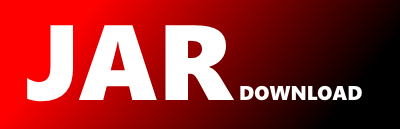
commonMain.aws.sdk.kotlin.services.firehose.model.SplunkDestinationConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of firehose-jvm Show documentation
Show all versions of firehose-jvm Show documentation
The AWS SDK for Kotlin client for Firehose
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.firehose.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Describes the configuration of a destination in Splunk.
*/
public class SplunkDestinationConfiguration private constructor(builder: Builder) {
/**
* The buffering options. If no value is specified, the default values for Splunk are used.
*/
public val bufferingHints: aws.sdk.kotlin.services.firehose.model.SplunkBufferingHints? = builder.bufferingHints
/**
* The Amazon CloudWatch logging options for your delivery stream.
*/
public val cloudWatchLoggingOptions: aws.sdk.kotlin.services.firehose.model.CloudWatchLoggingOptions? = builder.cloudWatchLoggingOptions
/**
* The amount of time that Firehose waits to receive an acknowledgment from Splunk after it sends it data. At the end of the timeout period, Firehose either tries to send the data again or considers it an error, based on your retry settings.
*/
public val hecAcknowledgmentTimeoutInSeconds: kotlin.Int? = builder.hecAcknowledgmentTimeoutInSeconds
/**
* The HTTP Event Collector (HEC) endpoint to which Firehose sends your data.
*/
public val hecEndpoint: kotlin.String = requireNotNull(builder.hecEndpoint) { "A non-null value must be provided for hecEndpoint" }
/**
* This type can be either "Raw" or "Event."
*/
public val hecEndpointType: aws.sdk.kotlin.services.firehose.model.HecEndpointType = requireNotNull(builder.hecEndpointType) { "A non-null value must be provided for hecEndpointType" }
/**
* This is a GUID that you obtain from your Splunk cluster when you create a new HEC endpoint.
*/
public val hecToken: kotlin.String? = builder.hecToken
/**
* The data processing configuration.
*/
public val processingConfiguration: aws.sdk.kotlin.services.firehose.model.ProcessingConfiguration? = builder.processingConfiguration
/**
* The retry behavior in case Firehose is unable to deliver data to Splunk, or if it doesn't receive an acknowledgment of receipt from Splunk.
*/
public val retryOptions: aws.sdk.kotlin.services.firehose.model.SplunkRetryOptions? = builder.retryOptions
/**
* Defines how documents should be delivered to Amazon S3. When set to `FailedEventsOnly`, Firehose writes any data that could not be indexed to the configured Amazon S3 destination. When set to `AllEvents`, Firehose delivers all incoming records to Amazon S3, and also writes failed documents to Amazon S3. The default value is `FailedEventsOnly`.
*
* You can update this backup mode from `FailedEventsOnly` to `AllEvents`. You can't update it from `AllEvents` to `FailedEventsOnly`.
*/
public val s3BackupMode: aws.sdk.kotlin.services.firehose.model.SplunkS3BackupMode? = builder.s3BackupMode
/**
* The configuration for the backup Amazon S3 location.
*/
public val s3Configuration: aws.sdk.kotlin.services.firehose.model.S3DestinationConfiguration? = builder.s3Configuration
/**
* The configuration that defines how you access secrets for Splunk.
*/
public val secretsManagerConfiguration: aws.sdk.kotlin.services.firehose.model.SecretsManagerConfiguration? = builder.secretsManagerConfiguration
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.firehose.model.SplunkDestinationConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SplunkDestinationConfiguration(")
append("bufferingHints=$bufferingHints,")
append("cloudWatchLoggingOptions=$cloudWatchLoggingOptions,")
append("hecAcknowledgmentTimeoutInSeconds=$hecAcknowledgmentTimeoutInSeconds,")
append("hecEndpoint=$hecEndpoint,")
append("hecEndpointType=$hecEndpointType,")
append("hecToken=$hecToken,")
append("processingConfiguration=$processingConfiguration,")
append("retryOptions=$retryOptions,")
append("s3BackupMode=$s3BackupMode,")
append("s3Configuration=$s3Configuration,")
append("secretsManagerConfiguration=$secretsManagerConfiguration")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bufferingHints?.hashCode() ?: 0
result = 31 * result + (cloudWatchLoggingOptions?.hashCode() ?: 0)
result = 31 * result + (hecAcknowledgmentTimeoutInSeconds ?: 0)
result = 31 * result + (hecEndpoint.hashCode())
result = 31 * result + (hecEndpointType.hashCode())
result = 31 * result + (hecToken?.hashCode() ?: 0)
result = 31 * result + (processingConfiguration?.hashCode() ?: 0)
result = 31 * result + (retryOptions?.hashCode() ?: 0)
result = 31 * result + (s3BackupMode?.hashCode() ?: 0)
result = 31 * result + (s3Configuration?.hashCode() ?: 0)
result = 31 * result + (secretsManagerConfiguration?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SplunkDestinationConfiguration
if (bufferingHints != other.bufferingHints) return false
if (cloudWatchLoggingOptions != other.cloudWatchLoggingOptions) return false
if (hecAcknowledgmentTimeoutInSeconds != other.hecAcknowledgmentTimeoutInSeconds) return false
if (hecEndpoint != other.hecEndpoint) return false
if (hecEndpointType != other.hecEndpointType) return false
if (hecToken != other.hecToken) return false
if (processingConfiguration != other.processingConfiguration) return false
if (retryOptions != other.retryOptions) return false
if (s3BackupMode != other.s3BackupMode) return false
if (s3Configuration != other.s3Configuration) return false
if (secretsManagerConfiguration != other.secretsManagerConfiguration) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.firehose.model.SplunkDestinationConfiguration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The buffering options. If no value is specified, the default values for Splunk are used.
*/
public var bufferingHints: aws.sdk.kotlin.services.firehose.model.SplunkBufferingHints? = null
/**
* The Amazon CloudWatch logging options for your delivery stream.
*/
public var cloudWatchLoggingOptions: aws.sdk.kotlin.services.firehose.model.CloudWatchLoggingOptions? = null
/**
* The amount of time that Firehose waits to receive an acknowledgment from Splunk after it sends it data. At the end of the timeout period, Firehose either tries to send the data again or considers it an error, based on your retry settings.
*/
public var hecAcknowledgmentTimeoutInSeconds: kotlin.Int? = null
/**
* The HTTP Event Collector (HEC) endpoint to which Firehose sends your data.
*/
public var hecEndpoint: kotlin.String? = null
/**
* This type can be either "Raw" or "Event."
*/
public var hecEndpointType: aws.sdk.kotlin.services.firehose.model.HecEndpointType? = null
/**
* This is a GUID that you obtain from your Splunk cluster when you create a new HEC endpoint.
*/
public var hecToken: kotlin.String? = null
/**
* The data processing configuration.
*/
public var processingConfiguration: aws.sdk.kotlin.services.firehose.model.ProcessingConfiguration? = null
/**
* The retry behavior in case Firehose is unable to deliver data to Splunk, or if it doesn't receive an acknowledgment of receipt from Splunk.
*/
public var retryOptions: aws.sdk.kotlin.services.firehose.model.SplunkRetryOptions? = null
/**
* Defines how documents should be delivered to Amazon S3. When set to `FailedEventsOnly`, Firehose writes any data that could not be indexed to the configured Amazon S3 destination. When set to `AllEvents`, Firehose delivers all incoming records to Amazon S3, and also writes failed documents to Amazon S3. The default value is `FailedEventsOnly`.
*
* You can update this backup mode from `FailedEventsOnly` to `AllEvents`. You can't update it from `AllEvents` to `FailedEventsOnly`.
*/
public var s3BackupMode: aws.sdk.kotlin.services.firehose.model.SplunkS3BackupMode? = null
/**
* The configuration for the backup Amazon S3 location.
*/
public var s3Configuration: aws.sdk.kotlin.services.firehose.model.S3DestinationConfiguration? = null
/**
* The configuration that defines how you access secrets for Splunk.
*/
public var secretsManagerConfiguration: aws.sdk.kotlin.services.firehose.model.SecretsManagerConfiguration? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.firehose.model.SplunkDestinationConfiguration) : this() {
this.bufferingHints = x.bufferingHints
this.cloudWatchLoggingOptions = x.cloudWatchLoggingOptions
this.hecAcknowledgmentTimeoutInSeconds = x.hecAcknowledgmentTimeoutInSeconds
this.hecEndpoint = x.hecEndpoint
this.hecEndpointType = x.hecEndpointType
this.hecToken = x.hecToken
this.processingConfiguration = x.processingConfiguration
this.retryOptions = x.retryOptions
this.s3BackupMode = x.s3BackupMode
this.s3Configuration = x.s3Configuration
this.secretsManagerConfiguration = x.secretsManagerConfiguration
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.firehose.model.SplunkDestinationConfiguration = SplunkDestinationConfiguration(this)
/**
* construct an [aws.sdk.kotlin.services.firehose.model.SplunkBufferingHints] inside the given [block]
*/
public fun bufferingHints(block: aws.sdk.kotlin.services.firehose.model.SplunkBufferingHints.Builder.() -> kotlin.Unit) {
this.bufferingHints = aws.sdk.kotlin.services.firehose.model.SplunkBufferingHints.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.firehose.model.CloudWatchLoggingOptions] inside the given [block]
*/
public fun cloudWatchLoggingOptions(block: aws.sdk.kotlin.services.firehose.model.CloudWatchLoggingOptions.Builder.() -> kotlin.Unit) {
this.cloudWatchLoggingOptions = aws.sdk.kotlin.services.firehose.model.CloudWatchLoggingOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.firehose.model.ProcessingConfiguration] inside the given [block]
*/
public fun processingConfiguration(block: aws.sdk.kotlin.services.firehose.model.ProcessingConfiguration.Builder.() -> kotlin.Unit) {
this.processingConfiguration = aws.sdk.kotlin.services.firehose.model.ProcessingConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.firehose.model.SplunkRetryOptions] inside the given [block]
*/
public fun retryOptions(block: aws.sdk.kotlin.services.firehose.model.SplunkRetryOptions.Builder.() -> kotlin.Unit) {
this.retryOptions = aws.sdk.kotlin.services.firehose.model.SplunkRetryOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.firehose.model.S3DestinationConfiguration] inside the given [block]
*/
public fun s3Configuration(block: aws.sdk.kotlin.services.firehose.model.S3DestinationConfiguration.Builder.() -> kotlin.Unit) {
this.s3Configuration = aws.sdk.kotlin.services.firehose.model.S3DestinationConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.firehose.model.SecretsManagerConfiguration] inside the given [block]
*/
public fun secretsManagerConfiguration(block: aws.sdk.kotlin.services.firehose.model.SecretsManagerConfiguration.Builder.() -> kotlin.Unit) {
this.secretsManagerConfiguration = aws.sdk.kotlin.services.firehose.model.SecretsManagerConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (hecEndpoint == null) hecEndpoint = ""
if (hecEndpointType == null) hecEndpointType = HecEndpointType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy