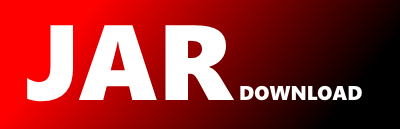
commonMain.aws.sdk.kotlin.services.glacier.model.CsvOutput.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
/**
* Contains information about the comma-separated value (CSV) file that the job results are stored in.
*/
public class CsvOutput private constructor(builder: Builder) {
/**
* A value used to separate individual fields from each other within a record.
*/
public val fieldDelimiter: kotlin.String? = builder.fieldDelimiter
/**
* A value used as an escape character where the field delimiter is part of the value.
*/
public val quoteCharacter: kotlin.String? = builder.quoteCharacter
/**
* A single character used for escaping the quotation-mark character inside an already escaped value.
*/
public val quoteEscapeCharacter: kotlin.String? = builder.quoteEscapeCharacter
/**
* A value that indicates whether all output fields should be contained within quotation marks.
*/
public val quoteFields: aws.sdk.kotlin.services.glacier.model.QuoteFields? = builder.quoteFields
/**
* A value used to separate individual records from each other.
*/
public val recordDelimiter: kotlin.String? = builder.recordDelimiter
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.CsvOutput = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CsvOutput(")
append("fieldDelimiter=$fieldDelimiter,")
append("quoteCharacter=$quoteCharacter,")
append("quoteEscapeCharacter=$quoteEscapeCharacter,")
append("quoteFields=$quoteFields,")
append("recordDelimiter=$recordDelimiter")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = fieldDelimiter?.hashCode() ?: 0
result = 31 * result + (quoteCharacter?.hashCode() ?: 0)
result = 31 * result + (quoteEscapeCharacter?.hashCode() ?: 0)
result = 31 * result + (quoteFields?.hashCode() ?: 0)
result = 31 * result + (recordDelimiter?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CsvOutput
if (fieldDelimiter != other.fieldDelimiter) return false
if (quoteCharacter != other.quoteCharacter) return false
if (quoteEscapeCharacter != other.quoteEscapeCharacter) return false
if (quoteFields != other.quoteFields) return false
if (recordDelimiter != other.recordDelimiter) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.CsvOutput = Builder(this).apply(block).build()
public class Builder {
/**
* A value used to separate individual fields from each other within a record.
*/
public var fieldDelimiter: kotlin.String? = null
/**
* A value used as an escape character where the field delimiter is part of the value.
*/
public var quoteCharacter: kotlin.String? = null
/**
* A single character used for escaping the quotation-mark character inside an already escaped value.
*/
public var quoteEscapeCharacter: kotlin.String? = null
/**
* A value that indicates whether all output fields should be contained within quotation marks.
*/
public var quoteFields: aws.sdk.kotlin.services.glacier.model.QuoteFields? = null
/**
* A value used to separate individual records from each other.
*/
public var recordDelimiter: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.CsvOutput) : this() {
this.fieldDelimiter = x.fieldDelimiter
this.quoteCharacter = x.quoteCharacter
this.quoteEscapeCharacter = x.quoteEscapeCharacter
this.quoteFields = x.quoteFields
this.recordDelimiter = x.recordDelimiter
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.CsvOutput = CsvOutput(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy