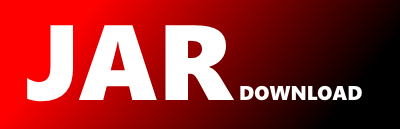
commonMain.aws.sdk.kotlin.services.finspace.model.FederationParameters.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Configuration information when authentication mode is FEDERATED.
*/
public class FederationParameters private constructor(builder: Builder) {
/**
* The redirect or sign-in URL that should be entered into the SAML 2.0 compliant identity provider configuration (IdP).
*/
public val applicationCallBackUrl: kotlin.String? = builder.applicationCallBackUrl
/**
* SAML attribute name and value. The name must always be `Email` and the value should be set to the attribute definition in which user email is set. For example, name would be `Email` and value `http://schemas.xmlsoap.org/ws/2005/05/identity/claims/emailaddress`. Please check your SAML 2.0 compliant identity provider (IdP) documentation for details.
*/
public val attributeMap: Map? = builder.attributeMap
/**
* Name of the identity provider (IdP).
*/
public val federationProviderName: kotlin.String? = builder.federationProviderName
/**
* The Uniform Resource Name (URN). Also referred as Service Provider URN or Audience URI or Service Provider Entity ID.
*/
public val federationUrn: kotlin.String? = builder.federationUrn
/**
* SAML 2.0 Metadata document from identity provider (IdP).
*/
public val samlMetadataDocument: kotlin.String? = builder.samlMetadataDocument
/**
* Provide the metadata URL from your SAML 2.0 compliant identity provider (IdP).
*/
public val samlMetadataUrl: kotlin.String? = builder.samlMetadataUrl
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspace.model.FederationParameters = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("FederationParameters(")
append("applicationCallBackUrl=$applicationCallBackUrl,")
append("attributeMap=$attributeMap,")
append("federationProviderName=$federationProviderName,")
append("federationUrn=$federationUrn,")
append("samlMetadataDocument=$samlMetadataDocument,")
append("samlMetadataUrl=$samlMetadataUrl")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationCallBackUrl?.hashCode() ?: 0
result = 31 * result + (attributeMap?.hashCode() ?: 0)
result = 31 * result + (federationProviderName?.hashCode() ?: 0)
result = 31 * result + (federationUrn?.hashCode() ?: 0)
result = 31 * result + (samlMetadataDocument?.hashCode() ?: 0)
result = 31 * result + (samlMetadataUrl?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as FederationParameters
if (applicationCallBackUrl != other.applicationCallBackUrl) return false
if (attributeMap != other.attributeMap) return false
if (federationProviderName != other.federationProviderName) return false
if (federationUrn != other.federationUrn) return false
if (samlMetadataDocument != other.samlMetadataDocument) return false
if (samlMetadataUrl != other.samlMetadataUrl) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspace.model.FederationParameters = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The redirect or sign-in URL that should be entered into the SAML 2.0 compliant identity provider configuration (IdP).
*/
public var applicationCallBackUrl: kotlin.String? = null
/**
* SAML attribute name and value. The name must always be `Email` and the value should be set to the attribute definition in which user email is set. For example, name would be `Email` and value `http://schemas.xmlsoap.org/ws/2005/05/identity/claims/emailaddress`. Please check your SAML 2.0 compliant identity provider (IdP) documentation for details.
*/
public var attributeMap: Map? = null
/**
* Name of the identity provider (IdP).
*/
public var federationProviderName: kotlin.String? = null
/**
* The Uniform Resource Name (URN). Also referred as Service Provider URN or Audience URI or Service Provider Entity ID.
*/
public var federationUrn: kotlin.String? = null
/**
* SAML 2.0 Metadata document from identity provider (IdP).
*/
public var samlMetadataDocument: kotlin.String? = null
/**
* Provide the metadata URL from your SAML 2.0 compliant identity provider (IdP).
*/
public var samlMetadataUrl: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspace.model.FederationParameters) : this() {
this.applicationCallBackUrl = x.applicationCallBackUrl
this.attributeMap = x.attributeMap
this.federationProviderName = x.federationProviderName
this.federationUrn = x.federationUrn
this.samlMetadataDocument = x.samlMetadataDocument
this.samlMetadataUrl = x.samlMetadataUrl
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspace.model.FederationParameters = FederationParameters(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy