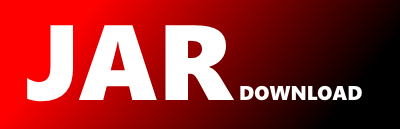
commonMain.aws.sdk.kotlin.services.finspacedata.model.GetDatasetResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Response for the GetDataset operation
*/
public class GetDatasetResponse private constructor(builder: Builder) {
/**
* The unique resource identifier for a Dataset.
*/
public val alias: kotlin.String? = builder.alias
/**
* The timestamp at which the Dataset was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val createTime: kotlin.Long = builder.createTime
/**
* The ARN identifier of the Dataset.
*/
public val datasetArn: kotlin.String? = builder.datasetArn
/**
* A description of the Dataset.
*/
public val datasetDescription: kotlin.String? = builder.datasetDescription
/**
* The unique identifier for a Dataset.
*/
public val datasetId: kotlin.String? = builder.datasetId
/**
* Display title for a Dataset.
*/
public val datasetTitle: kotlin.String? = builder.datasetTitle
/**
* The format in which Dataset data is structured.
* + `TABULAR` – Data is structured in a tabular format.
* + `NON_TABULAR` – Data is structured in a non-tabular format.
*/
public val kind: aws.sdk.kotlin.services.finspacedata.model.DatasetKind? = builder.kind
/**
* The last time that the Dataset was modified. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public val lastModifiedTime: kotlin.Long = builder.lastModifiedTime
/**
* Definition for a schema on a tabular Dataset.
*/
public val schemaDefinition: aws.sdk.kotlin.services.finspacedata.model.SchemaUnion? = builder.schemaDefinition
/**
* Status of the Dataset creation.
* + `PENDING` – Dataset is pending creation.
* + `FAILED` – Dataset creation has failed.
* + `SUCCESS` – Dataset creation has succeeded.
* + `RUNNING` – Dataset creation is running.
*/
public val status: aws.sdk.kotlin.services.finspacedata.model.DatasetStatus? = builder.status
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspacedata.model.GetDatasetResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetDatasetResponse(")
append("alias=$alias,")
append("createTime=$createTime,")
append("datasetArn=$datasetArn,")
append("datasetDescription=$datasetDescription,")
append("datasetId=$datasetId,")
append("datasetTitle=$datasetTitle,")
append("kind=$kind,")
append("lastModifiedTime=$lastModifiedTime,")
append("schemaDefinition=$schemaDefinition,")
append("status=$status")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = alias?.hashCode() ?: 0
result = 31 * result + (createTime.hashCode())
result = 31 * result + (datasetArn?.hashCode() ?: 0)
result = 31 * result + (datasetDescription?.hashCode() ?: 0)
result = 31 * result + (datasetId?.hashCode() ?: 0)
result = 31 * result + (datasetTitle?.hashCode() ?: 0)
result = 31 * result + (kind?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime.hashCode())
result = 31 * result + (schemaDefinition?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetDatasetResponse
if (alias != other.alias) return false
if (createTime != other.createTime) return false
if (datasetArn != other.datasetArn) return false
if (datasetDescription != other.datasetDescription) return false
if (datasetId != other.datasetId) return false
if (datasetTitle != other.datasetTitle) return false
if (kind != other.kind) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (schemaDefinition != other.schemaDefinition) return false
if (status != other.status) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspacedata.model.GetDatasetResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The unique resource identifier for a Dataset.
*/
public var alias: kotlin.String? = null
/**
* The timestamp at which the Dataset was created in FinSpace. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var createTime: kotlin.Long = 0L
/**
* The ARN identifier of the Dataset.
*/
public var datasetArn: kotlin.String? = null
/**
* A description of the Dataset.
*/
public var datasetDescription: kotlin.String? = null
/**
* The unique identifier for a Dataset.
*/
public var datasetId: kotlin.String? = null
/**
* Display title for a Dataset.
*/
public var datasetTitle: kotlin.String? = null
/**
* The format in which Dataset data is structured.
* + `TABULAR` – Data is structured in a tabular format.
* + `NON_TABULAR` – Data is structured in a non-tabular format.
*/
public var kind: aws.sdk.kotlin.services.finspacedata.model.DatasetKind? = null
/**
* The last time that the Dataset was modified. The value is determined as epoch time in milliseconds. For example, the value for Monday, November 1, 2021 12:00:00 PM UTC is specified as 1635768000000.
*/
public var lastModifiedTime: kotlin.Long = 0L
/**
* Definition for a schema on a tabular Dataset.
*/
public var schemaDefinition: aws.sdk.kotlin.services.finspacedata.model.SchemaUnion? = null
/**
* Status of the Dataset creation.
* + `PENDING` – Dataset is pending creation.
* + `FAILED` – Dataset creation has failed.
* + `SUCCESS` – Dataset creation has succeeded.
* + `RUNNING` – Dataset creation is running.
*/
public var status: aws.sdk.kotlin.services.finspacedata.model.DatasetStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspacedata.model.GetDatasetResponse) : this() {
this.alias = x.alias
this.createTime = x.createTime
this.datasetArn = x.datasetArn
this.datasetDescription = x.datasetDescription
this.datasetId = x.datasetId
this.datasetTitle = x.datasetTitle
this.kind = x.kind
this.lastModifiedTime = x.lastModifiedTime
this.schemaDefinition = x.schemaDefinition
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspacedata.model.GetDatasetResponse = GetDatasetResponse(this)
/**
* construct an [aws.sdk.kotlin.services.finspacedata.model.SchemaUnion] inside the given [block]
*/
public fun schemaDefinition(block: aws.sdk.kotlin.services.finspacedata.model.SchemaUnion.Builder.() -> kotlin.Unit) {
this.schemaDefinition = aws.sdk.kotlin.services.finspacedata.model.SchemaUnion.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy