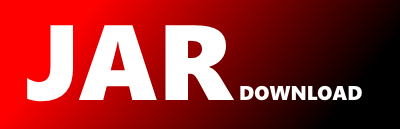
commonMain.aws.sdk.kotlin.services.freetier.model.FreeTierUsage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of freetier-jvm Show documentation
Show all versions of freetier-jvm Show documentation
The AWS SDK for Kotlin client for FreeTier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.freetier.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Consists of a Amazon Web Services Free Tier offer’s metadata and your data usage for the offer.
*/
public class FreeTierUsage private constructor(builder: Builder) {
/**
* Describes the actual usage accrued month-to-day (MTD) that you've used so far.
*/
public val actualUsageAmount: kotlin.Double = builder.actualUsageAmount
/**
* The description of the Free Tier offer.
*/
public val description: kotlin.String? = builder.description
/**
* Describes the forecasted usage by the month that you're expected to use.
*/
public val forecastedUsageAmount: kotlin.Double = builder.forecastedUsageAmount
/**
* Describes the type of the Free Tier offer. For example, the offer can be `"12 Months Free"`, `"Always Free"`, and `"Free Trial"`.
*/
public val freeTierType: kotlin.String? = builder.freeTierType
/**
* Describes the maximum usage allowed in Free Tier.
*/
public val limit: kotlin.Double = builder.limit
/**
* Describes `usageType` more granularly with the specific Amazon Web Service API operation. For example, this can be the `RunInstances` API operation for Amazon Elastic Compute Cloud.
*/
public val operation: kotlin.String? = builder.operation
/**
* Describes the Amazon Web Services Region for which this offer is applicable
*/
public val region: kotlin.String? = builder.region
/**
* The name of the Amazon Web Service providing the Free Tier offer. For example, this can be Amazon Elastic Compute Cloud.
*/
public val service: kotlin.String? = builder.service
/**
* Describes the unit of the `usageType`, such as `Hrs`.
*/
public val unit: kotlin.String? = builder.unit
/**
* Describes the usage details of the offer. For example, this might be `Global-BoxUsage:freetrial`.
*/
public val usageType: kotlin.String? = builder.usageType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.freetier.model.FreeTierUsage = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("FreeTierUsage(")
append("actualUsageAmount=$actualUsageAmount,")
append("description=$description,")
append("forecastedUsageAmount=$forecastedUsageAmount,")
append("freeTierType=$freeTierType,")
append("limit=$limit,")
append("operation=$operation,")
append("region=$region,")
append("service=$service,")
append("unit=$unit,")
append("usageType=$usageType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actualUsageAmount.hashCode()
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (forecastedUsageAmount.hashCode())
result = 31 * result + (freeTierType?.hashCode() ?: 0)
result = 31 * result + (limit.hashCode())
result = 31 * result + (operation?.hashCode() ?: 0)
result = 31 * result + (region?.hashCode() ?: 0)
result = 31 * result + (service?.hashCode() ?: 0)
result = 31 * result + (unit?.hashCode() ?: 0)
result = 31 * result + (usageType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as FreeTierUsage
if (!(actualUsageAmount?.equals(other.actualUsageAmount) ?: (other.actualUsageAmount == null))) return false
if (description != other.description) return false
if (!(forecastedUsageAmount?.equals(other.forecastedUsageAmount) ?: (other.forecastedUsageAmount == null))) return false
if (freeTierType != other.freeTierType) return false
if (!(limit?.equals(other.limit) ?: (other.limit == null))) return false
if (operation != other.operation) return false
if (region != other.region) return false
if (service != other.service) return false
if (unit != other.unit) return false
if (usageType != other.usageType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.freetier.model.FreeTierUsage = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Describes the actual usage accrued month-to-day (MTD) that you've used so far.
*/
public var actualUsageAmount: kotlin.Double = 0.0
/**
* The description of the Free Tier offer.
*/
public var description: kotlin.String? = null
/**
* Describes the forecasted usage by the month that you're expected to use.
*/
public var forecastedUsageAmount: kotlin.Double = 0.0
/**
* Describes the type of the Free Tier offer. For example, the offer can be `"12 Months Free"`, `"Always Free"`, and `"Free Trial"`.
*/
public var freeTierType: kotlin.String? = null
/**
* Describes the maximum usage allowed in Free Tier.
*/
public var limit: kotlin.Double = 0.0
/**
* Describes `usageType` more granularly with the specific Amazon Web Service API operation. For example, this can be the `RunInstances` API operation for Amazon Elastic Compute Cloud.
*/
public var operation: kotlin.String? = null
/**
* Describes the Amazon Web Services Region for which this offer is applicable
*/
public var region: kotlin.String? = null
/**
* The name of the Amazon Web Service providing the Free Tier offer. For example, this can be Amazon Elastic Compute Cloud.
*/
public var service: kotlin.String? = null
/**
* Describes the unit of the `usageType`, such as `Hrs`.
*/
public var unit: kotlin.String? = null
/**
* Describes the usage details of the offer. For example, this might be `Global-BoxUsage:freetrial`.
*/
public var usageType: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.freetier.model.FreeTierUsage) : this() {
this.actualUsageAmount = x.actualUsageAmount
this.description = x.description
this.forecastedUsageAmount = x.forecastedUsageAmount
this.freeTierType = x.freeTierType
this.limit = x.limit
this.operation = x.operation
this.region = x.region
this.service = x.service
this.unit = x.unit
this.usageType = x.usageType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.freetier.model.FreeTierUsage = FreeTierUsage(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy