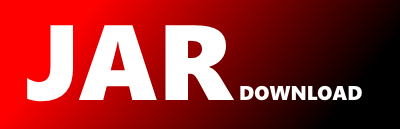
commonMain.aws.sdk.kotlin.services.finspace.model.AutoScalingConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace.model
/**
* The configuration based on which FinSpace will scale in or scale out nodes in your cluster.
*/
public class AutoScalingConfiguration private constructor(builder: Builder) {
/**
* The metric your cluster will track in order to scale in and out. For example, `CPU_UTILIZATION_PERCENTAGE` is the average CPU usage across all the nodes in a cluster.
*/
public val autoScalingMetric: aws.sdk.kotlin.services.finspace.model.AutoScalingMetric? = builder.autoScalingMetric
/**
* The highest number of nodes to scale. This value cannot be greater than 5.
*/
public val maxNodeCount: kotlin.Int? = builder.maxNodeCount
/**
* The desired value of the chosen `autoScalingMetric`. When the metric drops below this value, the cluster will scale in. When the metric goes above this value, the cluster will scale out. You can set the target value between 1 and 100 percent.
*/
public val metricTarget: kotlin.Double? = builder.metricTarget
/**
* The lowest number of nodes to scale. This value must be at least 1 and less than the `maxNodeCount`. If the nodes in a cluster belong to multiple availability zones, then `minNodeCount` must be at least 3.
*/
public val minNodeCount: kotlin.Int? = builder.minNodeCount
/**
* The duration in seconds that FinSpace will wait after a scale in event before initiating another scaling event.
*/
public val scaleInCooldownSeconds: kotlin.Double? = builder.scaleInCooldownSeconds
/**
* The duration in seconds that FinSpace will wait after a scale out event before initiating another scaling event.
*/
public val scaleOutCooldownSeconds: kotlin.Double? = builder.scaleOutCooldownSeconds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspace.model.AutoScalingConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AutoScalingConfiguration(")
append("autoScalingMetric=$autoScalingMetric,")
append("maxNodeCount=$maxNodeCount,")
append("metricTarget=$metricTarget,")
append("minNodeCount=$minNodeCount,")
append("scaleInCooldownSeconds=$scaleInCooldownSeconds,")
append("scaleOutCooldownSeconds=$scaleOutCooldownSeconds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = autoScalingMetric?.hashCode() ?: 0
result = 31 * result + (maxNodeCount ?: 0)
result = 31 * result + (metricTarget?.hashCode() ?: 0)
result = 31 * result + (minNodeCount ?: 0)
result = 31 * result + (scaleInCooldownSeconds?.hashCode() ?: 0)
result = 31 * result + (scaleOutCooldownSeconds?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AutoScalingConfiguration
if (autoScalingMetric != other.autoScalingMetric) return false
if (maxNodeCount != other.maxNodeCount) return false
if (metricTarget != other.metricTarget) return false
if (minNodeCount != other.minNodeCount) return false
if (scaleInCooldownSeconds != other.scaleInCooldownSeconds) return false
if (scaleOutCooldownSeconds != other.scaleOutCooldownSeconds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspace.model.AutoScalingConfiguration = Builder(this).apply(block).build()
public class Builder {
/**
* The metric your cluster will track in order to scale in and out. For example, `CPU_UTILIZATION_PERCENTAGE` is the average CPU usage across all the nodes in a cluster.
*/
public var autoScalingMetric: aws.sdk.kotlin.services.finspace.model.AutoScalingMetric? = null
/**
* The highest number of nodes to scale. This value cannot be greater than 5.
*/
public var maxNodeCount: kotlin.Int? = null
/**
* The desired value of the chosen `autoScalingMetric`. When the metric drops below this value, the cluster will scale in. When the metric goes above this value, the cluster will scale out. You can set the target value between 1 and 100 percent.
*/
public var metricTarget: kotlin.Double? = null
/**
* The lowest number of nodes to scale. This value must be at least 1 and less than the `maxNodeCount`. If the nodes in a cluster belong to multiple availability zones, then `minNodeCount` must be at least 3.
*/
public var minNodeCount: kotlin.Int? = null
/**
* The duration in seconds that FinSpace will wait after a scale in event before initiating another scaling event.
*/
public var scaleInCooldownSeconds: kotlin.Double? = null
/**
* The duration in seconds that FinSpace will wait after a scale out event before initiating another scaling event.
*/
public var scaleOutCooldownSeconds: kotlin.Double? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspace.model.AutoScalingConfiguration) : this() {
this.autoScalingMetric = x.autoScalingMetric
this.maxNodeCount = x.maxNodeCount
this.metricTarget = x.metricTarget
this.minNodeCount = x.minNodeCount
this.scaleInCooldownSeconds = x.scaleInCooldownSeconds
this.scaleOutCooldownSeconds = x.scaleOutCooldownSeconds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspace.model.AutoScalingConfiguration = AutoScalingConfiguration(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy