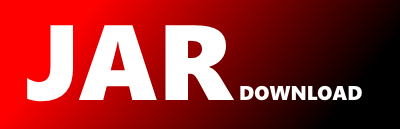
commonMain.aws.sdk.kotlin.services.finspacedata.model.GetChangesetResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata.model
/**
* The response from a describe changeset operation
*/
public class GetChangesetResponse private constructor(builder: Builder) {
/**
* Beginning time from which the Changeset is active. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val activeFromTimestamp: kotlin.Long? = builder.activeFromTimestamp
/**
* Time until which the Changeset is active. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val activeUntilTimestamp: kotlin.Long? = builder.activeUntilTimestamp
/**
* Type that indicates how a Changeset is applied to a Dataset.
* + `REPLACE` – Changeset is considered as a replacement to all prior loaded Changesets.
* + `APPEND` – Changeset is considered as an addition to the end of all prior loaded Changesets.
* + `MODIFY` – Changeset is considered as a replacement to a specific prior ingested Changeset.
*/
public val changeType: aws.sdk.kotlin.services.finspacedata.model.ChangeType? = builder.changeType
/**
* The ARN identifier of the Changeset.
*/
public val changesetArn: kotlin.String? = builder.changesetArn
/**
* The unique identifier for a Changeset.
*/
public val changesetId: kotlin.String? = builder.changesetId
/**
* The timestamp at which the Changeset was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val createTime: kotlin.Long = builder.createTime
/**
* The unique identifier for the FinSpace Dataset where the Changeset is created.
*/
public val datasetId: kotlin.String? = builder.datasetId
/**
* The structure with error messages.
*/
public val errorInfo: aws.sdk.kotlin.services.finspacedata.model.ChangesetErrorInfo? = builder.errorInfo
/**
* Structure of the source file(s).
*/
public val formatParams: Map? = builder.formatParams
/**
* Options that define the location of the data being ingested.
*/
public val sourceParams: Map? = builder.sourceParams
/**
* The status of Changeset creation operation.
*/
public val status: aws.sdk.kotlin.services.finspacedata.model.IngestionStatus? = builder.status
/**
* The unique identifier of the updated Changeset.
*/
public val updatedByChangesetId: kotlin.String? = builder.updatedByChangesetId
/**
* The unique identifier of the Changeset that is being updated.
*/
public val updatesChangesetId: kotlin.String? = builder.updatesChangesetId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspacedata.model.GetChangesetResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetChangesetResponse(")
append("activeFromTimestamp=$activeFromTimestamp,")
append("activeUntilTimestamp=$activeUntilTimestamp,")
append("changeType=$changeType,")
append("changesetArn=$changesetArn,")
append("changesetId=$changesetId,")
append("createTime=$createTime,")
append("datasetId=$datasetId,")
append("errorInfo=$errorInfo,")
append("formatParams=$formatParams,")
append("sourceParams=$sourceParams,")
append("status=$status,")
append("updatedByChangesetId=$updatedByChangesetId,")
append("updatesChangesetId=$updatesChangesetId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = activeFromTimestamp?.hashCode() ?: 0
result = 31 * result + (activeUntilTimestamp?.hashCode() ?: 0)
result = 31 * result + (changeType?.hashCode() ?: 0)
result = 31 * result + (changesetArn?.hashCode() ?: 0)
result = 31 * result + (changesetId?.hashCode() ?: 0)
result = 31 * result + (createTime.hashCode())
result = 31 * result + (datasetId?.hashCode() ?: 0)
result = 31 * result + (errorInfo?.hashCode() ?: 0)
result = 31 * result + (formatParams?.hashCode() ?: 0)
result = 31 * result + (sourceParams?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (updatedByChangesetId?.hashCode() ?: 0)
result = 31 * result + (updatesChangesetId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetChangesetResponse
if (activeFromTimestamp != other.activeFromTimestamp) return false
if (activeUntilTimestamp != other.activeUntilTimestamp) return false
if (changeType != other.changeType) return false
if (changesetArn != other.changesetArn) return false
if (changesetId != other.changesetId) return false
if (createTime != other.createTime) return false
if (datasetId != other.datasetId) return false
if (errorInfo != other.errorInfo) return false
if (formatParams != other.formatParams) return false
if (sourceParams != other.sourceParams) return false
if (status != other.status) return false
if (updatedByChangesetId != other.updatedByChangesetId) return false
if (updatesChangesetId != other.updatesChangesetId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspacedata.model.GetChangesetResponse = Builder(this).apply(block).build()
public class Builder {
/**
* Beginning time from which the Changeset is active. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var activeFromTimestamp: kotlin.Long? = null
/**
* Time until which the Changeset is active. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var activeUntilTimestamp: kotlin.Long? = null
/**
* Type that indicates how a Changeset is applied to a Dataset.
* + `REPLACE` – Changeset is considered as a replacement to all prior loaded Changesets.
* + `APPEND` – Changeset is considered as an addition to the end of all prior loaded Changesets.
* + `MODIFY` – Changeset is considered as a replacement to a specific prior ingested Changeset.
*/
public var changeType: aws.sdk.kotlin.services.finspacedata.model.ChangeType? = null
/**
* The ARN identifier of the Changeset.
*/
public var changesetArn: kotlin.String? = null
/**
* The unique identifier for a Changeset.
*/
public var changesetId: kotlin.String? = null
/**
* The timestamp at which the Changeset was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var createTime: kotlin.Long = 0L
/**
* The unique identifier for the FinSpace Dataset where the Changeset is created.
*/
public var datasetId: kotlin.String? = null
/**
* The structure with error messages.
*/
public var errorInfo: aws.sdk.kotlin.services.finspacedata.model.ChangesetErrorInfo? = null
/**
* Structure of the source file(s).
*/
public var formatParams: Map? = null
/**
* Options that define the location of the data being ingested.
*/
public var sourceParams: Map? = null
/**
* The status of Changeset creation operation.
*/
public var status: aws.sdk.kotlin.services.finspacedata.model.IngestionStatus? = null
/**
* The unique identifier of the updated Changeset.
*/
public var updatedByChangesetId: kotlin.String? = null
/**
* The unique identifier of the Changeset that is being updated.
*/
public var updatesChangesetId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspacedata.model.GetChangesetResponse) : this() {
this.activeFromTimestamp = x.activeFromTimestamp
this.activeUntilTimestamp = x.activeUntilTimestamp
this.changeType = x.changeType
this.changesetArn = x.changesetArn
this.changesetId = x.changesetId
this.createTime = x.createTime
this.datasetId = x.datasetId
this.errorInfo = x.errorInfo
this.formatParams = x.formatParams
this.sourceParams = x.sourceParams
this.status = x.status
this.updatedByChangesetId = x.updatedByChangesetId
this.updatesChangesetId = x.updatesChangesetId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspacedata.model.GetChangesetResponse = GetChangesetResponse(this)
/**
* construct an [aws.sdk.kotlin.services.finspacedata.model.ChangesetErrorInfo] inside the given [block]
*/
public fun errorInfo(block: aws.sdk.kotlin.services.finspacedata.model.ChangesetErrorInfo.Builder.() -> kotlin.Unit) {
this.errorInfo = aws.sdk.kotlin.services.finspacedata.model.ChangesetErrorInfo.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy