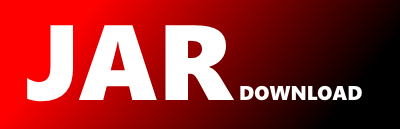
commonMain.aws.sdk.kotlin.services.freetier.model.Expression.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freetier-jvm Show documentation
Show all versions of freetier-jvm Show documentation
The AWS SDK for Kotlin client for FreeTier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.freetier.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Use `Expression` to filter in the `GetFreeTierUsage` API operation.
*
* You can use the following patterns:
* + Simple dimension values (`Dimensions` root operator)
* + Complex expressions with logical operators (`AND`, `NOT`, and `OR` root operators).
*
* For *simple dimension values*, you can set the dimension name, values, and match type for the filters that you plan to use.
*
* **Example for simple dimension values**
*
* You can filter to match exactly for `REGION==us-east-1 OR REGION==us-west-1`.
*
* The corresponding `Expression` appears like the following: `{ "Dimensions": { "Key": "REGION", "Values": [ "us-east-1", "us-west-1" ], "MatchOptions": ["EQUALS"] } }`
*
* As shown in the previous example, lists of dimension values are combined with `OR` when you apply the filter.
*
* For *complex expressions with logical operators*, you can have nested expressions to use the logical operators and specify advanced filtering.
*
* **Example for complex expressions with logical operators**
*
* You can filter by `((REGION == us-east-1 OR REGION == us-west-1) OR (SERVICE CONTAINS AWSLambda)) AND (USAGE_TYPE !CONTAINS DataTransfer)`.
*
* The corresponding `Expression` appears like the following: `{ "And": [ {"Or": [ {"Dimensions": { "Key": "REGION", "Values": [ "us-east-1", "us-west-1" ], "MatchOptions": ["EQUALS"] }}, {"Dimensions": { "Key": "SERVICE", "Values": ["AWSLambda"], "MatchOptions": ["CONTAINS"] } } ]}, {"Not": {"Dimensions": { "Key": "USAGE_TYPE", "Values": ["DataTransfer"], "MatchOptions": ["CONTAINS"] }}} ] }`
*
* In the following **Contents**, you must specify exactly one of the following root operators.
*/
public class Expression private constructor(builder: Builder) {
/**
* Return results that match all `Expressions` that you specified in the array.
*/
public val and: List? = builder.and
/**
* The specific dimension, values, and match type to filter objects with.
*/
public val dimensions: aws.sdk.kotlin.services.freetier.model.DimensionValues? = builder.dimensions
/**
* Return results that don’t match the `Expression` that you specified.
*/
public val not: aws.sdk.kotlin.services.freetier.model.Expression? = builder.not
/**
* Return results that match any of the `Expressions` that you specified. in the array.
*/
public val or: List? = builder.or
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.freetier.model.Expression = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Expression(")
append("and=$and,")
append("dimensions=$dimensions,")
append("not=$not,")
append("or=$or")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = and?.hashCode() ?: 0
result = 31 * result + (dimensions?.hashCode() ?: 0)
result = 31 * result + (not?.hashCode() ?: 0)
result = 31 * result + (or?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Expression
if (and != other.and) return false
if (dimensions != other.dimensions) return false
if (not != other.not) return false
if (or != other.or) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.freetier.model.Expression = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Return results that match all `Expressions` that you specified in the array.
*/
public var and: List? = null
/**
* The specific dimension, values, and match type to filter objects with.
*/
public var dimensions: aws.sdk.kotlin.services.freetier.model.DimensionValues? = null
/**
* Return results that don’t match the `Expression` that you specified.
*/
public var not: aws.sdk.kotlin.services.freetier.model.Expression? = null
/**
* Return results that match any of the `Expressions` that you specified. in the array.
*/
public var or: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.freetier.model.Expression) : this() {
this.and = x.and
this.dimensions = x.dimensions
this.not = x.not
this.or = x.or
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.freetier.model.Expression = Expression(this)
/**
* construct an [aws.sdk.kotlin.services.freetier.model.DimensionValues] inside the given [block]
*/
public fun dimensions(block: aws.sdk.kotlin.services.freetier.model.DimensionValues.Builder.() -> kotlin.Unit) {
this.dimensions = aws.sdk.kotlin.services.freetier.model.DimensionValues.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.freetier.model.Expression] inside the given [block]
*/
public fun not(block: aws.sdk.kotlin.services.freetier.model.Expression.Builder.() -> kotlin.Unit) {
this.not = aws.sdk.kotlin.services.freetier.model.Expression.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy