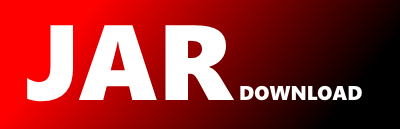
commonMain.aws.sdk.kotlin.services.gamelift.model.CreateScriptRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gamelift-jvm Show documentation
Show all versions of gamelift-jvm Show documentation
The AWS SDK for Kotlin client for GameLift
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateScriptRequest private constructor(builder: Builder) {
/**
* A descriptive label that is associated with a script. Script names do not need to be unique. You can use [UpdateScript](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UpdateScript.html) to change this value later.
*/
public val name: kotlin.String? = builder.name
/**
* The location of the Amazon S3 bucket where a zipped file containing your Realtime scripts is stored. The storage location must specify the Amazon S3 bucket name, the zip file name (the "key"), and a role ARN that allows Amazon GameLift to access the Amazon S3 storage location. The S3 bucket must be in the same Region where you want to create a new script. By default, Amazon GameLift uploads the latest version of the zip file; if you have S3 object versioning turned on, you can use the `ObjectVersion` parameter to specify an earlier version.
*/
public val storageLocation: aws.sdk.kotlin.services.gamelift.model.S3Location? = builder.storageLocation
/**
* A list of labels to assign to the new script resource. Tags are developer-defined key-value pairs. Tagging Amazon Web Services resources are useful for resource management, access management and cost allocation. For more information, see [ Tagging Amazon Web Services Resources](https://docs.aws.amazon.com/general/latest/gr/aws_tagging.html) in the *Amazon Web Services General Reference*. Once the resource is created, you can use [TagResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_TagResource.html), [UntagResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UntagResource.html), and [ListTagsForResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_ListTagsForResource.html) to add, remove, and view tags. The maximum tag limit may be lower than stated. See the Amazon Web Services General Reference for actual tagging limits.
*/
public val tags: List? = builder.tags
/**
* Version information that is associated with a build or script. Version strings do not need to be unique. You can use [UpdateScript](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UpdateScript.html) to change this value later.
*/
public val version: kotlin.String? = builder.version
/**
* A data object containing your Realtime scripts and dependencies as a zip file. The zip file can have one or multiple files. Maximum size of a zip file is 5 MB.
*
* When using the Amazon Web Services CLI tool to create a script, this parameter is set to the zip file name. It must be prepended with the string "fileb://" to indicate that the file data is a binary object. For example: `--zip-file fileb://myRealtimeScript.zip`.
*/
public val zipFile: kotlin.ByteArray? = builder.zipFile
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.CreateScriptRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateScriptRequest(")
append("name=$name,")
append("storageLocation=$storageLocation,")
append("tags=$tags,")
append("version=$version,")
append("zipFile=$zipFile")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = name?.hashCode() ?: 0
result = 31 * result + (storageLocation?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (version?.hashCode() ?: 0)
result = 31 * result + (zipFile?.contentHashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateScriptRequest
if (name != other.name) return false
if (storageLocation != other.storageLocation) return false
if (tags != other.tags) return false
if (version != other.version) return false
if (zipFile != null) {
if (other.zipFile == null) return false
if (!zipFile.contentEquals(other.zipFile)) return false
} else if (other.zipFile != null) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.CreateScriptRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A descriptive label that is associated with a script. Script names do not need to be unique. You can use [UpdateScript](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UpdateScript.html) to change this value later.
*/
public var name: kotlin.String? = null
/**
* The location of the Amazon S3 bucket where a zipped file containing your Realtime scripts is stored. The storage location must specify the Amazon S3 bucket name, the zip file name (the "key"), and a role ARN that allows Amazon GameLift to access the Amazon S3 storage location. The S3 bucket must be in the same Region where you want to create a new script. By default, Amazon GameLift uploads the latest version of the zip file; if you have S3 object versioning turned on, you can use the `ObjectVersion` parameter to specify an earlier version.
*/
public var storageLocation: aws.sdk.kotlin.services.gamelift.model.S3Location? = null
/**
* A list of labels to assign to the new script resource. Tags are developer-defined key-value pairs. Tagging Amazon Web Services resources are useful for resource management, access management and cost allocation. For more information, see [ Tagging Amazon Web Services Resources](https://docs.aws.amazon.com/general/latest/gr/aws_tagging.html) in the *Amazon Web Services General Reference*. Once the resource is created, you can use [TagResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_TagResource.html), [UntagResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UntagResource.html), and [ListTagsForResource](https://docs.aws.amazon.com/gamelift/latest/apireference/API_ListTagsForResource.html) to add, remove, and view tags. The maximum tag limit may be lower than stated. See the Amazon Web Services General Reference for actual tagging limits.
*/
public var tags: List? = null
/**
* Version information that is associated with a build or script. Version strings do not need to be unique. You can use [UpdateScript](https://docs.aws.amazon.com/gamelift/latest/apireference/API_UpdateScript.html) to change this value later.
*/
public var version: kotlin.String? = null
/**
* A data object containing your Realtime scripts and dependencies as a zip file. The zip file can have one or multiple files. Maximum size of a zip file is 5 MB.
*
* When using the Amazon Web Services CLI tool to create a script, this parameter is set to the zip file name. It must be prepended with the string "fileb://" to indicate that the file data is a binary object. For example: `--zip-file fileb://myRealtimeScript.zip`.
*/
public var zipFile: kotlin.ByteArray? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.CreateScriptRequest) : this() {
this.name = x.name
this.storageLocation = x.storageLocation
this.tags = x.tags
this.version = x.version
this.zipFile = x.zipFile
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.CreateScriptRequest = CreateScriptRequest(this)
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.S3Location] inside the given [block]
*/
public fun storageLocation(block: aws.sdk.kotlin.services.gamelift.model.S3Location.Builder.() -> kotlin.Unit) {
this.storageLocation = aws.sdk.kotlin.services.gamelift.model.S3Location.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy