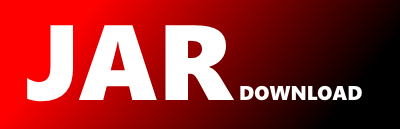
commonMain.aws.sdk.kotlin.services.finspacedata.model.CreateChangesetRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* The request for a CreateChangeset operation.
*/
public class CreateChangesetRequest private constructor(builder: Builder) {
/**
* The option to indicate how a Changeset will be applied to a Dataset.
* + `REPLACE` – Changeset will be considered as a replacement to all prior loaded Changesets.
* + `APPEND` – Changeset will be considered as an addition to the end of all prior loaded Changesets.
* + `MODIFY` – Changeset is considered as a replacement to a specific prior ingested Changeset.
*/
public val changeType: aws.sdk.kotlin.services.finspacedata.model.ChangeType? = builder.changeType
/**
* A token that ensures idempotency. This token expires in 10 minutes.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The unique identifier for the FinSpace Dataset where the Changeset will be created.
*/
public val datasetId: kotlin.String? = builder.datasetId
/**
* Options that define the structure of the source file(s) including the format type (`formatType`), header row (`withHeader`), data separation character (`separator`) and the type of compression (`compression`).
*
* `formatType` is a required attribute and can have the following values:
* + `PARQUET` – Parquet source file format.
* + `CSV` – CSV source file format.
* + `JSON` – JSON source file format.
* + `XML` – XML source file format.
*
* Here is an example of how you could specify the `formatParams`:
*
* ` "formatParams": { "formatType": "CSV", "withHeader": "true", "separator": ",", "compression":"None" } `
*
* Note that if you only provide `formatType` as `CSV`, the rest of the attributes will automatically default to CSV values as following:
*
* ` { "withHeader": "true", "separator": "," } `
*
* For more information about supported file formats, see [Supported Data Types and File Formats](https://docs.aws.amazon.com/finspace/latest/userguide/supported-data-types.html) in the FinSpace User Guide.
*/
public val formatParams: Map? = builder.formatParams
/**
* Options that define the location of the data being ingested (`s3SourcePath`) and the source of the changeset (`sourceType`).
*
* Both `s3SourcePath` and `sourceType` are required attributes.
*
* Here is an example of how you could specify the `sourceParams`:
*
* ` "sourceParams": { "s3SourcePath": "s3://finspace-landing-us-east-2-bk7gcfvitndqa6ebnvys4d/scratch/wr5hh8pwkpqqkxa4sxrmcw/ingestion/equity.csv", "sourceType": "S3" } `
*
* The S3 path that you specify must allow the FinSpace role access. To do that, you first need to configure the IAM policy on S3 bucket. For more information, see [Loading data from an Amazon S3 Bucket using the FinSpace API](https://docs.aws.amazon.com/finspace/latest/data-api/fs-using-the-finspace-api.html#access-s3-buckets) section.
*/
public val sourceParams: Map? = builder.sourceParams
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspacedata.model.CreateChangesetRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateChangesetRequest(")
append("changeType=$changeType,")
append("clientToken=$clientToken,")
append("datasetId=$datasetId,")
append("formatParams=$formatParams,")
append("sourceParams=$sourceParams")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = changeType?.hashCode() ?: 0
result = 31 * result + (clientToken?.hashCode() ?: 0)
result = 31 * result + (datasetId?.hashCode() ?: 0)
result = 31 * result + (formatParams?.hashCode() ?: 0)
result = 31 * result + (sourceParams?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateChangesetRequest
if (changeType != other.changeType) return false
if (clientToken != other.clientToken) return false
if (datasetId != other.datasetId) return false
if (formatParams != other.formatParams) return false
if (sourceParams != other.sourceParams) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspacedata.model.CreateChangesetRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The option to indicate how a Changeset will be applied to a Dataset.
* + `REPLACE` – Changeset will be considered as a replacement to all prior loaded Changesets.
* + `APPEND` – Changeset will be considered as an addition to the end of all prior loaded Changesets.
* + `MODIFY` – Changeset is considered as a replacement to a specific prior ingested Changeset.
*/
public var changeType: aws.sdk.kotlin.services.finspacedata.model.ChangeType? = null
/**
* A token that ensures idempotency. This token expires in 10 minutes.
*/
public var clientToken: kotlin.String? = null
/**
* The unique identifier for the FinSpace Dataset where the Changeset will be created.
*/
public var datasetId: kotlin.String? = null
/**
* Options that define the structure of the source file(s) including the format type (`formatType`), header row (`withHeader`), data separation character (`separator`) and the type of compression (`compression`).
*
* `formatType` is a required attribute and can have the following values:
* + `PARQUET` – Parquet source file format.
* + `CSV` – CSV source file format.
* + `JSON` – JSON source file format.
* + `XML` – XML source file format.
*
* Here is an example of how you could specify the `formatParams`:
*
* ` "formatParams": { "formatType": "CSV", "withHeader": "true", "separator": ",", "compression":"None" } `
*
* Note that if you only provide `formatType` as `CSV`, the rest of the attributes will automatically default to CSV values as following:
*
* ` { "withHeader": "true", "separator": "," } `
*
* For more information about supported file formats, see [Supported Data Types and File Formats](https://docs.aws.amazon.com/finspace/latest/userguide/supported-data-types.html) in the FinSpace User Guide.
*/
public var formatParams: Map? = null
/**
* Options that define the location of the data being ingested (`s3SourcePath`) and the source of the changeset (`sourceType`).
*
* Both `s3SourcePath` and `sourceType` are required attributes.
*
* Here is an example of how you could specify the `sourceParams`:
*
* ` "sourceParams": { "s3SourcePath": "s3://finspace-landing-us-east-2-bk7gcfvitndqa6ebnvys4d/scratch/wr5hh8pwkpqqkxa4sxrmcw/ingestion/equity.csv", "sourceType": "S3" } `
*
* The S3 path that you specify must allow the FinSpace role access. To do that, you first need to configure the IAM policy on S3 bucket. For more information, see [Loading data from an Amazon S3 Bucket using the FinSpace API](https://docs.aws.amazon.com/finspace/latest/data-api/fs-using-the-finspace-api.html#access-s3-buckets) section.
*/
public var sourceParams: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspacedata.model.CreateChangesetRequest) : this() {
this.changeType = x.changeType
this.clientToken = x.clientToken
this.datasetId = x.datasetId
this.formatParams = x.formatParams
this.sourceParams = x.sourceParams
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspacedata.model.CreateChangesetRequest = CreateChangesetRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy