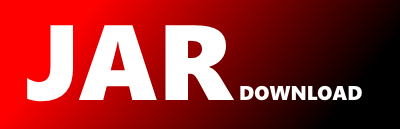
commonMain.aws.sdk.kotlin.services.finspace.model.CreateKxScalingGroupResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class CreateKxScalingGroupResponse private constructor(builder: Builder) {
/**
* The identifier of the availability zones.
*/
public val availabilityZoneId: kotlin.String? = builder.availabilityZoneId
/**
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val createdTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.createdTimestamp
/**
* A unique identifier for the kdb environment, where you create the scaling group.
*/
public val environmentId: kotlin.String? = builder.environmentId
/**
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*/
public val hostType: kotlin.String? = builder.hostType
/**
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val lastModifiedTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTimestamp
/**
* A unique identifier for the kdb scaling group.
*/
public val scalingGroupName: kotlin.String? = builder.scalingGroupName
/**
* The status of scaling group.
* + CREATING – The scaling group creation is in progress.
* + CREATE_FAILED – The scaling group creation has failed.
* + ACTIVE – The scaling group is active.
* + UPDATING – The scaling group is in the process of being updated.
* + UPDATE_FAILED – The update action failed.
* + DELETING – The scaling group is in the process of being deleted.
* + DELETE_FAILED – The system failed to delete the scaling group.
* + DELETED – The scaling group is successfully deleted.
*/
public val status: aws.sdk.kotlin.services.finspace.model.KxScalingGroupStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspace.model.CreateKxScalingGroupResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateKxScalingGroupResponse(")
append("availabilityZoneId=$availabilityZoneId,")
append("createdTimestamp=$createdTimestamp,")
append("environmentId=$environmentId,")
append("hostType=$hostType,")
append("lastModifiedTimestamp=$lastModifiedTimestamp,")
append("scalingGroupName=$scalingGroupName,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = availabilityZoneId?.hashCode() ?: 0
result = 31 * result + (createdTimestamp?.hashCode() ?: 0)
result = 31 * result + (environmentId?.hashCode() ?: 0)
result = 31 * result + (hostType?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTimestamp?.hashCode() ?: 0)
result = 31 * result + (scalingGroupName?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateKxScalingGroupResponse
if (availabilityZoneId != other.availabilityZoneId) return false
if (createdTimestamp != other.createdTimestamp) return false
if (environmentId != other.environmentId) return false
if (hostType != other.hostType) return false
if (lastModifiedTimestamp != other.lastModifiedTimestamp) return false
if (scalingGroupName != other.scalingGroupName) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspace.model.CreateKxScalingGroupResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The identifier of the availability zones.
*/
public var availabilityZoneId: kotlin.String? = null
/**
* The timestamp at which the scaling group was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var createdTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A unique identifier for the kdb environment, where you create the scaling group.
*/
public var environmentId: kotlin.String? = null
/**
* The memory and CPU capabilities of the scaling group host on which FinSpace Managed kdb clusters will be placed.
*/
public var hostType: kotlin.String? = null
/**
* The last time that the scaling group was updated in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var lastModifiedTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A unique identifier for the kdb scaling group.
*/
public var scalingGroupName: kotlin.String? = null
/**
* The status of scaling group.
* + CREATING – The scaling group creation is in progress.
* + CREATE_FAILED – The scaling group creation has failed.
* + ACTIVE – The scaling group is active.
* + UPDATING – The scaling group is in the process of being updated.
* + UPDATE_FAILED – The update action failed.
* + DELETING – The scaling group is in the process of being deleted.
* + DELETE_FAILED – The system failed to delete the scaling group.
* + DELETED – The scaling group is successfully deleted.
*/
public var status: aws.sdk.kotlin.services.finspace.model.KxScalingGroupStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspace.model.CreateKxScalingGroupResponse) : this() {
this.availabilityZoneId = x.availabilityZoneId
this.createdTimestamp = x.createdTimestamp
this.environmentId = x.environmentId
this.hostType = x.hostType
this.lastModifiedTimestamp = x.lastModifiedTimestamp
this.scalingGroupName = x.scalingGroupName
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspace.model.CreateKxScalingGroupResponse = CreateKxScalingGroupResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy