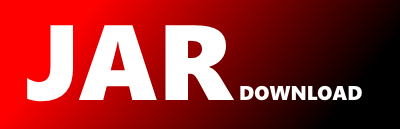
commonMain.aws.sdk.kotlin.services.gamelift.model.GameServerContainerDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gamelift-jvm Show documentation
Show all versions of gamelift-jvm Show documentation
The AWS SDK for Kotlin client for GameLift
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Describes the game server container in an existing game server container group. A game server container identifies a container image with your game server build. A game server container is automatically considered essential; if an essential container fails, the entire container group restarts.
*
* You can update a container definition and deploy the updates to an existing fleet. When creating or updating a game server container group definition, use the property GameServerContainerDefinitionInput.
*
* **Part of:**ContainerGroupDefinition
*
* **Returned by:**DescribeContainerGroupDefinition, ListContainerGroupDefinitions, UpdateContainerGroupDefinition
*/
public class GameServerContainerDefinition private constructor(builder: Builder) {
/**
* The container definition identifier. Container names are unique within a container group definition.
*/
public val containerName: kotlin.String? = builder.containerName
/**
* Indicates that the container relies on the status of other containers in the same container group during startup and shutdown sequences. A container might have dependencies on multiple containers.
*/
public val dependsOn: List? = builder.dependsOn
/**
* A set of environment variables that's passed to the container on startup. See the [ContainerDefinition::environment](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_ContainerDefinition.html#ECS-Type-ContainerDefinition-environment) parameter in the *Amazon Elastic Container Service API Reference*.
*/
public val environmentOverride: List? = builder.environmentOverride
/**
* The URI to the image that Amazon GameLift uses when deploying this container to a container fleet. For a more specific identifier, see `ResolvedImageDigest`.
*/
public val imageUri: kotlin.String? = builder.imageUri
/**
* A mount point that binds a path inside the container to a file or directory on the host system and lets it access the file or directory.
*/
public val mountPoints: List? = builder.mountPoints
/**
* The set of ports that are available to bind to processes in the container. For example, a game server process requires a container port to allow game clients to connect to it. Container ports aren't directly accessed by inbound traffic. Amazon GameLift maps these container ports to externally accessible connection ports, which are assigned as needed from the container fleet's `ConnectionPortRange`.
*/
public val portConfiguration: aws.sdk.kotlin.services.gamelift.model.ContainerPortConfiguration? = builder.portConfiguration
/**
* A unique and immutable identifier for the container image. The digest is a SHA 256 hash of the container image manifest.
*/
public val resolvedImageDigest: kotlin.String? = builder.resolvedImageDigest
/**
* The Amazon GameLift server SDK version that the game server is integrated with. Only game servers using 5.2.0 or higher are compatible with container fleets.
*/
public val serverSdkVersion: kotlin.String? = builder.serverSdkVersion
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.GameServerContainerDefinition = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GameServerContainerDefinition(")
append("containerName=$containerName,")
append("dependsOn=$dependsOn,")
append("environmentOverride=$environmentOverride,")
append("imageUri=$imageUri,")
append("mountPoints=$mountPoints,")
append("portConfiguration=$portConfiguration,")
append("resolvedImageDigest=$resolvedImageDigest,")
append("serverSdkVersion=$serverSdkVersion")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = containerName?.hashCode() ?: 0
result = 31 * result + (dependsOn?.hashCode() ?: 0)
result = 31 * result + (environmentOverride?.hashCode() ?: 0)
result = 31 * result + (imageUri?.hashCode() ?: 0)
result = 31 * result + (mountPoints?.hashCode() ?: 0)
result = 31 * result + (portConfiguration?.hashCode() ?: 0)
result = 31 * result + (resolvedImageDigest?.hashCode() ?: 0)
result = 31 * result + (serverSdkVersion?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GameServerContainerDefinition
if (containerName != other.containerName) return false
if (dependsOn != other.dependsOn) return false
if (environmentOverride != other.environmentOverride) return false
if (imageUri != other.imageUri) return false
if (mountPoints != other.mountPoints) return false
if (portConfiguration != other.portConfiguration) return false
if (resolvedImageDigest != other.resolvedImageDigest) return false
if (serverSdkVersion != other.serverSdkVersion) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.GameServerContainerDefinition = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The container definition identifier. Container names are unique within a container group definition.
*/
public var containerName: kotlin.String? = null
/**
* Indicates that the container relies on the status of other containers in the same container group during startup and shutdown sequences. A container might have dependencies on multiple containers.
*/
public var dependsOn: List? = null
/**
* A set of environment variables that's passed to the container on startup. See the [ContainerDefinition::environment](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_ContainerDefinition.html#ECS-Type-ContainerDefinition-environment) parameter in the *Amazon Elastic Container Service API Reference*.
*/
public var environmentOverride: List? = null
/**
* The URI to the image that Amazon GameLift uses when deploying this container to a container fleet. For a more specific identifier, see `ResolvedImageDigest`.
*/
public var imageUri: kotlin.String? = null
/**
* A mount point that binds a path inside the container to a file or directory on the host system and lets it access the file or directory.
*/
public var mountPoints: List? = null
/**
* The set of ports that are available to bind to processes in the container. For example, a game server process requires a container port to allow game clients to connect to it. Container ports aren't directly accessed by inbound traffic. Amazon GameLift maps these container ports to externally accessible connection ports, which are assigned as needed from the container fleet's `ConnectionPortRange`.
*/
public var portConfiguration: aws.sdk.kotlin.services.gamelift.model.ContainerPortConfiguration? = null
/**
* A unique and immutable identifier for the container image. The digest is a SHA 256 hash of the container image manifest.
*/
public var resolvedImageDigest: kotlin.String? = null
/**
* The Amazon GameLift server SDK version that the game server is integrated with. Only game servers using 5.2.0 or higher are compatible with container fleets.
*/
public var serverSdkVersion: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.GameServerContainerDefinition) : this() {
this.containerName = x.containerName
this.dependsOn = x.dependsOn
this.environmentOverride = x.environmentOverride
this.imageUri = x.imageUri
this.mountPoints = x.mountPoints
this.portConfiguration = x.portConfiguration
this.resolvedImageDigest = x.resolvedImageDigest
this.serverSdkVersion = x.serverSdkVersion
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.GameServerContainerDefinition = GameServerContainerDefinition(this)
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.ContainerPortConfiguration] inside the given [block]
*/
public fun portConfiguration(block: aws.sdk.kotlin.services.gamelift.model.ContainerPortConfiguration.Builder.() -> kotlin.Unit) {
this.portConfiguration = aws.sdk.kotlin.services.gamelift.model.ContainerPortConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy