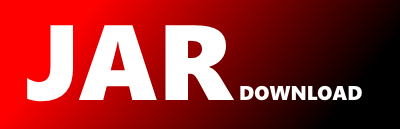
commonMain.aws.sdk.kotlin.services.gamelift.model.Script.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gamelift-jvm Show documentation
Show all versions of gamelift-jvm Show documentation
The AWS SDK for Kotlin client for GameLift
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Properties describing a Realtime script.
*
* **Related actions**
*
* [All APIs by task](https://docs.aws.amazon.com/gamelift/latest/developerguide/reference-awssdk.html#reference-awssdk-resources-fleets)
*/
public class Script private constructor(builder: Builder) {
/**
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* A descriptive label that is associated with a script. Script names do not need to be unique.
*/
public val name: kotlin.String? = builder.name
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) that is assigned to a Amazon GameLift script resource and uniquely identifies it. ARNs are unique across all Regions. In a GameLift script ARN, the resource ID matches the *ScriptId* value.
*/
public val scriptArn: kotlin.String? = builder.scriptArn
/**
* A unique identifier for the Realtime script
*/
public val scriptId: kotlin.String? = builder.scriptId
/**
* The file size of the uploaded Realtime script, expressed in bytes. When files are uploaded from an S3 location, this value remains at "0".
*/
public val sizeOnDisk: kotlin.Long? = builder.sizeOnDisk
/**
* The location of the Amazon S3 bucket where a zipped file containing your Realtime scripts is stored. The storage location must specify the Amazon S3 bucket name, the zip file name (the "key"), and a role ARN that allows Amazon GameLift to access the Amazon S3 storage location. The S3 bucket must be in the same Region where you want to create a new script. By default, Amazon GameLift uploads the latest version of the zip file; if you have S3 object versioning turned on, you can use the `ObjectVersion` parameter to specify an earlier version.
*/
public val storageLocation: aws.sdk.kotlin.services.gamelift.model.S3Location? = builder.storageLocation
/**
* Version information that is associated with a build or script. Version strings do not need to be unique.
*/
public val version: kotlin.String? = builder.version
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.Script = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Script(")
append("creationTime=$creationTime,")
append("name=$name,")
append("scriptArn=$scriptArn,")
append("scriptId=$scriptId,")
append("sizeOnDisk=$sizeOnDisk,")
append("storageLocation=$storageLocation,")
append("version=$version")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (scriptArn?.hashCode() ?: 0)
result = 31 * result + (scriptId?.hashCode() ?: 0)
result = 31 * result + (sizeOnDisk?.hashCode() ?: 0)
result = 31 * result + (storageLocation?.hashCode() ?: 0)
result = 31 * result + (version?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Script
if (creationTime != other.creationTime) return false
if (name != other.name) return false
if (scriptArn != other.scriptArn) return false
if (scriptId != other.scriptId) return false
if (sizeOnDisk != other.sizeOnDisk) return false
if (storageLocation != other.storageLocation) return false
if (version != other.version) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.Script = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A time stamp indicating when this data object was created. Format is a number expressed in Unix time as milliseconds (for example `"1469498468.057"`).
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A descriptive label that is associated with a script. Script names do not need to be unique.
*/
public var name: kotlin.String? = null
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) that is assigned to a Amazon GameLift script resource and uniquely identifies it. ARNs are unique across all Regions. In a GameLift script ARN, the resource ID matches the *ScriptId* value.
*/
public var scriptArn: kotlin.String? = null
/**
* A unique identifier for the Realtime script
*/
public var scriptId: kotlin.String? = null
/**
* The file size of the uploaded Realtime script, expressed in bytes. When files are uploaded from an S3 location, this value remains at "0".
*/
public var sizeOnDisk: kotlin.Long? = null
/**
* The location of the Amazon S3 bucket where a zipped file containing your Realtime scripts is stored. The storage location must specify the Amazon S3 bucket name, the zip file name (the "key"), and a role ARN that allows Amazon GameLift to access the Amazon S3 storage location. The S3 bucket must be in the same Region where you want to create a new script. By default, Amazon GameLift uploads the latest version of the zip file; if you have S3 object versioning turned on, you can use the `ObjectVersion` parameter to specify an earlier version.
*/
public var storageLocation: aws.sdk.kotlin.services.gamelift.model.S3Location? = null
/**
* Version information that is associated with a build or script. Version strings do not need to be unique.
*/
public var version: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.Script) : this() {
this.creationTime = x.creationTime
this.name = x.name
this.scriptArn = x.scriptArn
this.scriptId = x.scriptId
this.sizeOnDisk = x.sizeOnDisk
this.storageLocation = x.storageLocation
this.version = x.version
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.Script = Script(this)
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.S3Location] inside the given [block]
*/
public fun storageLocation(block: aws.sdk.kotlin.services.gamelift.model.S3Location.Builder.() -> kotlin.Unit) {
this.storageLocation = aws.sdk.kotlin.services.gamelift.model.S3Location.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy