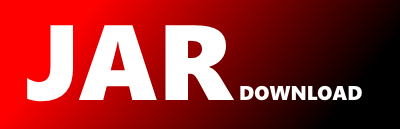
commonMain.aws.sdk.kotlin.services.finspace.DefaultFinspaceClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.finspace.auth.AuthSchemeProviderAdapter
import aws.sdk.kotlin.services.finspace.auth.IdentityProviderConfigAdapter
import aws.sdk.kotlin.services.finspace.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.finspace.model.*
import aws.sdk.kotlin.services.finspace.transform.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.HttpAuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.sdkRequestId
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
import aws.smithy.kotlin.runtime.tracing.withRootTraceSpan
import aws.smithy.kotlin.runtime.util.putIfAbsent
import kotlin.coroutines.coroutineContext
public const val ServiceId: String = "finspace"
public const val ServiceApiVersion: String = "2021-03-12"
public const val SdkVersion: String = "0.28.2-beta"
internal class DefaultFinspaceClient(override val config: FinspaceClient.Config) : FinspaceClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = IdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(HttpAuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "finspace")
}
toMap()
}
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion))
/**
* Create a new FinSpace environment.
*/
override suspend fun createEnvironment(input: CreateEnvironmentRequest): CreateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = CreateEnvironmentOperationSerializer()
deserializer = CreateEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Creates a changeset for a kdb database. A changeset allows you to add and delete existing files by using an ordered list of change requests.
*/
override suspend fun createKxChangeset(input: CreateKxChangesetRequest): CreateKxChangesetResponse {
val op = SdkHttpOperation.build {
serializer = CreateKxChangesetOperationSerializer()
deserializer = CreateKxChangesetOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateKxChangeset"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateKxChangeset-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Creates a new kdb cluster.
*/
override suspend fun createKxCluster(input: CreateKxClusterRequest): CreateKxClusterResponse {
val op = SdkHttpOperation.build {
serializer = CreateKxClusterOperationSerializer()
deserializer = CreateKxClusterOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateKxCluster"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateKxCluster-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Creates a new kdb database in the environment.
*/
override suspend fun createKxDatabase(input: CreateKxDatabaseRequest): CreateKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializer = CreateKxDatabaseOperationSerializer()
deserializer = CreateKxDatabaseOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateKxDatabase"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateKxDatabase-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Creates a managed kdb environment for the account.
*/
override suspend fun createKxEnvironment(input: CreateKxEnvironmentRequest): CreateKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = CreateKxEnvironmentOperationSerializer()
deserializer = CreateKxEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateKxEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateKxEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Creates a user in FinSpace kdb environment with an associated IAM role.
*/
override suspend fun createKxUser(input: CreateKxUserRequest): CreateKxUserResponse {
val op = SdkHttpOperation.build {
serializer = CreateKxUserOperationSerializer()
deserializer = CreateKxUserOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateKxUser"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("CreateKxUser-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Delete an FinSpace environment.
*/
override suspend fun deleteEnvironment(input: DeleteEnvironmentRequest): DeleteEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = DeleteEnvironmentOperationSerializer()
deserializer = DeleteEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DeleteEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("DeleteEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Deletes a kdb cluster.
*/
override suspend fun deleteKxCluster(input: DeleteKxClusterRequest): DeleteKxClusterResponse {
val op = SdkHttpOperation.build {
serializer = DeleteKxClusterOperationSerializer()
deserializer = DeleteKxClusterOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DeleteKxCluster"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("DeleteKxCluster-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Deletes the specified database and all of its associated data. This action is irreversible. You must copy any data out of the database before deleting it if the data is to be retained.
*/
override suspend fun deleteKxDatabase(input: DeleteKxDatabaseRequest): DeleteKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializer = DeleteKxDatabaseOperationSerializer()
deserializer = DeleteKxDatabaseOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DeleteKxDatabase"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("DeleteKxDatabase-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Deletes the kdb environment. This action is irreversible. Deleting a kdb environment will remove all the associated data and any services running in it.
*/
override suspend fun deleteKxEnvironment(input: DeleteKxEnvironmentRequest): DeleteKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = DeleteKxEnvironmentOperationSerializer()
deserializer = DeleteKxEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DeleteKxEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("DeleteKxEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Deletes a user in the specified kdb environment.
*/
override suspend fun deleteKxUser(input: DeleteKxUserRequest): DeleteKxUserResponse {
val op = SdkHttpOperation.build {
serializer = DeleteKxUserOperationSerializer()
deserializer = DeleteKxUserOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DeleteKxUser"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("DeleteKxUser-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns the FinSpace environment object.
*/
override suspend fun getEnvironment(input: GetEnvironmentRequest): GetEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = GetEnvironmentOperationSerializer()
deserializer = GetEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns information about a kdb changeset.
*/
override suspend fun getKxChangeset(input: GetKxChangesetRequest): GetKxChangesetResponse {
val op = SdkHttpOperation.build {
serializer = GetKxChangesetOperationSerializer()
deserializer = GetKxChangesetOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxChangeset"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxChangeset-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Retrieves information about a kdb cluster.
*/
override suspend fun getKxCluster(input: GetKxClusterRequest): GetKxClusterResponse {
val op = SdkHttpOperation.build {
serializer = GetKxClusterOperationSerializer()
deserializer = GetKxClusterOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxCluster"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxCluster-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Retrieves a connection string for a user to connect to a kdb cluster. You must call this API using the same role that you have defined while creating a user.
*/
override suspend fun getKxConnectionString(input: GetKxConnectionStringRequest): GetKxConnectionStringResponse {
val op = SdkHttpOperation.build {
serializer = GetKxConnectionStringOperationSerializer()
deserializer = GetKxConnectionStringOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxConnectionString"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxConnectionString-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns database information for the specified environment ID.
*/
override suspend fun getKxDatabase(input: GetKxDatabaseRequest): GetKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializer = GetKxDatabaseOperationSerializer()
deserializer = GetKxDatabaseOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxDatabase"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxDatabase-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Retrieves all the information for the specified kdb environment.
*/
override suspend fun getKxEnvironment(input: GetKxEnvironmentRequest): GetKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = GetKxEnvironmentOperationSerializer()
deserializer = GetKxEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Retrieves information about the specified kdb user.
*/
override suspend fun getKxUser(input: GetKxUserRequest): GetKxUserResponse {
val op = SdkHttpOperation.build {
serializer = GetKxUserOperationSerializer()
deserializer = GetKxUserOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetKxUser"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetKxUser-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* A list of all of your FinSpace environments.
*/
override suspend fun listEnvironments(input: ListEnvironmentsRequest): ListEnvironmentsResponse {
val op = SdkHttpOperation.build {
serializer = ListEnvironmentsOperationSerializer()
deserializer = ListEnvironmentsOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListEnvironments"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListEnvironments-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns a list of all the changesets for a database.
*/
override suspend fun listKxChangesets(input: ListKxChangesetsRequest): ListKxChangesetsResponse {
val op = SdkHttpOperation.build {
serializer = ListKxChangesetsOperationSerializer()
deserializer = ListKxChangesetsOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxChangesets"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxChangesets-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Lists all the nodes in a kdb cluster.
*/
override suspend fun listKxClusterNodes(input: ListKxClusterNodesRequest): ListKxClusterNodesResponse {
val op = SdkHttpOperation.build {
serializer = ListKxClusterNodesOperationSerializer()
deserializer = ListKxClusterNodesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxClusterNodes"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxClusterNodes-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns a list of clusters.
*/
override suspend fun listKxClusters(input: ListKxClustersRequest): ListKxClustersResponse {
val op = SdkHttpOperation.build {
serializer = ListKxClustersOperationSerializer()
deserializer = ListKxClustersOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxClusters"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxClusters-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns a list of all the databases in the kdb environment.
*/
override suspend fun listKxDatabases(input: ListKxDatabasesRequest): ListKxDatabasesResponse {
val op = SdkHttpOperation.build {
serializer = ListKxDatabasesOperationSerializer()
deserializer = ListKxDatabasesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxDatabases"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxDatabases-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Returns a list of kdb environments created in an account.
*/
override suspend fun listKxEnvironments(input: ListKxEnvironmentsRequest): ListKxEnvironmentsResponse {
val op = SdkHttpOperation.build {
serializer = ListKxEnvironmentsOperationSerializer()
deserializer = ListKxEnvironmentsOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxEnvironments"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxEnvironments-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Lists all the users in a kdb environment.
*/
override suspend fun listKxUsers(input: ListKxUsersRequest): ListKxUsersResponse {
val op = SdkHttpOperation.build {
serializer = ListKxUsersOperationSerializer()
deserializer = ListKxUsersOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListKxUsers"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListKxUsers-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* A list of all tags for a resource.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializer = ListTagsForResourceOperationSerializer()
deserializer = ListTagsForResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListTagsForResource"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("ListTagsForResource-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Adds metadata tags to a FinSpace resource.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializer = TagResourceOperationSerializer()
deserializer = TagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "TagResource"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("TagResource-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Removes metadata tags from a FinSpace resource.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializer = UntagResourceOperationSerializer()
deserializer = UntagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UntagResource"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UntagResource-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Update your FinSpace environment.
*/
override suspend fun updateEnvironment(input: UpdateEnvironmentRequest): UpdateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = UpdateEnvironmentOperationSerializer()
deserializer = UpdateEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Updates the databases mounted on a kdb cluster, which includes the `changesetId` and all the dbPaths to be cached. This API does not allow you to change a database name or add a database if you created a cluster without one.
*
* Using this API you can point a cluster to a different changeset and modify a list of partitions being cached.
*/
override suspend fun updateKxClusterDatabases(input: UpdateKxClusterDatabasesRequest): UpdateKxClusterDatabasesResponse {
val op = SdkHttpOperation.build {
serializer = UpdateKxClusterDatabasesOperationSerializer()
deserializer = UpdateKxClusterDatabasesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateKxClusterDatabases"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateKxClusterDatabases-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Updates information for the given kdb database.
*/
override suspend fun updateKxDatabase(input: UpdateKxDatabaseRequest): UpdateKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializer = UpdateKxDatabaseOperationSerializer()
deserializer = UpdateKxDatabaseOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateKxDatabase"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateKxDatabase-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Updates information for the given kdb environment.
*/
override suspend fun updateKxEnvironment(input: UpdateKxEnvironmentRequest): UpdateKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializer = UpdateKxEnvironmentOperationSerializer()
deserializer = UpdateKxEnvironmentOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateKxEnvironment"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateKxEnvironment-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Updates environment network to connect to your internal network by using a transit gateway. This API supports request to create a transit gateway attachment from FinSpace VPC to your transit gateway ID and create a custom Route-53 outbound resolvers.
*
* Once you send a request to update a network, you cannot change it again. Network update might require termination of any clusters that are running in the existing network.
*/
override suspend fun updateKxEnvironmentNetwork(input: UpdateKxEnvironmentNetworkRequest): UpdateKxEnvironmentNetworkResponse {
val op = SdkHttpOperation.build {
serializer = UpdateKxEnvironmentNetworkOperationSerializer()
deserializer = UpdateKxEnvironmentNetworkOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateKxEnvironmentNetwork"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateKxEnvironmentNetwork-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Updates the user details. You can only update the IAM role associated with a user.
*/
override suspend fun updateKxUser(input: UpdateKxUserRequest): UpdateKxUserResponse {
val op = SdkHttpOperation.build {
serializer = UpdateKxUserOperationSerializer()
deserializer = UpdateKxUserOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UpdateKxUser"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("UpdateKxUser-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private suspend fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(AwsClientOption.Region, config.region)
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "finspace")
ctx.putIfAbsent(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
config.idempotencyTokenProvider?.let { ctx[SdkClientOption.IdempotencyTokenProvider] = it }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy