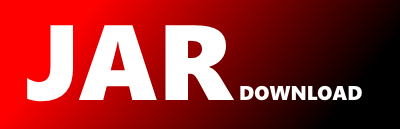
commonMain.aws.sdk.kotlin.services.finspace.model.UpdateKxEnvironmentNetworkResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace.model
import aws.smithy.kotlin.runtime.time.Instant
public class UpdateKxEnvironmentNetworkResponse private constructor(builder: Builder) {
/**
* The identifier of the availability zones where subnets for the environment are created.
*/
public val availabilityZoneIds: List? = builder.availabilityZoneIds
/**
* The unique identifier of the AWS account that is used to create the kdb environment.
*/
public val awsAccountId: kotlin.String? = builder.awsAccountId
/**
* The timestamp at which the kdb environment was created in FinSpace.
*/
public val creationTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTimestamp
/**
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*/
public val customDnsConfiguration: List? = builder.customDnsConfiguration
/**
* A unique identifier for the AWS environment infrastructure account.
*/
public val dedicatedServiceAccountId: kotlin.String? = builder.dedicatedServiceAccountId
/**
* The description of the environment.
*/
public val description: kotlin.String? = builder.description
/**
* The status of DNS configuration.
*/
public val dnsStatus: aws.sdk.kotlin.services.finspace.model.DnsStatus? = builder.dnsStatus
/**
* The ARN identifier of the environment.
*/
public val environmentArn: kotlin.String? = builder.environmentArn
/**
* A unique identifier for the kdb environment.
*/
public val environmentId: kotlin.String? = builder.environmentId
/**
* Specifies the error message that appears if a flow fails.
*/
public val errorMessage: kotlin.String? = builder.errorMessage
/**
* The KMS key ID to encrypt your data in the FinSpace environment.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
/**
* The name of the kdb environment.
*/
public val name: kotlin.String? = builder.name
/**
* The status of the kdb environment.
*/
public val status: aws.sdk.kotlin.services.finspace.model.EnvironmentStatus? = builder.status
/**
* The status of the network configuration.
*/
public val tgwStatus: aws.sdk.kotlin.services.finspace.model.TgwStatus? = builder.tgwStatus
/**
* The structure of the transit gateway and network configuration that is used to connect the kdb environment to an internal network.
*/
public val transitGatewayConfiguration: aws.sdk.kotlin.services.finspace.model.TransitGatewayConfiguration? = builder.transitGatewayConfiguration
/**
* The timestamp at which the kdb environment was updated.
*/
public val updateTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.updateTimestamp
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspace.model.UpdateKxEnvironmentNetworkResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateKxEnvironmentNetworkResponse(")
append("availabilityZoneIds=$availabilityZoneIds,")
append("awsAccountId=$awsAccountId,")
append("creationTimestamp=$creationTimestamp,")
append("customDnsConfiguration=$customDnsConfiguration,")
append("dedicatedServiceAccountId=$dedicatedServiceAccountId,")
append("description=$description,")
append("dnsStatus=$dnsStatus,")
append("environmentArn=$environmentArn,")
append("environmentId=$environmentId,")
append("errorMessage=$errorMessage,")
append("kmsKeyId=$kmsKeyId,")
append("name=$name,")
append("status=$status,")
append("tgwStatus=$tgwStatus,")
append("transitGatewayConfiguration=$transitGatewayConfiguration,")
append("updateTimestamp=$updateTimestamp")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = availabilityZoneIds?.hashCode() ?: 0
result = 31 * result + (awsAccountId?.hashCode() ?: 0)
result = 31 * result + (creationTimestamp?.hashCode() ?: 0)
result = 31 * result + (customDnsConfiguration?.hashCode() ?: 0)
result = 31 * result + (dedicatedServiceAccountId?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (dnsStatus?.hashCode() ?: 0)
result = 31 * result + (environmentArn?.hashCode() ?: 0)
result = 31 * result + (environmentId?.hashCode() ?: 0)
result = 31 * result + (errorMessage?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (tgwStatus?.hashCode() ?: 0)
result = 31 * result + (transitGatewayConfiguration?.hashCode() ?: 0)
result = 31 * result + (updateTimestamp?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateKxEnvironmentNetworkResponse
if (availabilityZoneIds != other.availabilityZoneIds) return false
if (awsAccountId != other.awsAccountId) return false
if (creationTimestamp != other.creationTimestamp) return false
if (customDnsConfiguration != other.customDnsConfiguration) return false
if (dedicatedServiceAccountId != other.dedicatedServiceAccountId) return false
if (description != other.description) return false
if (dnsStatus != other.dnsStatus) return false
if (environmentArn != other.environmentArn) return false
if (environmentId != other.environmentId) return false
if (errorMessage != other.errorMessage) return false
if (kmsKeyId != other.kmsKeyId) return false
if (name != other.name) return false
if (status != other.status) return false
if (tgwStatus != other.tgwStatus) return false
if (transitGatewayConfiguration != other.transitGatewayConfiguration) return false
if (updateTimestamp != other.updateTimestamp) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspace.model.UpdateKxEnvironmentNetworkResponse = Builder(this).apply(block).build()
public class Builder {
/**
* The identifier of the availability zones where subnets for the environment are created.
*/
public var availabilityZoneIds: List? = null
/**
* The unique identifier of the AWS account that is used to create the kdb environment.
*/
public var awsAccountId: kotlin.String? = null
/**
* The timestamp at which the kdb environment was created in FinSpace.
*/
public var creationTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A list of DNS server name and server IP. This is used to set up Route-53 outbound resolvers.
*/
public var customDnsConfiguration: List? = null
/**
* A unique identifier for the AWS environment infrastructure account.
*/
public var dedicatedServiceAccountId: kotlin.String? = null
/**
* The description of the environment.
*/
public var description: kotlin.String? = null
/**
* The status of DNS configuration.
*/
public var dnsStatus: aws.sdk.kotlin.services.finspace.model.DnsStatus? = null
/**
* The ARN identifier of the environment.
*/
public var environmentArn: kotlin.String? = null
/**
* A unique identifier for the kdb environment.
*/
public var environmentId: kotlin.String? = null
/**
* Specifies the error message that appears if a flow fails.
*/
public var errorMessage: kotlin.String? = null
/**
* The KMS key ID to encrypt your data in the FinSpace environment.
*/
public var kmsKeyId: kotlin.String? = null
/**
* The name of the kdb environment.
*/
public var name: kotlin.String? = null
/**
* The status of the kdb environment.
*/
public var status: aws.sdk.kotlin.services.finspace.model.EnvironmentStatus? = null
/**
* The status of the network configuration.
*/
public var tgwStatus: aws.sdk.kotlin.services.finspace.model.TgwStatus? = null
/**
* The structure of the transit gateway and network configuration that is used to connect the kdb environment to an internal network.
*/
public var transitGatewayConfiguration: aws.sdk.kotlin.services.finspace.model.TransitGatewayConfiguration? = null
/**
* The timestamp at which the kdb environment was updated.
*/
public var updateTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspace.model.UpdateKxEnvironmentNetworkResponse) : this() {
this.availabilityZoneIds = x.availabilityZoneIds
this.awsAccountId = x.awsAccountId
this.creationTimestamp = x.creationTimestamp
this.customDnsConfiguration = x.customDnsConfiguration
this.dedicatedServiceAccountId = x.dedicatedServiceAccountId
this.description = x.description
this.dnsStatus = x.dnsStatus
this.environmentArn = x.environmentArn
this.environmentId = x.environmentId
this.errorMessage = x.errorMessage
this.kmsKeyId = x.kmsKeyId
this.name = x.name
this.status = x.status
this.tgwStatus = x.tgwStatus
this.transitGatewayConfiguration = x.transitGatewayConfiguration
this.updateTimestamp = x.updateTimestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspace.model.UpdateKxEnvironmentNetworkResponse = UpdateKxEnvironmentNetworkResponse(this)
/**
* construct an [aws.sdk.kotlin.services.finspace.model.TransitGatewayConfiguration] inside the given [block]
*/
public fun transitGatewayConfiguration(block: aws.sdk.kotlin.services.finspace.model.TransitGatewayConfiguration.Builder.() -> kotlin.Unit) {
this.transitGatewayConfiguration = aws.sdk.kotlin.services.finspace.model.TransitGatewayConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy