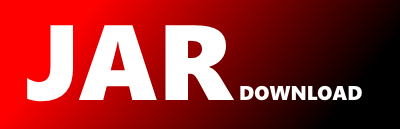
commonMain.aws.sdk.kotlin.services.finspace.model.NetworkAclEntry.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspace-jvm Show documentation
Show all versions of finspace-jvm Show documentation
The AWS SDK for Kotlin client for finspace
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspace.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The network access control list (ACL) is an optional layer of security for your VPC that acts as a firewall for controlling traffic in and out of one or more subnets. The entry is a set of numbered ingress and egress rules that determine whether a packet should be allowed in or out of a subnet associated with the ACL. We process the entries in the ACL according to the rule numbers, in ascending order.
*/
public class NetworkAclEntry private constructor(builder: Builder) {
/**
* The IPv4 network range to allow or deny, in CIDR notation. For example, `172.16.0.0/24`. We modify the specified CIDR block to its canonical form. For example, if you specify `100.68.0.18/18`, we modify it to `100.68.0.0/18`.
*/
public val cidrBlock: kotlin.String = requireNotNull(builder.cidrBlock) { "A non-null value must be provided for cidrBlock" }
/**
* Defines the ICMP protocol that consists of the ICMP type and code.
*/
public val icmpTypeCode: aws.sdk.kotlin.services.finspace.model.IcmpTypeCode? = builder.icmpTypeCode
/**
* The range of ports the rule applies to.
*/
public val portRange: aws.sdk.kotlin.services.finspace.model.PortRange? = builder.portRange
/**
* The protocol number. A value of *-1* means all the protocols.
*/
public val protocol: kotlin.String = requireNotNull(builder.protocol) { "A non-null value must be provided for protocol" }
/**
* Indicates whether to allow or deny the traffic that matches the rule.
*/
public val ruleAction: aws.sdk.kotlin.services.finspace.model.RuleAction = requireNotNull(builder.ruleAction) { "A non-null value must be provided for ruleAction" }
/**
* The rule number for the entry. For example *100*. All the network ACL entries are processed in ascending order by rule number.
*/
public val ruleNumber: kotlin.Int = requireNotNull(builder.ruleNumber) { "A non-null value must be provided for ruleNumber" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspace.model.NetworkAclEntry = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("NetworkAclEntry(")
append("cidrBlock=$cidrBlock,")
append("icmpTypeCode=$icmpTypeCode,")
append("portRange=$portRange,")
append("protocol=$protocol,")
append("ruleAction=$ruleAction,")
append("ruleNumber=$ruleNumber")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = cidrBlock.hashCode()
result = 31 * result + (icmpTypeCode?.hashCode() ?: 0)
result = 31 * result + (portRange?.hashCode() ?: 0)
result = 31 * result + (protocol.hashCode())
result = 31 * result + (ruleAction.hashCode())
result = 31 * result + (ruleNumber)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as NetworkAclEntry
if (cidrBlock != other.cidrBlock) return false
if (icmpTypeCode != other.icmpTypeCode) return false
if (portRange != other.portRange) return false
if (protocol != other.protocol) return false
if (ruleAction != other.ruleAction) return false
if (ruleNumber != other.ruleNumber) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspace.model.NetworkAclEntry = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The IPv4 network range to allow or deny, in CIDR notation. For example, `172.16.0.0/24`. We modify the specified CIDR block to its canonical form. For example, if you specify `100.68.0.18/18`, we modify it to `100.68.0.0/18`.
*/
public var cidrBlock: kotlin.String? = null
/**
* Defines the ICMP protocol that consists of the ICMP type and code.
*/
public var icmpTypeCode: aws.sdk.kotlin.services.finspace.model.IcmpTypeCode? = null
/**
* The range of ports the rule applies to.
*/
public var portRange: aws.sdk.kotlin.services.finspace.model.PortRange? = null
/**
* The protocol number. A value of *-1* means all the protocols.
*/
public var protocol: kotlin.String? = null
/**
* Indicates whether to allow or deny the traffic that matches the rule.
*/
public var ruleAction: aws.sdk.kotlin.services.finspace.model.RuleAction? = null
/**
* The rule number for the entry. For example *100*. All the network ACL entries are processed in ascending order by rule number.
*/
public var ruleNumber: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspace.model.NetworkAclEntry) : this() {
this.cidrBlock = x.cidrBlock
this.icmpTypeCode = x.icmpTypeCode
this.portRange = x.portRange
this.protocol = x.protocol
this.ruleAction = x.ruleAction
this.ruleNumber = x.ruleNumber
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspace.model.NetworkAclEntry = NetworkAclEntry(this)
/**
* construct an [aws.sdk.kotlin.services.finspace.model.IcmpTypeCode] inside the given [block]
*/
public fun icmpTypeCode(block: aws.sdk.kotlin.services.finspace.model.IcmpTypeCode.Builder.() -> kotlin.Unit) {
this.icmpTypeCode = aws.sdk.kotlin.services.finspace.model.IcmpTypeCode.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.finspace.model.PortRange] inside the given [block]
*/
public fun portRange(block: aws.sdk.kotlin.services.finspace.model.PortRange.Builder.() -> kotlin.Unit) {
this.portRange = aws.sdk.kotlin.services.finspace.model.PortRange.invoke(block)
}
internal fun correctErrors(): Builder {
if (cidrBlock == null) cidrBlock = ""
if (protocol == null) protocol = ""
if (ruleAction == null) ruleAction = RuleAction.SdkUnknown("no value provided")
if (ruleNumber == null) ruleNumber = 0
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy