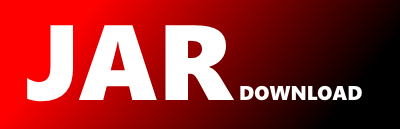
commonMain.aws.sdk.kotlin.services.gamelift.model.DescribeFleetCapacityRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
public class DescribeFleetCapacityRequest private constructor(builder: Builder) {
/**
* A unique identifier for the fleet to retrieve capacity information for. You can use either the fleet ID or ARN value. Leave this parameter empty to retrieve capacity information for all fleets.
*/
public val fleetIds: List? = builder.fleetIds
/**
* The maximum number of results to return. Use this parameter with `NextToken` to get results as a set of sequential pages. This parameter is ignored when the request specifies one or a list of fleet IDs.
*/
public val limit: kotlin.Int? = builder.limit
/**
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a previous call to this operation. To start at the beginning of the result set, do not specify a value. This parameter is ignored when the request specifies one or a list of fleet IDs.
*/
public val nextToken: kotlin.String? = builder.nextToken
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.DescribeFleetCapacityRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeFleetCapacityRequest(")
append("fleetIds=$fleetIds,")
append("limit=$limit,")
append("nextToken=$nextToken")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fleetIds?.hashCode() ?: 0
result = 31 * result + (limit ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeFleetCapacityRequest
if (fleetIds != other.fleetIds) return false
if (limit != other.limit) return false
if (nextToken != other.nextToken) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.DescribeFleetCapacityRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A unique identifier for the fleet to retrieve capacity information for. You can use either the fleet ID or ARN value. Leave this parameter empty to retrieve capacity information for all fleets.
*/
public var fleetIds: List? = null
/**
* The maximum number of results to return. Use this parameter with `NextToken` to get results as a set of sequential pages. This parameter is ignored when the request specifies one or a list of fleet IDs.
*/
public var limit: kotlin.Int? = null
/**
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a previous call to this operation. To start at the beginning of the result set, do not specify a value. This parameter is ignored when the request specifies one or a list of fleet IDs.
*/
public var nextToken: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.DescribeFleetCapacityRequest) : this() {
this.fleetIds = x.fleetIds
this.limit = x.limit
this.nextToken = x.nextToken
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.DescribeFleetCapacityRequest = DescribeFleetCapacityRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy