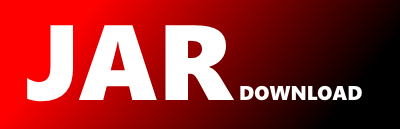
commonMain.aws.sdk.kotlin.services.finspace.DefaultFinspaceClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.finspace.auth.FinspaceAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.finspace.auth.FinspaceIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.finspace.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.finspace.model.*
import aws.sdk.kotlin.services.finspace.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultFinspaceClient(override val config: FinspaceClient.Config) : FinspaceClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = FinspaceIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "finspace")
}
toMap()
}
private val authSchemeAdapter = FinspaceAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.finspace"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Create a new FinSpace environment.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createEnvironment(input: CreateEnvironmentRequest): CreateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateEnvironmentOperationSerializer()
deserializeWith = CreateEnvironmentOperationDeserializer()
operationName = "CreateEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a changeset for a kdb database. A changeset allows you to add and delete existing files by using an ordered list of change requests.
*/
override suspend fun createKxChangeset(input: CreateKxChangesetRequest): CreateKxChangesetResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxChangesetOperationSerializer()
deserializeWith = CreateKxChangesetOperationDeserializer()
operationName = "CreateKxChangeset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new kdb cluster.
*/
override suspend fun createKxCluster(input: CreateKxClusterRequest): CreateKxClusterResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxClusterOperationSerializer()
deserializeWith = CreateKxClusterOperationDeserializer()
operationName = "CreateKxCluster"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new kdb database in the environment.
*/
override suspend fun createKxDatabase(input: CreateKxDatabaseRequest): CreateKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxDatabaseOperationSerializer()
deserializeWith = CreateKxDatabaseOperationDeserializer()
operationName = "CreateKxDatabase"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a snapshot of kdb database with tiered storage capabilities and a pre-warmed cache, ready for mounting on kdb clusters. Dataviews are only available for clusters running on a scaling group. They are not supported on dedicated clusters.
*/
override suspend fun createKxDataview(input: CreateKxDataviewRequest): CreateKxDataviewResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxDataviewOperationSerializer()
deserializeWith = CreateKxDataviewOperationDeserializer()
operationName = "CreateKxDataview"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a managed kdb environment for the account.
*/
override suspend fun createKxEnvironment(input: CreateKxEnvironmentRequest): CreateKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxEnvironmentOperationSerializer()
deserializeWith = CreateKxEnvironmentOperationDeserializer()
operationName = "CreateKxEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new scaling group.
*/
override suspend fun createKxScalingGroup(input: CreateKxScalingGroupRequest): CreateKxScalingGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxScalingGroupOperationSerializer()
deserializeWith = CreateKxScalingGroupOperationDeserializer()
operationName = "CreateKxScalingGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a user in FinSpace kdb environment with an associated IAM role.
*/
override suspend fun createKxUser(input: CreateKxUserRequest): CreateKxUserResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxUserOperationSerializer()
deserializeWith = CreateKxUserOperationDeserializer()
operationName = "CreateKxUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new volume with a specific amount of throughput and storage capacity.
*/
override suspend fun createKxVolume(input: CreateKxVolumeRequest): CreateKxVolumeResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateKxVolumeOperationSerializer()
deserializeWith = CreateKxVolumeOperationDeserializer()
operationName = "CreateKxVolume"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Delete an FinSpace environment.
*/
@Deprecated("This method will be discontinued.")
override suspend fun deleteEnvironment(input: DeleteEnvironmentRequest): DeleteEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteEnvironmentOperationSerializer()
deserializeWith = DeleteEnvironmentOperationDeserializer()
operationName = "DeleteEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a kdb cluster.
*/
override suspend fun deleteKxCluster(input: DeleteKxClusterRequest): DeleteKxClusterResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxClusterOperationSerializer()
deserializeWith = DeleteKxClusterOperationDeserializer()
operationName = "DeleteKxCluster"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified nodes from a cluster.
*/
override suspend fun deleteKxClusterNode(input: DeleteKxClusterNodeRequest): DeleteKxClusterNodeResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxClusterNodeOperationSerializer()
deserializeWith = DeleteKxClusterNodeOperationDeserializer()
operationName = "DeleteKxClusterNode"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified database and all of its associated data. This action is irreversible. You must copy any data out of the database before deleting it if the data is to be retained.
*/
override suspend fun deleteKxDatabase(input: DeleteKxDatabaseRequest): DeleteKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxDatabaseOperationSerializer()
deserializeWith = DeleteKxDatabaseOperationDeserializer()
operationName = "DeleteKxDatabase"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified dataview. Before deleting a dataview, make sure that it is not in use by any cluster.
*/
override suspend fun deleteKxDataview(input: DeleteKxDataviewRequest): DeleteKxDataviewResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxDataviewOperationSerializer()
deserializeWith = DeleteKxDataviewOperationDeserializer()
operationName = "DeleteKxDataview"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the kdb environment. This action is irreversible. Deleting a kdb environment will remove all the associated data and any services running in it.
*/
override suspend fun deleteKxEnvironment(input: DeleteKxEnvironmentRequest): DeleteKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxEnvironmentOperationSerializer()
deserializeWith = DeleteKxEnvironmentOperationDeserializer()
operationName = "DeleteKxEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes the specified scaling group. This action is irreversible. You cannot delete a scaling group until all the clusters running on it have been deleted.
*/
override suspend fun deleteKxScalingGroup(input: DeleteKxScalingGroupRequest): DeleteKxScalingGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxScalingGroupOperationSerializer()
deserializeWith = DeleteKxScalingGroupOperationDeserializer()
operationName = "DeleteKxScalingGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a user in the specified kdb environment.
*/
override suspend fun deleteKxUser(input: DeleteKxUserRequest): DeleteKxUserResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxUserOperationSerializer()
deserializeWith = DeleteKxUserOperationDeserializer()
operationName = "DeleteKxUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a volume. You can only delete a volume if it's not attached to a cluster or a dataview. When a volume is deleted, any data on the volume is lost. This action is irreversible.
*/
override suspend fun deleteKxVolume(input: DeleteKxVolumeRequest): DeleteKxVolumeResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteKxVolumeOperationSerializer()
deserializeWith = DeleteKxVolumeOperationDeserializer()
operationName = "DeleteKxVolume"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the FinSpace environment object.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getEnvironment(input: GetEnvironmentRequest): GetEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = GetEnvironmentOperationSerializer()
deserializeWith = GetEnvironmentOperationDeserializer()
operationName = "GetEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about a kdb changeset.
*/
override suspend fun getKxChangeset(input: GetKxChangesetRequest): GetKxChangesetResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxChangesetOperationSerializer()
deserializeWith = GetKxChangesetOperationDeserializer()
operationName = "GetKxChangeset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves information about a kdb cluster.
*/
override suspend fun getKxCluster(input: GetKxClusterRequest): GetKxClusterResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxClusterOperationSerializer()
deserializeWith = GetKxClusterOperationDeserializer()
operationName = "GetKxCluster"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves a connection string for a user to connect to a kdb cluster. You must call this API using the same role that you have defined while creating a user.
*/
override suspend fun getKxConnectionString(input: GetKxConnectionStringRequest): GetKxConnectionStringResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxConnectionStringOperationSerializer()
deserializeWith = GetKxConnectionStringOperationDeserializer()
operationName = "GetKxConnectionString"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns database information for the specified environment ID.
*/
override suspend fun getKxDatabase(input: GetKxDatabaseRequest): GetKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxDatabaseOperationSerializer()
deserializeWith = GetKxDatabaseOperationDeserializer()
operationName = "GetKxDatabase"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves details of the dataview.
*/
override suspend fun getKxDataview(input: GetKxDataviewRequest): GetKxDataviewResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxDataviewOperationSerializer()
deserializeWith = GetKxDataviewOperationDeserializer()
operationName = "GetKxDataview"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves all the information for the specified kdb environment.
*/
override suspend fun getKxEnvironment(input: GetKxEnvironmentRequest): GetKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxEnvironmentOperationSerializer()
deserializeWith = GetKxEnvironmentOperationDeserializer()
operationName = "GetKxEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves details of a scaling group.
*/
override suspend fun getKxScalingGroup(input: GetKxScalingGroupRequest): GetKxScalingGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxScalingGroupOperationSerializer()
deserializeWith = GetKxScalingGroupOperationDeserializer()
operationName = "GetKxScalingGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves information about the specified kdb user.
*/
override suspend fun getKxUser(input: GetKxUserRequest): GetKxUserResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxUserOperationSerializer()
deserializeWith = GetKxUserOperationDeserializer()
operationName = "GetKxUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves the information about the volume.
*/
override suspend fun getKxVolume(input: GetKxVolumeRequest): GetKxVolumeResponse {
val op = SdkHttpOperation.build {
serializeWith = GetKxVolumeOperationSerializer()
deserializeWith = GetKxVolumeOperationDeserializer()
operationName = "GetKxVolume"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* A list of all of your FinSpace environments.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listEnvironments(input: ListEnvironmentsRequest): ListEnvironmentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListEnvironmentsOperationSerializer()
deserializeWith = ListEnvironmentsOperationDeserializer()
operationName = "ListEnvironments"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of all the changesets for a database.
*/
override suspend fun listKxChangesets(input: ListKxChangesetsRequest): ListKxChangesetsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxChangesetsOperationSerializer()
deserializeWith = ListKxChangesetsOperationDeserializer()
operationName = "ListKxChangesets"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all the nodes in a kdb cluster.
*/
override suspend fun listKxClusterNodes(input: ListKxClusterNodesRequest): ListKxClusterNodesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxClusterNodesOperationSerializer()
deserializeWith = ListKxClusterNodesOperationDeserializer()
operationName = "ListKxClusterNodes"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of clusters.
*/
override suspend fun listKxClusters(input: ListKxClustersRequest): ListKxClustersResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxClustersOperationSerializer()
deserializeWith = ListKxClustersOperationDeserializer()
operationName = "ListKxClusters"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of all the databases in the kdb environment.
*/
override suspend fun listKxDatabases(input: ListKxDatabasesRequest): ListKxDatabasesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxDatabasesOperationSerializer()
deserializeWith = ListKxDatabasesOperationDeserializer()
operationName = "ListKxDatabases"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of all the dataviews in the database.
*/
override suspend fun listKxDataviews(input: ListKxDataviewsRequest): ListKxDataviewsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxDataviewsOperationSerializer()
deserializeWith = ListKxDataviewsOperationDeserializer()
operationName = "ListKxDataviews"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of kdb environments created in an account.
*/
override suspend fun listKxEnvironments(input: ListKxEnvironmentsRequest): ListKxEnvironmentsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxEnvironmentsOperationSerializer()
deserializeWith = ListKxEnvironmentsOperationDeserializer()
operationName = "ListKxEnvironments"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of scaling groups in a kdb environment.
*/
override suspend fun listKxScalingGroups(input: ListKxScalingGroupsRequest): ListKxScalingGroupsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxScalingGroupsOperationSerializer()
deserializeWith = ListKxScalingGroupsOperationDeserializer()
operationName = "ListKxScalingGroups"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all the users in a kdb environment.
*/
override suspend fun listKxUsers(input: ListKxUsersRequest): ListKxUsersResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxUsersOperationSerializer()
deserializeWith = ListKxUsersOperationDeserializer()
operationName = "ListKxUsers"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all the volumes in a kdb environment.
*/
override suspend fun listKxVolumes(input: ListKxVolumesRequest): ListKxVolumesResponse {
val op = SdkHttpOperation.build {
serializeWith = ListKxVolumesOperationSerializer()
deserializeWith = ListKxVolumesOperationDeserializer()
operationName = "ListKxVolumes"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* A list of all tags for a resource.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = ListTagsForResourceOperationSerializer()
deserializeWith = ListTagsForResourceOperationDeserializer()
operationName = "ListTagsForResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Adds metadata tags to a FinSpace resource.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = TagResourceOperationSerializer()
deserializeWith = TagResourceOperationDeserializer()
operationName = "TagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes metadata tags from a FinSpace resource.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializeWith = UntagResourceOperationSerializer()
deserializeWith = UntagResourceOperationDeserializer()
operationName = "UntagResource"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Update your FinSpace environment.
*/
@Deprecated("This method will be discontinued.")
override suspend fun updateEnvironment(input: UpdateEnvironmentRequest): UpdateEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateEnvironmentOperationSerializer()
deserializeWith = UpdateEnvironmentOperationDeserializer()
operationName = "UpdateEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Allows you to update code configuration on a running cluster. By using this API you can update the code, the initialization script path, and the command line arguments for a specific cluster. The configuration that you want to update will override any existing configurations on the cluster.
*/
override suspend fun updateKxClusterCodeConfiguration(input: UpdateKxClusterCodeConfigurationRequest): UpdateKxClusterCodeConfigurationResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxClusterCodeConfigurationOperationSerializer()
deserializeWith = UpdateKxClusterCodeConfigurationOperationDeserializer()
operationName = "UpdateKxClusterCodeConfiguration"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the databases mounted on a kdb cluster, which includes the `changesetId` and all the dbPaths to be cached. This API does not allow you to change a database name or add a database if you created a cluster without one.
*
* Using this API you can point a cluster to a different changeset and modify a list of partitions being cached.
*/
override suspend fun updateKxClusterDatabases(input: UpdateKxClusterDatabasesRequest): UpdateKxClusterDatabasesResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxClusterDatabasesOperationSerializer()
deserializeWith = UpdateKxClusterDatabasesOperationDeserializer()
operationName = "UpdateKxClusterDatabases"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates information for the given kdb database.
*/
override suspend fun updateKxDatabase(input: UpdateKxDatabaseRequest): UpdateKxDatabaseResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxDatabaseOperationSerializer()
deserializeWith = UpdateKxDatabaseOperationDeserializer()
operationName = "UpdateKxDatabase"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the specified dataview. The dataviews get automatically updated when any new changesets are ingested. Each update of the dataview creates a new version, including changeset details and cache configurations
*/
override suspend fun updateKxDataview(input: UpdateKxDataviewRequest): UpdateKxDataviewResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxDataviewOperationSerializer()
deserializeWith = UpdateKxDataviewOperationDeserializer()
operationName = "UpdateKxDataview"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates information for the given kdb environment.
*/
override suspend fun updateKxEnvironment(input: UpdateKxEnvironmentRequest): UpdateKxEnvironmentResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxEnvironmentOperationSerializer()
deserializeWith = UpdateKxEnvironmentOperationDeserializer()
operationName = "UpdateKxEnvironment"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates environment network to connect to your internal network by using a transit gateway. This API supports request to create a transit gateway attachment from FinSpace VPC to your transit gateway ID and create a custom Route-53 outbound resolvers.
*
* Once you send a request to update a network, you cannot change it again. Network update might require termination of any clusters that are running in the existing network.
*/
override suspend fun updateKxEnvironmentNetwork(input: UpdateKxEnvironmentNetworkRequest): UpdateKxEnvironmentNetworkResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxEnvironmentNetworkOperationSerializer()
deserializeWith = UpdateKxEnvironmentNetworkOperationDeserializer()
operationName = "UpdateKxEnvironmentNetwork"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the user details. You can only update the IAM role associated with a user.
*/
override suspend fun updateKxUser(input: UpdateKxUserRequest): UpdateKxUserResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxUserOperationSerializer()
deserializeWith = UpdateKxUserOperationDeserializer()
operationName = "UpdateKxUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates the throughput or capacity of a volume. During the update process, the filesystem might be unavailable for a few minutes. You can retry any operations after the update is complete.
*/
override suspend fun updateKxVolume(input: UpdateKxVolumeRequest): UpdateKxVolumeResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateKxVolumeOperationSerializer()
deserializeWith = UpdateKxVolumeOperationDeserializer()
operationName = "UpdateKxVolume"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(SdkClientOption.IdempotencyTokenProvider, config.idempotencyTokenProvider)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "finspace")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy