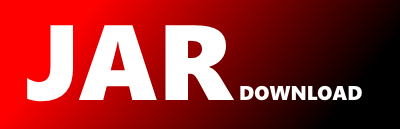
commonMain.aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnection.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.gamelift.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Represents a peering connection between a VPC on one of your Amazon Web Services accounts and the VPC for your Amazon GameLift fleets. This record may be for an active peering connection or a pending connection that has not yet been established.
*
* **Related actions**
*
* [All APIs by task](https://docs.aws.amazon.com/gamelift/latest/developerguide/reference-awssdk.html#reference-awssdk-resources-fleets)
*/
public class VpcPeeringConnection private constructor(builder: Builder) {
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) associated with the GameLift fleet resource for this connection.
*/
public val fleetArn: kotlin.String? = builder.fleetArn
/**
* A unique identifier for the fleet. This ID determines the ID of the Amazon GameLift VPC for your fleet.
*/
public val fleetId: kotlin.String? = builder.fleetId
/**
* A unique identifier for the VPC that contains the Amazon GameLift fleet for this connection. This VPC is managed by Amazon GameLift and does not appear in your Amazon Web Services account.
*/
public val gameLiftVpcId: kotlin.String? = builder.gameLiftVpcId
/**
* CIDR block of IPv4 addresses assigned to the VPC peering connection for the GameLift VPC. The peered VPC also has an IPv4 CIDR block associated with it; these blocks cannot overlap or the peering connection cannot be created.
*/
public val ipv4CidrBlock: kotlin.String? = builder.ipv4CidrBlock
/**
* A unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the same Region as your fleet. To look up a VPC ID, use the [VPC Dashboard](https://console.aws.amazon.com/vpc/) in the Amazon Web Services Management Console. Learn more about VPC peering in [VPC Peering with Amazon GameLift Fleets](https://docs.aws.amazon.com/gamelift/latest/developerguide/vpc-peering.html).
*/
public val peerVpcId: kotlin.String? = builder.peerVpcId
/**
* The status information about the connection. Status indicates if a connection is pending, successful, or failed.
*/
public val status: aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnectionStatus? = builder.status
/**
* A unique identifier that is automatically assigned to the connection record. This ID is referenced in VPC peering connection events, and is used when deleting a connection.
*/
public val vpcPeeringConnectionId: kotlin.String? = builder.vpcPeeringConnectionId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnection = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("VpcPeeringConnection(")
append("fleetArn=$fleetArn,")
append("fleetId=$fleetId,")
append("gameLiftVpcId=$gameLiftVpcId,")
append("ipv4CidrBlock=$ipv4CidrBlock,")
append("peerVpcId=$peerVpcId,")
append("status=$status,")
append("vpcPeeringConnectionId=$vpcPeeringConnectionId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fleetArn?.hashCode() ?: 0
result = 31 * result + (fleetId?.hashCode() ?: 0)
result = 31 * result + (gameLiftVpcId?.hashCode() ?: 0)
result = 31 * result + (ipv4CidrBlock?.hashCode() ?: 0)
result = 31 * result + (peerVpcId?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (vpcPeeringConnectionId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as VpcPeeringConnection
if (fleetArn != other.fleetArn) return false
if (fleetId != other.fleetId) return false
if (gameLiftVpcId != other.gameLiftVpcId) return false
if (ipv4CidrBlock != other.ipv4CidrBlock) return false
if (peerVpcId != other.peerVpcId) return false
if (status != other.status) return false
if (vpcPeeringConnectionId != other.vpcPeeringConnectionId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnection = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name ([ARN](https://docs.aws.amazon.com/AmazonS3/latest/dev/s3-arn-format.html)) associated with the GameLift fleet resource for this connection.
*/
public var fleetArn: kotlin.String? = null
/**
* A unique identifier for the fleet. This ID determines the ID of the Amazon GameLift VPC for your fleet.
*/
public var fleetId: kotlin.String? = null
/**
* A unique identifier for the VPC that contains the Amazon GameLift fleet for this connection. This VPC is managed by Amazon GameLift and does not appear in your Amazon Web Services account.
*/
public var gameLiftVpcId: kotlin.String? = null
/**
* CIDR block of IPv4 addresses assigned to the VPC peering connection for the GameLift VPC. The peered VPC also has an IPv4 CIDR block associated with it; these blocks cannot overlap or the peering connection cannot be created.
*/
public var ipv4CidrBlock: kotlin.String? = null
/**
* A unique identifier for a VPC with resources to be accessed by your Amazon GameLift fleet. The VPC must be in the same Region as your fleet. To look up a VPC ID, use the [VPC Dashboard](https://console.aws.amazon.com/vpc/) in the Amazon Web Services Management Console. Learn more about VPC peering in [VPC Peering with Amazon GameLift Fleets](https://docs.aws.amazon.com/gamelift/latest/developerguide/vpc-peering.html).
*/
public var peerVpcId: kotlin.String? = null
/**
* The status information about the connection. Status indicates if a connection is pending, successful, or failed.
*/
public var status: aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnectionStatus? = null
/**
* A unique identifier that is automatically assigned to the connection record. This ID is referenced in VPC peering connection events, and is used when deleting a connection.
*/
public var vpcPeeringConnectionId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnection) : this() {
this.fleetArn = x.fleetArn
this.fleetId = x.fleetId
this.gameLiftVpcId = x.gameLiftVpcId
this.ipv4CidrBlock = x.ipv4CidrBlock
this.peerVpcId = x.peerVpcId
this.status = x.status
this.vpcPeeringConnectionId = x.vpcPeeringConnectionId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnection = VpcPeeringConnection(this)
/**
* construct an [aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnectionStatus] inside the given [block]
*/
public fun status(block: aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnectionStatus.Builder.() -> kotlin.Unit) {
this.status = aws.sdk.kotlin.services.gamelift.model.VpcPeeringConnectionStatus.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy