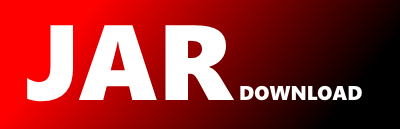
commonMain.aws.sdk.kotlin.services.gamelift.model.LogConfiguration.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A method for collecting container logs for the fleet. Amazon GameLift saves all standard output for each container in logs, including game session logs. You can select from the following methods:
*/
public class LogConfiguration private constructor(builder: Builder) {
/**
* The type of log collection to use for a fleet.
* + `CLOUDWATCH` -- (default value) Send logs to an Amazon CloudWatch log group that you define. Each container emits a log stream, which is organized in the log group.
* + `S3` -- Store logs in an Amazon S3 bucket that you define.
* + `NONE` -- Don't collect container logs.
*/
public val logDestination: aws.sdk.kotlin.services.gamelift.model.LogDestination? = builder.logDestination
/**
* If log destination is `CLOUDWATCH`, logs are sent to the specified log group in Amazon CloudWatch.
*/
public val logGroupArn: kotlin.String? = builder.logGroupArn
/**
* If log destination is `S3`, logs are sent to the specified Amazon S3 bucket name.
*/
public val s3BucketName: kotlin.String? = builder.s3BucketName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.LogConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("LogConfiguration(")
append("logDestination=$logDestination,")
append("logGroupArn=$logGroupArn,")
append("s3BucketName=$s3BucketName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = logDestination?.hashCode() ?: 0
result = 31 * result + (logGroupArn?.hashCode() ?: 0)
result = 31 * result + (s3BucketName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as LogConfiguration
if (logDestination != other.logDestination) return false
if (logGroupArn != other.logGroupArn) return false
if (s3BucketName != other.s3BucketName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.LogConfiguration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The type of log collection to use for a fleet.
* + `CLOUDWATCH` -- (default value) Send logs to an Amazon CloudWatch log group that you define. Each container emits a log stream, which is organized in the log group.
* + `S3` -- Store logs in an Amazon S3 bucket that you define.
* + `NONE` -- Don't collect container logs.
*/
public var logDestination: aws.sdk.kotlin.services.gamelift.model.LogDestination? = null
/**
* If log destination is `CLOUDWATCH`, logs are sent to the specified log group in Amazon CloudWatch.
*/
public var logGroupArn: kotlin.String? = null
/**
* If log destination is `S3`, logs are sent to the specified Amazon S3 bucket name.
*/
public var s3BucketName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.LogConfiguration) : this() {
this.logDestination = x.logDestination
this.logGroupArn = x.logGroupArn
this.s3BucketName = x.s3BucketName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.LogConfiguration = LogConfiguration(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy