION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Document, JsonSerialName("providerConfigurationDefinition"))
val PROVIDERENDPOINTCONFIGURATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("providerEndpointConfiguration"))
val PROVIDERENTITYOUTPUTDEFINITION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Document, JsonSerialName("providerEntityOutputDefinition"))
val PROVIDERIDNAMESPACECONFIGURATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("providerIdNameSpaceConfiguration"))
val PROVIDERINTERMEDIATEDATAACCESSCONFIGURATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("providerIntermediateDataAccessConfiguration"))
val PROVIDERJOBCONFIGURATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Document, JsonSerialName("providerJobConfiguration"))
val PROVIDERNAME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("providerName"))
val PROVIDERSERVICEARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("providerServiceArn"))
val PROVIDERSERVICEDISPLAYNAME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("providerServiceDisplayName"))
val PROVIDERSERVICENAME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("providerServiceName"))
val PROVIDERSERVICETYPE_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Enum, JsonSerialName("providerServiceType"))
val OBJ_DESCRIPTOR = SdkObjectDescriptor.build {
field(ANONYMIZEDOUTPUT_DESCRIPTOR)
field(PROVIDERCOMPONENTSCHEMA_DESCRIPTOR)
field(PROVIDERCONFIGURATIONDEFINITION_DESCRIPTOR)
field(PROVIDERENDPOINTCONFIGURATION_DESCRIPTOR)
field(PROVIDERENTITYOUTPUTDEFINITION_DESCRIPTOR)
field(PROVIDERIDNAMESPACECONFIGURATION_DESCRIPTOR)
field(PROVIDERINTERMEDIATEDATAACCESSCONFIGURATION_DESCRIPTOR)
field(PROVIDERJOBCONFIGURATION_DESCRIPTOR)
field(PROVIDERNAME_DESCRIPTOR)
field(PROVIDERSERVICEARN_DESCRIPTOR)
field(PROVIDERSERVICEDISPLAYNAME_DESCRIPTOR)
field(PROVIDERSERVICENAME_DESCRIPTOR)
field(PROVIDERSERVICETYPE_DESCRIPTOR)
}
deserializer.deserializeStruct(OBJ_DESCRIPTOR) {
loop@while (true) {
when (findNextFieldIndex()) {
ANONYMIZEDOUTPUT_DESCRIPTOR.index -> builder.anonymizedOutput = deserializeBoolean()
PROVIDERCOMPONENTSCHEMA_DESCRIPTOR.index -> builder.providerComponentSchema = deserializeProviderComponentSchemaDocument(deserializer)
PROVIDERCONFIGURATIONDEFINITION_DESCRIPTOR.index -> builder.providerConfigurationDefinition = deserializeDocument()
PROVIDERENDPOINTCONFIGURATION_DESCRIPTOR.index -> builder.providerEndpointConfiguration = deserializeProviderEndpointConfigurationDocument(deserializer)
PROVIDERENTITYOUTPUTDEFINITION_DESCRIPTOR.index -> builder.providerEntityOutputDefinition = deserializeDocument()
PROVIDERIDNAMESPACECONFIGURATION_DESCRIPTOR.index -> builder.providerIdNameSpaceConfiguration = deserializeProviderIdNameSpaceConfigurationDocument(deserializer)
PROVIDERINTERMEDIATEDATAACCESSCONFIGURATION_DESCRIPTOR.index -> builder.providerIntermediateDataAccessConfiguration = deserializeProviderIntermediateDataAccessConfigurationDocument(deserializer)
PROVIDERJOBCONFIGURATION_DESCRIPTOR.index -> builder.providerJobConfiguration = deserializeDocument()
PROVIDERNAME_DESCRIPTOR.index -> builder.providerName = deserializeString()
PROVIDERSERVICEARN_DESCRIPTOR.index -> builder.providerServiceArn = deserializeString()
PROVIDERSERVICEDISPLAYNAME_DESCRIPTOR.index -> builder.providerServiceDisplayName = deserializeString()
PROVIDERSERVICENAME_DESCRIPTOR.index -> builder.providerServiceName = deserializeString()
PROVIDERSERVICETYPE_DESCRIPTOR.index -> builder.providerServiceType = deserializeString().let { ServiceType.fromValue(it) }
null -> break@loop
else -> skipValue()
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy