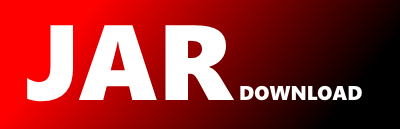
commonMain.aws.sdk.kotlin.services.glacier.model.Encryption.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
/**
* Contains information about the encryption used to store the job results in Amazon S3.
*/
public class Encryption private constructor(builder: Builder) {
/**
* The server-side encryption algorithm used when storing job results in Amazon S3, for example `AES256` or `aws:kms`.
*/
public val encryptionType: aws.sdk.kotlin.services.glacier.model.EncryptionType? = builder.encryptionType
/**
* Optional. If the encryption type is `aws:kms`, you can use this value to specify the encryption context for the job results.
*/
public val kmsContext: kotlin.String? = builder.kmsContext
/**
* The AWS KMS key ID to use for object encryption. All GET and PUT requests for an object protected by AWS KMS fail if not made by using Secure Sockets Layer (SSL) or Signature Version 4.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.Encryption = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Encryption(")
append("encryptionType=$encryptionType,")
append("kmsContext=$kmsContext,")
append("kmsKeyId=$kmsKeyId)")
}
override fun hashCode(): kotlin.Int {
var result = encryptionType?.hashCode() ?: 0
result = 31 * result + (kmsContext?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Encryption
if (encryptionType != other.encryptionType) return false
if (kmsContext != other.kmsContext) return false
if (kmsKeyId != other.kmsKeyId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.Encryption = Builder(this).apply(block).build()
public class Builder {
/**
* The server-side encryption algorithm used when storing job results in Amazon S3, for example `AES256` or `aws:kms`.
*/
public var encryptionType: aws.sdk.kotlin.services.glacier.model.EncryptionType? = null
/**
* Optional. If the encryption type is `aws:kms`, you can use this value to specify the encryption context for the job results.
*/
public var kmsContext: kotlin.String? = null
/**
* The AWS KMS key ID to use for object encryption. All GET and PUT requests for an object protected by AWS KMS fail if not made by using Secure Sockets Layer (SSL) or Signature Version 4.
*/
public var kmsKeyId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.Encryption) : this() {
this.encryptionType = x.encryptionType
this.kmsContext = x.kmsContext
this.kmsKeyId = x.kmsKeyId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.Encryption = Encryption(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy