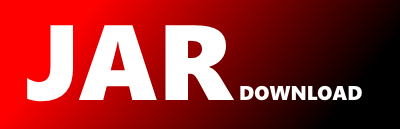
commonMain.aws.sdk.kotlin.services.gamelift.model.CreateGameSessionQueueRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateGameSessionQueueRequest private constructor(builder: Builder) {
/**
* Information to be added to all events that are related to this game session queue.
*/
public val customEventData: kotlin.String? = builder.customEventData
/**
* A list of fleets and/or fleet aliases that can be used to fulfill game session placement requests in the queue. Destinations are identified by either a fleet ARN or a fleet alias ARN, and are listed in order of placement preference.
*/
public val destinations: List? = builder.destinations
/**
* A list of locations where a queue is allowed to place new game sessions. Locations are specified in the form of Amazon Web Services Region codes, such as `us-west-2`. If this parameter is not set, game sessions can be placed in any queue location.
*/
public val filterConfiguration: aws.sdk.kotlin.services.gamelift.model.FilterConfiguration? = builder.filterConfiguration
/**
* A descriptive label that is associated with game session queue. Queue names must be unique within each Region.
*/
public val name: kotlin.String? = builder.name
/**
* An SNS topic ARN that is set up to receive game session placement notifications. See [ Setting up notifications for game session placement](https://docs.aws.amazon.com/gamelift/latest/developerguide/queue-notification.html).
*/
public val notificationTarget: kotlin.String? = builder.notificationTarget
/**
* A set of policies that enforce a sliding cap on player latency when processing game sessions placement requests. Use multiple policies to gradually relax the cap over time if Amazon GameLift can't make a placement. Policies are evaluated in order starting with the lowest maximum latency value.
*/
public val playerLatencyPolicies: List? = builder.playerLatencyPolicies
/**
* Custom settings to use when prioritizing destinations and locations for game session placements. This configuration replaces the FleetIQ default prioritization process. Priority types that are not explicitly named will be automatically applied at the end of the prioritization process.
*/
public val priorityConfiguration: aws.sdk.kotlin.services.gamelift.model.PriorityConfiguration? = builder.priorityConfiguration
/**
* A list of labels to assign to the new game session queue resource. Tags are developer-defined key-value pairs. Tagging Amazon Web Services resources are useful for resource management, access management and cost allocation. For more information, see [ Tagging Amazon Web Services Resources](https://docs.aws.amazon.com/general/latest/gr/aws_tagging.html) in the *Amazon Web Services General Reference*.
*/
public val tags: List? = builder.tags
/**
* The maximum time, in seconds, that a new game session placement request remains in the queue. When a request exceeds this time, the game session placement changes to a `TIMED_OUT` status.
*/
public val timeoutInSeconds: kotlin.Int? = builder.timeoutInSeconds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.CreateGameSessionQueueRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateGameSessionQueueRequest(")
append("customEventData=$customEventData,")
append("destinations=$destinations,")
append("filterConfiguration=$filterConfiguration,")
append("name=$name,")
append("notificationTarget=$notificationTarget,")
append("playerLatencyPolicies=$playerLatencyPolicies,")
append("priorityConfiguration=$priorityConfiguration,")
append("tags=$tags,")
append("timeoutInSeconds=$timeoutInSeconds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = customEventData?.hashCode() ?: 0
result = 31 * result + (destinations?.hashCode() ?: 0)
result = 31 * result + (filterConfiguration?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (notificationTarget?.hashCode() ?: 0)
result = 31 * result + (playerLatencyPolicies?.hashCode() ?: 0)
result = 31 * result + (priorityConfiguration?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (timeoutInSeconds ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateGameSessionQueueRequest
if (customEventData != other.customEventData) return false
if (destinations != other.destinations) return false
if (filterConfiguration != other.filterConfiguration) return false
if (name != other.name) return false
if (notificationTarget != other.notificationTarget) return false
if (playerLatencyPolicies != other.playerLatencyPolicies) return false
if (priorityConfiguration != other.priorityConfiguration) return false
if (tags != other.tags) return false
if (timeoutInSeconds != other.timeoutInSeconds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.CreateGameSessionQueueRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Information to be added to all events that are related to this game session queue.
*/
public var customEventData: kotlin.String? = null
/**
* A list of fleets and/or fleet aliases that can be used to fulfill game session placement requests in the queue. Destinations are identified by either a fleet ARN or a fleet alias ARN, and are listed in order of placement preference.
*/
public var destinations: List? = null
/**
* A list of locations where a queue is allowed to place new game sessions. Locations are specified in the form of Amazon Web Services Region codes, such as `us-west-2`. If this parameter is not set, game sessions can be placed in any queue location.
*/
public var filterConfiguration: aws.sdk.kotlin.services.gamelift.model.FilterConfiguration? = null
/**
* A descriptive label that is associated with game session queue. Queue names must be unique within each Region.
*/
public var name: kotlin.String? = null
/**
* An SNS topic ARN that is set up to receive game session placement notifications. See [ Setting up notifications for game session placement](https://docs.aws.amazon.com/gamelift/latest/developerguide/queue-notification.html).
*/
public var notificationTarget: kotlin.String? = null
/**
* A set of policies that enforce a sliding cap on player latency when processing game sessions placement requests. Use multiple policies to gradually relax the cap over time if Amazon GameLift can't make a placement. Policies are evaluated in order starting with the lowest maximum latency value.
*/
public var playerLatencyPolicies: List? = null
/**
* Custom settings to use when prioritizing destinations and locations for game session placements. This configuration replaces the FleetIQ default prioritization process. Priority types that are not explicitly named will be automatically applied at the end of the prioritization process.
*/
public var priorityConfiguration: aws.sdk.kotlin.services.gamelift.model.PriorityConfiguration? = null
/**
* A list of labels to assign to the new game session queue resource. Tags are developer-defined key-value pairs. Tagging Amazon Web Services resources are useful for resource management, access management and cost allocation. For more information, see [ Tagging Amazon Web Services Resources](https://docs.aws.amazon.com/general/latest/gr/aws_tagging.html) in the *Amazon Web Services General Reference*.
*/
public var tags: List? = null
/**
* The maximum time, in seconds, that a new game session placement request remains in the queue. When a request exceeds this time, the game session placement changes to a `TIMED_OUT` status.
*/
public var timeoutInSeconds: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.CreateGameSessionQueueRequest) : this() {
this.customEventData = x.customEventData
this.destinations = x.destinations
this.filterConfiguration = x.filterConfiguration
this.name = x.name
this.notificationTarget = x.notificationTarget
this.playerLatencyPolicies = x.playerLatencyPolicies
this.priorityConfiguration = x.priorityConfiguration
this.tags = x.tags
this.timeoutInSeconds = x.timeoutInSeconds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.CreateGameSessionQueueRequest = CreateGameSessionQueueRequest(this)
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.FilterConfiguration] inside the given [block]
*/
public fun filterConfiguration(block: aws.sdk.kotlin.services.gamelift.model.FilterConfiguration.Builder.() -> kotlin.Unit) {
this.filterConfiguration = aws.sdk.kotlin.services.gamelift.model.FilterConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.PriorityConfiguration] inside the given [block]
*/
public fun priorityConfiguration(block: aws.sdk.kotlin.services.gamelift.model.PriorityConfiguration.Builder.() -> kotlin.Unit) {
this.priorityConfiguration = aws.sdk.kotlin.services.gamelift.model.PriorityConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy