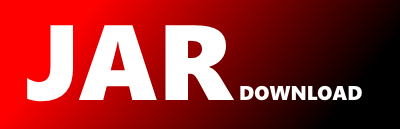
commonMain.aws.sdk.kotlin.services.finspacedata.DefaultFinspaceDataClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.interceptors.BusinessMetricsInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.finspacedata.auth.FinspaceDataAuthSchemeProviderAdapter
import aws.sdk.kotlin.services.finspacedata.auth.FinspaceDataIdentityProviderConfigAdapter
import aws.sdk.kotlin.services.finspacedata.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.finspacedata.model.*
import aws.sdk.kotlin.services.finspacedata.serde.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.awsprotocol.AwsAttributes
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.collections.attributesOf
import aws.smithy.kotlin.runtime.collections.putIfAbsent
import aws.smithy.kotlin.runtime.collections.putIfAbsentNotNull
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
internal class DefaultFinspaceDataClient(override val config: FinspaceDataClient.Config) : FinspaceDataClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = FinspaceDataIdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(AuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "finspace-api")
}
toMap()
}
private val authSchemeAdapter = FinspaceDataAuthSchemeProviderAdapter(config)
private val telemetryScope = "aws.sdk.kotlin.services.finspacedata"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion), config.applicationId)
/**
* Adds a user to a permission group to grant permissions for actions a user can perform in FinSpace.
*/
@Deprecated("This method will be discontinued.")
override suspend fun associateUserToPermissionGroup(input: AssociateUserToPermissionGroupRequest): AssociateUserToPermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = AssociateUserToPermissionGroupOperationSerializer()
deserializeWith = AssociateUserToPermissionGroupOperationDeserializer()
operationName = "AssociateUserToPermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new Changeset in a FinSpace Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createChangeset(input: CreateChangesetRequest): CreateChangesetResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateChangesetOperationSerializer()
deserializeWith = CreateChangesetOperationDeserializer()
operationName = "CreateChangeset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a Dataview for a Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createDataView(input: CreateDataViewRequest): CreateDataViewResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateDataViewOperationSerializer()
deserializeWith = CreateDataViewOperationDeserializer()
operationName = "CreateDataView"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new FinSpace Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createDataset(input: CreateDatasetRequest): CreateDatasetResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateDatasetOperationSerializer()
deserializeWith = CreateDatasetOperationDeserializer()
operationName = "CreateDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a group of permissions for various actions that a user can perform in FinSpace.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createPermissionGroup(input: CreatePermissionGroupRequest): CreatePermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = CreatePermissionGroupOperationSerializer()
deserializeWith = CreatePermissionGroupOperationDeserializer()
operationName = "CreatePermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Creates a new user in FinSpace.
*/
@Deprecated("This method will be discontinued.")
override suspend fun createUser(input: CreateUserRequest): CreateUserResponse {
val op = SdkHttpOperation.build {
serializeWith = CreateUserOperationSerializer()
deserializeWith = CreateUserOperationDeserializer()
operationName = "CreateUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a FinSpace Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun deleteDataset(input: DeleteDatasetRequest): DeleteDatasetResponse {
val op = SdkHttpOperation.build {
serializeWith = DeleteDatasetOperationSerializer()
deserializeWith = DeleteDatasetOperationDeserializer()
operationName = "DeleteDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Deletes a permission group. This action is irreversible.
*/
@Deprecated("This method will be discontinued.")
override suspend fun deletePermissionGroup(input: DeletePermissionGroupRequest): DeletePermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = DeletePermissionGroupOperationSerializer()
deserializeWith = DeletePermissionGroupOperationDeserializer()
operationName = "DeletePermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Denies access to the FinSpace web application and API for the specified user.
*/
@Deprecated("This method will be discontinued.")
override suspend fun disableUser(input: DisableUserRequest): DisableUserResponse {
val op = SdkHttpOperation.build {
serializeWith = DisableUserOperationSerializer()
deserializeWith = DisableUserOperationDeserializer()
operationName = "DisableUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes a user from a permission group.
*/
@Deprecated("This method will be discontinued.")
override suspend fun disassociateUserFromPermissionGroup(input: DisassociateUserFromPermissionGroupRequest): DisassociateUserFromPermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = DisassociateUserFromPermissionGroupOperationSerializer()
deserializeWith = DisassociateUserFromPermissionGroupOperationDeserializer()
operationName = "DisassociateUserFromPermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Allows the specified user to access the FinSpace web application and API.
*/
@Deprecated("This method will be discontinued.")
override suspend fun enableUser(input: EnableUserRequest): EnableUserResponse {
val op = SdkHttpOperation.build {
serializeWith = EnableUserOperationSerializer()
deserializeWith = EnableUserOperationDeserializer()
operationName = "EnableUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Get information about a Changeset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getChangeset(input: GetChangesetRequest): GetChangesetResponse {
val op = SdkHttpOperation.build {
serializeWith = GetChangesetOperationSerializer()
deserializeWith = GetChangesetOperationDeserializer()
operationName = "GetChangeset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Gets information about a Dataview.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getDataView(input: GetDataViewRequest): GetDataViewResponse {
val op = SdkHttpOperation.build {
serializeWith = GetDataViewOperationSerializer()
deserializeWith = GetDataViewOperationDeserializer()
operationName = "GetDataView"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns information about a Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getDataset(input: GetDatasetRequest): GetDatasetResponse {
val op = SdkHttpOperation.build {
serializeWith = GetDatasetOperationSerializer()
deserializeWith = GetDatasetOperationDeserializer()
operationName = "GetDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the credentials to access the external Dataview from an S3 location. To call this API:
* + You must retrieve the programmatic credentials.
* + You must be a member of a FinSpace user group, where the dataset that you want to access has `Read Dataset Data` permissions.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getExternalDataViewAccessDetails(input: GetExternalDataViewAccessDetailsRequest): GetExternalDataViewAccessDetailsResponse {
val op = SdkHttpOperation.build {
serializeWith = GetExternalDataViewAccessDetailsOperationSerializer()
deserializeWith = GetExternalDataViewAccessDetailsOperationDeserializer()
operationName = "GetExternalDataViewAccessDetails"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves the details of a specific permission group.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getPermissionGroup(input: GetPermissionGroupRequest): GetPermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = GetPermissionGroupOperationSerializer()
deserializeWith = GetPermissionGroupOperationDeserializer()
operationName = "GetPermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Request programmatic credentials to use with FinSpace SDK. For more information, see [Step 2. Access credentials programmatically using IAM access key id and secret access key](https://docs.aws.amazon.com/finspace/latest/data-api/fs-using-the-finspace-api.html#accessing-credentials).
*/
@Deprecated("This method will be discontinued.")
override suspend fun getProgrammaticAccessCredentials(input: GetProgrammaticAccessCredentialsRequest): GetProgrammaticAccessCredentialsResponse {
val op = SdkHttpOperation.build {
serializeWith = GetProgrammaticAccessCredentialsOperationSerializer()
deserializeWith = GetProgrammaticAccessCredentialsOperationDeserializer()
operationName = "GetProgrammaticAccessCredentials"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Retrieves details for a specific user.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getUser(input: GetUserRequest): GetUserResponse {
val op = SdkHttpOperation.build {
serializeWith = GetUserOperationSerializer()
deserializeWith = GetUserOperationDeserializer()
operationName = "GetUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* A temporary Amazon S3 location, where you can copy your files from a source location to stage or use as a scratch space in FinSpace notebook.
*/
@Deprecated("This method will be discontinued.")
override suspend fun getWorkingLocation(input: GetWorkingLocationRequest): GetWorkingLocationResponse {
val op = SdkHttpOperation.build {
serializeWith = GetWorkingLocationOperationSerializer()
deserializeWith = GetWorkingLocationOperationDeserializer()
operationName = "GetWorkingLocation"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists the FinSpace Changesets for a Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listChangesets(input: ListChangesetsRequest): ListChangesetsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListChangesetsOperationSerializer()
deserializeWith = ListChangesetsOperationDeserializer()
operationName = "ListChangesets"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all available Dataviews for a Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listDataViews(input: ListDataViewsRequest): ListDataViewsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListDataViewsOperationSerializer()
deserializeWith = ListDataViewsOperationDeserializer()
operationName = "ListDataViews"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all of the active Datasets that a user has access to.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listDatasets(input: ListDatasetsRequest): ListDatasetsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListDatasetsOperationSerializer()
deserializeWith = ListDatasetsOperationDeserializer()
operationName = "ListDatasets"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all available permission groups in FinSpace.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listPermissionGroups(input: ListPermissionGroupsRequest): ListPermissionGroupsResponse {
val op = SdkHttpOperation.build {
serializeWith = ListPermissionGroupsOperationSerializer()
deserializeWith = ListPermissionGroupsOperationDeserializer()
operationName = "ListPermissionGroups"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all the permission groups that are associated with a specific user.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listPermissionGroupsByUser(input: ListPermissionGroupsByUserRequest): ListPermissionGroupsByUserResponse {
val op = SdkHttpOperation.build {
serializeWith = ListPermissionGroupsByUserOperationSerializer()
deserializeWith = ListPermissionGroupsByUserOperationDeserializer()
operationName = "ListPermissionGroupsByUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists all available users in FinSpace.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listUsers(input: ListUsersRequest): ListUsersResponse {
val op = SdkHttpOperation.build {
serializeWith = ListUsersOperationSerializer()
deserializeWith = ListUsersOperationDeserializer()
operationName = "ListUsers"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Lists details of all the users in a specific permission group.
*/
@Deprecated("This method will be discontinued.")
override suspend fun listUsersByPermissionGroup(input: ListUsersByPermissionGroupRequest): ListUsersByPermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = ListUsersByPermissionGroupOperationSerializer()
deserializeWith = ListUsersByPermissionGroupOperationDeserializer()
operationName = "ListUsersByPermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Resets the password for a specified user ID and generates a temporary one. Only a superuser can reset password for other users. Resetting the password immediately invalidates the previous password associated with the user.
*/
@Deprecated("This method will be discontinued.")
override suspend fun resetUserPassword(input: ResetUserPasswordRequest): ResetUserPasswordResponse {
val op = SdkHttpOperation.build {
serializeWith = ResetUserPasswordOperationSerializer()
deserializeWith = ResetUserPasswordOperationDeserializer()
operationName = "ResetUserPassword"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates a FinSpace Changeset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun updateChangeset(input: UpdateChangesetRequest): UpdateChangesetResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateChangesetOperationSerializer()
deserializeWith = UpdateChangesetOperationDeserializer()
operationName = "UpdateChangeset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Updates a FinSpace Dataset.
*/
@Deprecated("This method will be discontinued.")
override suspend fun updateDataset(input: UpdateDatasetRequest): UpdateDatasetResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateDatasetOperationSerializer()
deserializeWith = UpdateDatasetOperationDeserializer()
operationName = "UpdateDataset"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the details of a permission group. You cannot modify a `permissionGroupID`.
*/
@Deprecated("This method will be discontinued.")
override suspend fun updatePermissionGroup(input: UpdatePermissionGroupRequest): UpdatePermissionGroupResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdatePermissionGroupOperationSerializer()
deserializeWith = UpdatePermissionGroupOperationDeserializer()
operationName = "UpdatePermissionGroup"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Modifies the details of the specified user. You cannot update the `userId` for a user.
*/
@Deprecated("This method will be discontinued.")
override suspend fun updateUser(input: UpdateUserRequest): UpdateUserResponse {
val op = SdkHttpOperation.build {
serializeWith = UpdateUserOperationSerializer()
deserializeWith = UpdateUserOperationDeserializer()
operationName = "UpdateUser"
serviceName = ServiceId
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(authSchemeAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
execution.retryPolicy = config.retryPolicy
}
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.interceptors.add(AwsSpanInterceptor)
op.interceptors.add(BusinessMetricsInterceptor())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsentNotNull(SdkClientOption.IdempotencyTokenProvider, config.idempotencyTokenProvider)
ctx.putIfAbsentNotNull(AwsAttributes.Region, config.region)
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "finspace-api")
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy