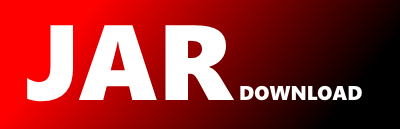
commonMain.aws.sdk.kotlin.services.finspacedata.model.DataViewSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata.model
/**
* Structure for the summary of a Dataview.
*/
public class DataViewSummary private constructor(builder: Builder) {
/**
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val asOfTimestamp: kotlin.Long? = builder.asOfTimestamp
/**
* The flag to indicate Dataview should be updated automatically.
*/
public val autoUpdate: kotlin.Boolean = builder.autoUpdate
/**
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val createTime: kotlin.Long = builder.createTime
/**
* The ARN identifier of the Dataview.
*/
public val dataViewArn: kotlin.String? = builder.dataViewArn
/**
* The unique identifier for the Dataview.
*/
public val dataViewId: kotlin.String? = builder.dataViewId
/**
* Th unique identifier for the Dataview Dataset.
*/
public val datasetId: kotlin.String? = builder.datasetId
/**
* Information about the Dataview destination.
*/
public val destinationTypeProperties: aws.sdk.kotlin.services.finspacedata.model.DataViewDestinationTypeParams? = builder.destinationTypeProperties
/**
* The structure with error messages.
*/
public val errorInfo: aws.sdk.kotlin.services.finspacedata.model.DataViewErrorInfo? = builder.errorInfo
/**
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val lastModifiedTime: kotlin.Long = builder.lastModifiedTime
/**
* Ordered set of column names used to partition data.
*/
public val partitionColumns: List? = builder.partitionColumns
/**
* Columns to be used for sorting the data.
*/
public val sortColumns: List? = builder.sortColumns
/**
* The status of a Dataview creation.
* + `RUNNING` – Dataview creation is running.
* + `STARTING` – Dataview creation is starting.
* + `FAILED` – Dataview creation has failed.
* + `CANCELLED` – Dataview creation has been cancelled.
* + `TIMEOUT` – Dataview creation has timed out.
* + `SUCCESS` – Dataview creation has succeeded.
* + `PENDING` – Dataview creation is pending.
* + `FAILED_CLEANUP_FAILED` – Dataview creation failed and resource cleanup failed.
*/
public val status: aws.sdk.kotlin.services.finspacedata.model.DataViewStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspacedata.model.DataViewSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DataViewSummary(")
append("asOfTimestamp=$asOfTimestamp,")
append("autoUpdate=$autoUpdate,")
append("createTime=$createTime,")
append("dataViewArn=$dataViewArn,")
append("dataViewId=$dataViewId,")
append("datasetId=$datasetId,")
append("destinationTypeProperties=$destinationTypeProperties,")
append("errorInfo=$errorInfo,")
append("lastModifiedTime=$lastModifiedTime,")
append("partitionColumns=$partitionColumns,")
append("sortColumns=$sortColumns,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = asOfTimestamp?.hashCode() ?: 0
result = 31 * result + (autoUpdate.hashCode())
result = 31 * result + (createTime.hashCode())
result = 31 * result + (dataViewArn?.hashCode() ?: 0)
result = 31 * result + (dataViewId?.hashCode() ?: 0)
result = 31 * result + (datasetId?.hashCode() ?: 0)
result = 31 * result + (destinationTypeProperties?.hashCode() ?: 0)
result = 31 * result + (errorInfo?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime.hashCode())
result = 31 * result + (partitionColumns?.hashCode() ?: 0)
result = 31 * result + (sortColumns?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DataViewSummary
if (asOfTimestamp != other.asOfTimestamp) return false
if (autoUpdate != other.autoUpdate) return false
if (createTime != other.createTime) return false
if (dataViewArn != other.dataViewArn) return false
if (dataViewId != other.dataViewId) return false
if (datasetId != other.datasetId) return false
if (destinationTypeProperties != other.destinationTypeProperties) return false
if (errorInfo != other.errorInfo) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (partitionColumns != other.partitionColumns) return false
if (sortColumns != other.sortColumns) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspacedata.model.DataViewSummary = Builder(this).apply(block).build()
public class Builder {
/**
* Time range to use for the Dataview. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var asOfTimestamp: kotlin.Long? = null
/**
* The flag to indicate Dataview should be updated automatically.
*/
public var autoUpdate: kotlin.Boolean = false
/**
* The timestamp at which the Dataview was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var createTime: kotlin.Long = 0L
/**
* The ARN identifier of the Dataview.
*/
public var dataViewArn: kotlin.String? = null
/**
* The unique identifier for the Dataview.
*/
public var dataViewId: kotlin.String? = null
/**
* Th unique identifier for the Dataview Dataset.
*/
public var datasetId: kotlin.String? = null
/**
* Information about the Dataview destination.
*/
public var destinationTypeProperties: aws.sdk.kotlin.services.finspacedata.model.DataViewDestinationTypeParams? = null
/**
* The structure with error messages.
*/
public var errorInfo: aws.sdk.kotlin.services.finspacedata.model.DataViewErrorInfo? = null
/**
* The last time that a Dataview was modified. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var lastModifiedTime: kotlin.Long = 0L
/**
* Ordered set of column names used to partition data.
*/
public var partitionColumns: List? = null
/**
* Columns to be used for sorting the data.
*/
public var sortColumns: List? = null
/**
* The status of a Dataview creation.
* + `RUNNING` – Dataview creation is running.
* + `STARTING` – Dataview creation is starting.
* + `FAILED` – Dataview creation has failed.
* + `CANCELLED` – Dataview creation has been cancelled.
* + `TIMEOUT` – Dataview creation has timed out.
* + `SUCCESS` – Dataview creation has succeeded.
* + `PENDING` – Dataview creation is pending.
* + `FAILED_CLEANUP_FAILED` – Dataview creation failed and resource cleanup failed.
*/
public var status: aws.sdk.kotlin.services.finspacedata.model.DataViewStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspacedata.model.DataViewSummary) : this() {
this.asOfTimestamp = x.asOfTimestamp
this.autoUpdate = x.autoUpdate
this.createTime = x.createTime
this.dataViewArn = x.dataViewArn
this.dataViewId = x.dataViewId
this.datasetId = x.datasetId
this.destinationTypeProperties = x.destinationTypeProperties
this.errorInfo = x.errorInfo
this.lastModifiedTime = x.lastModifiedTime
this.partitionColumns = x.partitionColumns
this.sortColumns = x.sortColumns
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspacedata.model.DataViewSummary = DataViewSummary(this)
/**
* construct an [aws.sdk.kotlin.services.finspacedata.model.DataViewDestinationTypeParams] inside the given [block]
*/
public fun destinationTypeProperties(block: aws.sdk.kotlin.services.finspacedata.model.DataViewDestinationTypeParams.Builder.() -> kotlin.Unit) {
this.destinationTypeProperties = aws.sdk.kotlin.services.finspacedata.model.DataViewDestinationTypeParams.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.finspacedata.model.DataViewErrorInfo] inside the given [block]
*/
public fun errorInfo(block: aws.sdk.kotlin.services.finspacedata.model.DataViewErrorInfo.Builder.() -> kotlin.Unit) {
this.errorInfo = aws.sdk.kotlin.services.finspacedata.model.DataViewErrorInfo.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy