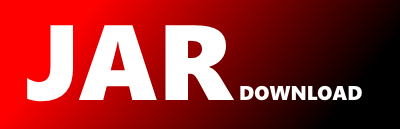
commonMain.aws.sdk.kotlin.services.gamelift.model.DescribeMatchmakingConfigurationsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
public class DescribeMatchmakingConfigurationsRequest private constructor(builder: Builder) {
/**
* The maximum number of results to return. Use this parameter with `NextToken` to get results as a set of sequential pages. This parameter is limited to 10.
*/
public val limit: kotlin.Int? = builder.limit
/**
* A unique identifier for the matchmaking configuration(s) to retrieve. You can use either the configuration name or ARN value. To request all existing configurations, leave this parameter empty.
*/
public val names: List? = builder.names
/**
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a previous call to this operation. To start at the beginning of the result set, do not specify a value.
*/
public val nextToken: kotlin.String? = builder.nextToken
/**
* A unique identifier for the matchmaking rule set. You can use either the rule set name or ARN value. Use this parameter to retrieve all matchmaking configurations that use this rule set.
*/
public val ruleSetName: kotlin.String? = builder.ruleSetName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.DescribeMatchmakingConfigurationsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeMatchmakingConfigurationsRequest(")
append("limit=$limit,")
append("names=$names,")
append("nextToken=$nextToken,")
append("ruleSetName=$ruleSetName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = limit ?: 0
result = 31 * result + (names?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (ruleSetName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeMatchmakingConfigurationsRequest
if (limit != other.limit) return false
if (names != other.names) return false
if (nextToken != other.nextToken) return false
if (ruleSetName != other.ruleSetName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.DescribeMatchmakingConfigurationsRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The maximum number of results to return. Use this parameter with `NextToken` to get results as a set of sequential pages. This parameter is limited to 10.
*/
public var limit: kotlin.Int? = null
/**
* A unique identifier for the matchmaking configuration(s) to retrieve. You can use either the configuration name or ARN value. To request all existing configurations, leave this parameter empty.
*/
public var names: List? = null
/**
* A token that indicates the start of the next sequential page of results. Use the token that is returned with a previous call to this operation. To start at the beginning of the result set, do not specify a value.
*/
public var nextToken: kotlin.String? = null
/**
* A unique identifier for the matchmaking rule set. You can use either the rule set name or ARN value. Use this parameter to retrieve all matchmaking configurations that use this rule set.
*/
public var ruleSetName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.DescribeMatchmakingConfigurationsRequest) : this() {
this.limit = x.limit
this.names = x.names
this.nextToken = x.nextToken
this.ruleSetName = x.ruleSetName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.DescribeMatchmakingConfigurationsRequest = DescribeMatchmakingConfigurationsRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy