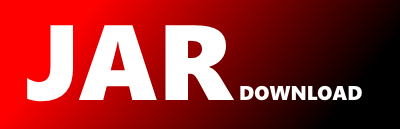
commonMain.aws.sdk.kotlin.services.finspacedata.model.GetUserResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of finspacedata-jvm Show documentation
Show all versions of finspacedata-jvm Show documentation
The AWS SDK for Kotlin client for finspace data
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.finspacedata.model
import aws.smithy.kotlin.runtime.SdkDsl
public class GetUserResponse private constructor(builder: Builder) {
/**
* Indicates whether the user can use the `GetProgrammaticAccessCredentials` API to obtain credentials that can then be used to access other FinSpace Data API operations.
* + `ENABLED` – The user has permissions to use the APIs.
* + `DISABLED` – The user does not have permissions to use any APIs.
*/
public val apiAccess: aws.sdk.kotlin.services.finspacedata.model.ApiAccess? = builder.apiAccess
/**
* The ARN identifier of an AWS user or role that is allowed to call the `GetProgrammaticAccessCredentials` API to obtain a credentials token for a specific FinSpace user. This must be an IAM role within your FinSpace account.
*/
public val apiAccessPrincipalArn: kotlin.String? = builder.apiAccessPrincipalArn
/**
* The timestamp at which the user was created in FinSpace. The value is determined as epoch time in milliseconds.
*/
public val createTime: kotlin.Long = builder.createTime
/**
* The email address that is associated with the user.
*/
public val emailAddress: kotlin.String? = builder.emailAddress
/**
* The first name of the user.
*/
public val firstName: kotlin.String? = builder.firstName
/**
* Describes the last time the user was deactivated. The value is determined as epoch time in milliseconds.
*/
public val lastDisabledTime: kotlin.Long = builder.lastDisabledTime
/**
* Describes the last time the user was activated. The value is determined as epoch time in milliseconds.
*/
public val lastEnabledTime: kotlin.Long = builder.lastEnabledTime
/**
* Describes the last time that the user logged into their account. The value is determined as epoch time in milliseconds.
*/
public val lastLoginTime: kotlin.Long = builder.lastLoginTime
/**
* Describes the last time the user details were updated. The value is determined as epoch time in milliseconds.
*/
public val lastModifiedTime: kotlin.Long = builder.lastModifiedTime
/**
* The last name of the user.
*/
public val lastName: kotlin.String? = builder.lastName
/**
* The current status of the user.
* + `CREATING` – The creation is in progress.
* + `ENABLED` – The user is created and is currently active.
* + `DISABLED` – The user is currently inactive.
*/
public val status: aws.sdk.kotlin.services.finspacedata.model.UserStatus? = builder.status
/**
* Indicates the type of user.
* + `SUPER_USER` – A user with permission to all the functionality and data in FinSpace.
*
* + `APP_USER` – A user with specific permissions in FinSpace. The users are assigned permissions by adding them to a permission group.
*/
public val type: aws.sdk.kotlin.services.finspacedata.model.UserType? = builder.type
/**
* The unique identifier for the user that is retrieved.
*/
public val userId: kotlin.String? = builder.userId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.finspacedata.model.GetUserResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetUserResponse(")
append("apiAccess=$apiAccess,")
append("apiAccessPrincipalArn=$apiAccessPrincipalArn,")
append("createTime=$createTime,")
append("emailAddress=*** Sensitive Data Redacted ***,")
append("firstName=*** Sensitive Data Redacted ***,")
append("lastDisabledTime=$lastDisabledTime,")
append("lastEnabledTime=$lastEnabledTime,")
append("lastLoginTime=$lastLoginTime,")
append("lastModifiedTime=$lastModifiedTime,")
append("lastName=*** Sensitive Data Redacted ***,")
append("status=$status,")
append("type=$type,")
append("userId=$userId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = apiAccess?.hashCode() ?: 0
result = 31 * result + (apiAccessPrincipalArn?.hashCode() ?: 0)
result = 31 * result + (createTime.hashCode())
result = 31 * result + (emailAddress?.hashCode() ?: 0)
result = 31 * result + (firstName?.hashCode() ?: 0)
result = 31 * result + (lastDisabledTime.hashCode())
result = 31 * result + (lastEnabledTime.hashCode())
result = 31 * result + (lastLoginTime.hashCode())
result = 31 * result + (lastModifiedTime.hashCode())
result = 31 * result + (lastName?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (userId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetUserResponse
if (apiAccess != other.apiAccess) return false
if (apiAccessPrincipalArn != other.apiAccessPrincipalArn) return false
if (createTime != other.createTime) return false
if (emailAddress != other.emailAddress) return false
if (firstName != other.firstName) return false
if (lastDisabledTime != other.lastDisabledTime) return false
if (lastEnabledTime != other.lastEnabledTime) return false
if (lastLoginTime != other.lastLoginTime) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (lastName != other.lastName) return false
if (status != other.status) return false
if (type != other.type) return false
if (userId != other.userId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.finspacedata.model.GetUserResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Indicates whether the user can use the `GetProgrammaticAccessCredentials` API to obtain credentials that can then be used to access other FinSpace Data API operations.
* + `ENABLED` – The user has permissions to use the APIs.
* + `DISABLED` – The user does not have permissions to use any APIs.
*/
public var apiAccess: aws.sdk.kotlin.services.finspacedata.model.ApiAccess? = null
/**
* The ARN identifier of an AWS user or role that is allowed to call the `GetProgrammaticAccessCredentials` API to obtain a credentials token for a specific FinSpace user. This must be an IAM role within your FinSpace account.
*/
public var apiAccessPrincipalArn: kotlin.String? = null
/**
* The timestamp at which the user was created in FinSpace. The value is determined as epoch time in milliseconds.
*/
public var createTime: kotlin.Long = 0L
/**
* The email address that is associated with the user.
*/
public var emailAddress: kotlin.String? = null
/**
* The first name of the user.
*/
public var firstName: kotlin.String? = null
/**
* Describes the last time the user was deactivated. The value is determined as epoch time in milliseconds.
*/
public var lastDisabledTime: kotlin.Long = 0L
/**
* Describes the last time the user was activated. The value is determined as epoch time in milliseconds.
*/
public var lastEnabledTime: kotlin.Long = 0L
/**
* Describes the last time that the user logged into their account. The value is determined as epoch time in milliseconds.
*/
public var lastLoginTime: kotlin.Long = 0L
/**
* Describes the last time the user details were updated. The value is determined as epoch time in milliseconds.
*/
public var lastModifiedTime: kotlin.Long = 0L
/**
* The last name of the user.
*/
public var lastName: kotlin.String? = null
/**
* The current status of the user.
* + `CREATING` – The creation is in progress.
* + `ENABLED` – The user is created and is currently active.
* + `DISABLED` – The user is currently inactive.
*/
public var status: aws.sdk.kotlin.services.finspacedata.model.UserStatus? = null
/**
* Indicates the type of user.
* + `SUPER_USER` – A user with permission to all the functionality and data in FinSpace.
*
* + `APP_USER` – A user with specific permissions in FinSpace. The users are assigned permissions by adding them to a permission group.
*/
public var type: aws.sdk.kotlin.services.finspacedata.model.UserType? = null
/**
* The unique identifier for the user that is retrieved.
*/
public var userId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.finspacedata.model.GetUserResponse) : this() {
this.apiAccess = x.apiAccess
this.apiAccessPrincipalArn = x.apiAccessPrincipalArn
this.createTime = x.createTime
this.emailAddress = x.emailAddress
this.firstName = x.firstName
this.lastDisabledTime = x.lastDisabledTime
this.lastEnabledTime = x.lastEnabledTime
this.lastLoginTime = x.lastLoginTime
this.lastModifiedTime = x.lastModifiedTime
this.lastName = x.lastName
this.status = x.status
this.type = x.type
this.userId = x.userId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.finspacedata.model.GetUserResponse = GetUserResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy