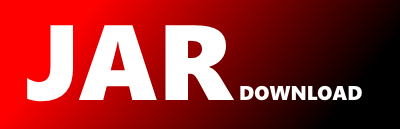
commonMain.aws.sdk.kotlin.services.glacier.model.Grant.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
/**
* Contains information about a grant.
*/
public class Grant private constructor(builder: Builder) {
/**
* The grantee.
*/
public val grantee: aws.sdk.kotlin.services.glacier.model.Grantee? = builder.grantee
/**
* Specifies the permission given to the grantee.
*/
public val permission: aws.sdk.kotlin.services.glacier.model.Permission? = builder.permission
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.Grant = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Grant(")
append("grantee=$grantee,")
append("permission=$permission")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = grantee?.hashCode() ?: 0
result = 31 * result + (permission?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Grant
if (grantee != other.grantee) return false
if (permission != other.permission) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.Grant = Builder(this).apply(block).build()
public class Builder {
/**
* The grantee.
*/
public var grantee: aws.sdk.kotlin.services.glacier.model.Grantee? = null
/**
* Specifies the permission given to the grantee.
*/
public var permission: aws.sdk.kotlin.services.glacier.model.Permission? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.Grant) : this() {
this.grantee = x.grantee
this.permission = x.permission
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.Grant = Grant(this)
/**
* construct an [aws.sdk.kotlin.services.glacier.model.Grantee] inside the given [block]
*/
public fun grantee(block: aws.sdk.kotlin.services.glacier.model.Grantee.Builder.() -> kotlin.Unit) {
this.grantee = aws.sdk.kotlin.services.glacier.model.Grantee.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy