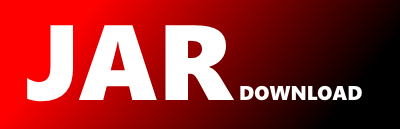
commonMain.aws.sdk.kotlin.services.gamelift.model.GameSession.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gamelift-jvm Show documentation
Show all versions of gamelift-jvm Show documentation
The AWS SDK for Kotlin client for GameLift
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Properties describing a game session.
*
* A game session in ACTIVE status can host players. When a game session ends, its status is set to `TERMINATED`.
*
* Amazon GameLift retains a game session resource for 30 days after the game session ends. You can reuse idempotency token values after this time. Game session logs are retained for 14 days.
*
* [All APIs by task](https://docs.aws.amazon.com/gamelift/latest/developerguide/reference-awssdk.html#reference-awssdk-resources-fleets)
*/
public class GameSession private constructor(builder: Builder) {
/**
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that limits the number of game sessions a player can create.
*/
public val creatorId: kotlin.String? = builder.creatorId
/**
* Number of players currently in the game session.
*/
public val currentPlayerSessionCount: kotlin.Int? = builder.currentPlayerSessionCount
/**
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
* + TLS-enabled fleets: `..amazongamelift.com`.
* + Non-TLS-enabled fleets: `ec2-.compute.amazonaws.com`. (See [Amazon EC2 Instance IP Addressing](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/using-instance-addressing.html#concepts-public-addresses).)
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP address.
*/
public val dnsName: kotlin.String? = builder.dnsName
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) associated with the GameLift fleet that this game session is running on.
*/
public val fleetArn: kotlin.String? = builder.fleetArn
/**
* A unique identifier for the fleet that the game session is running on.
*/
public val fleetId: kotlin.String? = builder.fleetId
/**
* A set of key-value pairs that can store custom data in a game session. For example: `{"Key": "difficulty", "Value": "novice"}`.
*/
public val gameProperties: List? = builder.gameProperties
/**
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server process with a request to start a new game session. For more information, see [Start a game session](https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-sdk-server-api.html#gamelift-sdk-server-startsession).
*/
public val gameSessionData: kotlin.String? = builder.gameSessionData
/**
* A unique identifier for the game session. A game session ARN has the following format: `arn:aws:gamelift:::gamesession//`.
*/
public val gameSessionId: kotlin.String? = builder.gameSessionId
/**
* The IP address of the game session. To connect to a Amazon GameLift game server, an app needs both the IP address and port number.
*/
public val ipAddress: kotlin.String? = builder.ipAddress
/**
* The fleet location where the game session is running. This value might specify the fleet's home Region or a remote location. Location is expressed as an Amazon Web Services Region code such as `us-west-2`.
*/
public val location: kotlin.String? = builder.location
/**
* Information about the matchmaking process that resulted in the game session, if matchmaking was used. Data is in JSON syntax, formatted as a string. Information includes the matchmaker ID as well as player attributes and team assignments. For more details on matchmaker data, see [Match Data](https://docs.aws.amazon.com/gamelift/latest/flexmatchguide/match-server.html#match-server-data). Matchmaker data is updated whenever new players are added during a successful backfill (see [StartMatchBackfill](https://docs.aws.amazon.com/gamelift/latest/apireference/API_StartMatchBackfill.html)).
*/
public val matchmakerData: kotlin.String? = builder.matchmakerData
/**
* The maximum number of players that can be connected simultaneously to the game session.
*/
public val maximumPlayerSessionCount: kotlin.Int? = builder.maximumPlayerSessionCount
/**
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*/
public val name: kotlin.String? = builder.name
/**
* Indicates whether or not the game session is accepting new players.
*/
public val playerSessionCreationPolicy: aws.sdk.kotlin.services.gamelift.model.PlayerSessionCreationPolicy? = builder.playerSessionCreationPolicy
/**
* The port number for the game session. To connect to a Amazon GameLift game server, an app needs both the IP address and port number.
*/
public val port: kotlin.Int? = builder.port
/**
* Current status of the game session. A game session must have an `ACTIVE` status to have player sessions.
*/
public val status: aws.sdk.kotlin.services.gamelift.model.GameSessionStatus? = builder.status
/**
* Provides additional information about game session status. `INTERRUPTED` indicates that the game session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*/
public val statusReason: aws.sdk.kotlin.services.gamelift.model.GameSessionStatusReason? = builder.statusReason
/**
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public val terminationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.terminationTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.GameSession = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GameSession(")
append("creationTime=$creationTime,")
append("creatorId=$creatorId,")
append("currentPlayerSessionCount=$currentPlayerSessionCount,")
append("dnsName=$dnsName,")
append("fleetArn=$fleetArn,")
append("fleetId=$fleetId,")
append("gameProperties=$gameProperties,")
append("gameSessionData=$gameSessionData,")
append("gameSessionId=$gameSessionId,")
append("ipAddress=*** Sensitive Data Redacted ***,")
append("location=$location,")
append("matchmakerData=$matchmakerData,")
append("maximumPlayerSessionCount=$maximumPlayerSessionCount,")
append("name=$name,")
append("playerSessionCreationPolicy=$playerSessionCreationPolicy,")
append("port=*** Sensitive Data Redacted ***,")
append("status=$status,")
append("statusReason=$statusReason,")
append("terminationTime=$terminationTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (creatorId?.hashCode() ?: 0)
result = 31 * result + (currentPlayerSessionCount ?: 0)
result = 31 * result + (dnsName?.hashCode() ?: 0)
result = 31 * result + (fleetArn?.hashCode() ?: 0)
result = 31 * result + (fleetId?.hashCode() ?: 0)
result = 31 * result + (gameProperties?.hashCode() ?: 0)
result = 31 * result + (gameSessionData?.hashCode() ?: 0)
result = 31 * result + (gameSessionId?.hashCode() ?: 0)
result = 31 * result + (ipAddress?.hashCode() ?: 0)
result = 31 * result + (location?.hashCode() ?: 0)
result = 31 * result + (matchmakerData?.hashCode() ?: 0)
result = 31 * result + (maximumPlayerSessionCount ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (playerSessionCreationPolicy?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (statusReason?.hashCode() ?: 0)
result = 31 * result + (terminationTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GameSession
if (creationTime != other.creationTime) return false
if (creatorId != other.creatorId) return false
if (currentPlayerSessionCount != other.currentPlayerSessionCount) return false
if (dnsName != other.dnsName) return false
if (fleetArn != other.fleetArn) return false
if (fleetId != other.fleetId) return false
if (gameProperties != other.gameProperties) return false
if (gameSessionData != other.gameSessionData) return false
if (gameSessionId != other.gameSessionId) return false
if (ipAddress != other.ipAddress) return false
if (location != other.location) return false
if (matchmakerData != other.matchmakerData) return false
if (maximumPlayerSessionCount != other.maximumPlayerSessionCount) return false
if (name != other.name) return false
if (playerSessionCreationPolicy != other.playerSessionCreationPolicy) return false
if (port != other.port) return false
if (status != other.status) return false
if (statusReason != other.statusReason) return false
if (terminationTime != other.terminationTime) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.GameSession = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A unique identifier for a player. This ID is used to enforce a resource protection policy (if one exists), that limits the number of game sessions a player can create.
*/
public var creatorId: kotlin.String? = null
/**
* Number of players currently in the game session.
*/
public var currentPlayerSessionCount: kotlin.Int? = null
/**
* The DNS identifier assigned to the instance that is running the game session. Values have the following format:
* + TLS-enabled fleets: `..amazongamelift.com`.
* + Non-TLS-enabled fleets: `ec2-.compute.amazonaws.com`. (See [Amazon EC2 Instance IP Addressing](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/using-instance-addressing.html#concepts-public-addresses).)
*
* When connecting to a game session that is running on a TLS-enabled fleet, you must use the DNS name, not the IP address.
*/
public var dnsName: kotlin.String? = null
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) associated with the GameLift fleet that this game session is running on.
*/
public var fleetArn: kotlin.String? = null
/**
* A unique identifier for the fleet that the game session is running on.
*/
public var fleetId: kotlin.String? = null
/**
* A set of key-value pairs that can store custom data in a game session. For example: `{"Key": "difficulty", "Value": "novice"}`.
*/
public var gameProperties: List? = null
/**
* A set of custom game session properties, formatted as a single string value. This data is passed to a game server process with a request to start a new game session. For more information, see [Start a game session](https://docs.aws.amazon.com/gamelift/latest/developerguide/gamelift-sdk-server-api.html#gamelift-sdk-server-startsession).
*/
public var gameSessionData: kotlin.String? = null
/**
* A unique identifier for the game session. A game session ARN has the following format: `arn:aws:gamelift:::gamesession//`.
*/
public var gameSessionId: kotlin.String? = null
/**
* The IP address of the game session. To connect to a Amazon GameLift game server, an app needs both the IP address and port number.
*/
public var ipAddress: kotlin.String? = null
/**
* The fleet location where the game session is running. This value might specify the fleet's home Region or a remote location. Location is expressed as an Amazon Web Services Region code such as `us-west-2`.
*/
public var location: kotlin.String? = null
/**
* Information about the matchmaking process that resulted in the game session, if matchmaking was used. Data is in JSON syntax, formatted as a string. Information includes the matchmaker ID as well as player attributes and team assignments. For more details on matchmaker data, see [Match Data](https://docs.aws.amazon.com/gamelift/latest/flexmatchguide/match-server.html#match-server-data). Matchmaker data is updated whenever new players are added during a successful backfill (see [StartMatchBackfill](https://docs.aws.amazon.com/gamelift/latest/apireference/API_StartMatchBackfill.html)).
*/
public var matchmakerData: kotlin.String? = null
/**
* The maximum number of players that can be connected simultaneously to the game session.
*/
public var maximumPlayerSessionCount: kotlin.Int? = null
/**
* A descriptive label that is associated with a game session. Session names do not need to be unique.
*/
public var name: kotlin.String? = null
/**
* Indicates whether or not the game session is accepting new players.
*/
public var playerSessionCreationPolicy: aws.sdk.kotlin.services.gamelift.model.PlayerSessionCreationPolicy? = null
/**
* The port number for the game session. To connect to a Amazon GameLift game server, an app needs both the IP address and port number.
*/
public var port: kotlin.Int? = null
/**
* Current status of the game session. A game session must have an `ACTIVE` status to have player sessions.
*/
public var status: aws.sdk.kotlin.services.gamelift.model.GameSessionStatus? = null
/**
* Provides additional information about game session status. `INTERRUPTED` indicates that the game session was hosted on a spot instance that was reclaimed, causing the active game session to be terminated.
*/
public var statusReason: aws.sdk.kotlin.services.gamelift.model.GameSessionStatusReason? = null
/**
* A time stamp indicating when this data object was terminated. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public var terminationTime: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.GameSession) : this() {
this.creationTime = x.creationTime
this.creatorId = x.creatorId
this.currentPlayerSessionCount = x.currentPlayerSessionCount
this.dnsName = x.dnsName
this.fleetArn = x.fleetArn
this.fleetId = x.fleetId
this.gameProperties = x.gameProperties
this.gameSessionData = x.gameSessionData
this.gameSessionId = x.gameSessionId
this.ipAddress = x.ipAddress
this.location = x.location
this.matchmakerData = x.matchmakerData
this.maximumPlayerSessionCount = x.maximumPlayerSessionCount
this.name = x.name
this.playerSessionCreationPolicy = x.playerSessionCreationPolicy
this.port = x.port
this.status = x.status
this.statusReason = x.statusReason
this.terminationTime = x.terminationTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.GameSession = GameSession(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy