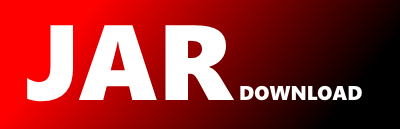
commonMain.aws.sdk.kotlin.services.glacier.model.DescribeJobResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains the description of an Amazon S3 Glacier job.
*/
public class DescribeJobResponse private constructor(builder: Builder) {
/**
* The job type. This value is either `ArchiveRetrieval`, `InventoryRetrieval`, or `Select`.
*/
public val action: aws.sdk.kotlin.services.glacier.model.ActionCode? = builder.action
/**
* The archive ID requested for a select job or archive retrieval. Otherwise, this field is null.
*/
public val archiveId: kotlin.String? = builder.archiveId
/**
* The SHA256 tree hash of the entire archive for an archive retrieval. For inventory retrieval or select jobs, this field is null.
*/
public val archiveSha256TreeHash: kotlin.String? = builder.archiveSha256TreeHash
/**
* For an archive retrieval job, this value is the size in bytes of the archive being requested for download. For an inventory retrieval or select job, this value is null.
*/
public val archiveSizeInBytes: kotlin.Long? = builder.archiveSizeInBytes
/**
* The job status. When a job is completed, you get the job's output using Get Job Output (GET output).
*/
public val completed: kotlin.Boolean = builder.completed
/**
* The UTC time that the job request completed. While the job is in progress, the value is null.
*/
public val completionDate: kotlin.String? = builder.completionDate
/**
* The UTC date when the job was created. This value is a string representation of ISO 8601 date format, for example `"2012-03-20T17:03:43.221Z"`.
*/
public val creationDate: kotlin.String? = builder.creationDate
/**
* Parameters used for range inventory retrieval.
*/
public val inventoryRetrievalParameters: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobDescription? = builder.inventoryRetrievalParameters
/**
* For an inventory retrieval job, this value is the size in bytes of the inventory requested for download. For an archive retrieval or select job, this value is null.
*/
public val inventorySizeInBytes: kotlin.Long? = builder.inventorySizeInBytes
/**
* The job description provided when initiating the job.
*/
public val jobDescription: kotlin.String? = builder.jobDescription
/**
* An opaque string that identifies an Amazon S3 Glacier job.
*/
public val jobId: kotlin.String? = builder.jobId
/**
* Contains the job output location.
*/
public val jobOutputPath: kotlin.String? = builder.jobOutputPath
/**
* Contains the location where the data from the select job is stored.
*/
public val outputLocation: aws.sdk.kotlin.services.glacier.model.OutputLocation? = builder.outputLocation
/**
* The retrieved byte range for archive retrieval jobs in the form *StartByteValue*-*EndByteValue*. If no range was specified in the archive retrieval, then the whole archive is retrieved. In this case, *StartByteValue* equals 0 and *EndByteValue* equals the size of the archive minus 1. For inventory retrieval or select jobs, this field is null.
*/
public val retrievalByteRange: kotlin.String? = builder.retrievalByteRange
/**
* Contains the parameters used for a select.
*/
public val selectParameters: aws.sdk.kotlin.services.glacier.model.SelectParameters? = builder.selectParameters
/**
* For an archive retrieval job, this value is the checksum of the archive. Otherwise, this value is null.
*
* The SHA256 tree hash value for the requested range of an archive. If the **InitiateJob** request for an archive specified a tree-hash aligned range, then this field returns a value.
*
* If the whole archive is retrieved, this value is the same as the ArchiveSHA256TreeHash value.
*
* This field is null for the following:
* + Archive retrieval jobs that specify a range that is not tree-hash aligned
*
* + Archival jobs that specify a range that is equal to the whole archive, when the job status is `InProgress`
*
* + Inventory jobs
* + Select jobs
*/
public val sha256TreeHash: kotlin.String? = builder.sha256TreeHash
/**
* An Amazon SNS topic that receives notification.
*/
public val snsTopic: kotlin.String? = builder.snsTopic
/**
* The status code can be `InProgress`, `Succeeded`, or `Failed`, and indicates the status of the job.
*/
public val statusCode: aws.sdk.kotlin.services.glacier.model.StatusCode? = builder.statusCode
/**
* A friendly message that describes the job status.
*/
public val statusMessage: kotlin.String? = builder.statusMessage
/**
* The tier to use for a select or an archive retrieval. Valid values are `Expedited`, `Standard`, or `Bulk`. `Standard` is the default.
*/
public val tier: kotlin.String? = builder.tier
/**
* The Amazon Resource Name (ARN) of the vault from which an archive retrieval was requested.
*/
public val vaultArn: kotlin.String? = builder.vaultArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.DescribeJobResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeJobResponse(")
append("action=$action,")
append("archiveId=$archiveId,")
append("archiveSha256TreeHash=$archiveSha256TreeHash,")
append("archiveSizeInBytes=$archiveSizeInBytes,")
append("completed=$completed,")
append("completionDate=$completionDate,")
append("creationDate=$creationDate,")
append("inventoryRetrievalParameters=$inventoryRetrievalParameters,")
append("inventorySizeInBytes=$inventorySizeInBytes,")
append("jobDescription=$jobDescription,")
append("jobId=$jobId,")
append("jobOutputPath=$jobOutputPath,")
append("outputLocation=$outputLocation,")
append("retrievalByteRange=$retrievalByteRange,")
append("selectParameters=$selectParameters,")
append("sha256TreeHash=$sha256TreeHash,")
append("snsTopic=$snsTopic,")
append("statusCode=$statusCode,")
append("statusMessage=$statusMessage,")
append("tier=$tier,")
append("vaultArn=$vaultArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = action?.hashCode() ?: 0
result = 31 * result + (archiveId?.hashCode() ?: 0)
result = 31 * result + (archiveSha256TreeHash?.hashCode() ?: 0)
result = 31 * result + (archiveSizeInBytes?.hashCode() ?: 0)
result = 31 * result + (completed.hashCode())
result = 31 * result + (completionDate?.hashCode() ?: 0)
result = 31 * result + (creationDate?.hashCode() ?: 0)
result = 31 * result + (inventoryRetrievalParameters?.hashCode() ?: 0)
result = 31 * result + (inventorySizeInBytes?.hashCode() ?: 0)
result = 31 * result + (jobDescription?.hashCode() ?: 0)
result = 31 * result + (jobId?.hashCode() ?: 0)
result = 31 * result + (jobOutputPath?.hashCode() ?: 0)
result = 31 * result + (outputLocation?.hashCode() ?: 0)
result = 31 * result + (retrievalByteRange?.hashCode() ?: 0)
result = 31 * result + (selectParameters?.hashCode() ?: 0)
result = 31 * result + (sha256TreeHash?.hashCode() ?: 0)
result = 31 * result + (snsTopic?.hashCode() ?: 0)
result = 31 * result + (statusCode?.hashCode() ?: 0)
result = 31 * result + (statusMessage?.hashCode() ?: 0)
result = 31 * result + (tier?.hashCode() ?: 0)
result = 31 * result + (vaultArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeJobResponse
if (action != other.action) return false
if (archiveId != other.archiveId) return false
if (archiveSha256TreeHash != other.archiveSha256TreeHash) return false
if (archiveSizeInBytes != other.archiveSizeInBytes) return false
if (completed != other.completed) return false
if (completionDate != other.completionDate) return false
if (creationDate != other.creationDate) return false
if (inventoryRetrievalParameters != other.inventoryRetrievalParameters) return false
if (inventorySizeInBytes != other.inventorySizeInBytes) return false
if (jobDescription != other.jobDescription) return false
if (jobId != other.jobId) return false
if (jobOutputPath != other.jobOutputPath) return false
if (outputLocation != other.outputLocation) return false
if (retrievalByteRange != other.retrievalByteRange) return false
if (selectParameters != other.selectParameters) return false
if (sha256TreeHash != other.sha256TreeHash) return false
if (snsTopic != other.snsTopic) return false
if (statusCode != other.statusCode) return false
if (statusMessage != other.statusMessage) return false
if (tier != other.tier) return false
if (vaultArn != other.vaultArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.DescribeJobResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The job type. This value is either `ArchiveRetrieval`, `InventoryRetrieval`, or `Select`.
*/
public var action: aws.sdk.kotlin.services.glacier.model.ActionCode? = null
/**
* The archive ID requested for a select job or archive retrieval. Otherwise, this field is null.
*/
public var archiveId: kotlin.String? = null
/**
* The SHA256 tree hash of the entire archive for an archive retrieval. For inventory retrieval or select jobs, this field is null.
*/
public var archiveSha256TreeHash: kotlin.String? = null
/**
* For an archive retrieval job, this value is the size in bytes of the archive being requested for download. For an inventory retrieval or select job, this value is null.
*/
public var archiveSizeInBytes: kotlin.Long? = null
/**
* The job status. When a job is completed, you get the job's output using Get Job Output (GET output).
*/
public var completed: kotlin.Boolean = false
/**
* The UTC time that the job request completed. While the job is in progress, the value is null.
*/
public var completionDate: kotlin.String? = null
/**
* The UTC date when the job was created. This value is a string representation of ISO 8601 date format, for example `"2012-03-20T17:03:43.221Z"`.
*/
public var creationDate: kotlin.String? = null
/**
* Parameters used for range inventory retrieval.
*/
public var inventoryRetrievalParameters: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobDescription? = null
/**
* For an inventory retrieval job, this value is the size in bytes of the inventory requested for download. For an archive retrieval or select job, this value is null.
*/
public var inventorySizeInBytes: kotlin.Long? = null
/**
* The job description provided when initiating the job.
*/
public var jobDescription: kotlin.String? = null
/**
* An opaque string that identifies an Amazon S3 Glacier job.
*/
public var jobId: kotlin.String? = null
/**
* Contains the job output location.
*/
public var jobOutputPath: kotlin.String? = null
/**
* Contains the location where the data from the select job is stored.
*/
public var outputLocation: aws.sdk.kotlin.services.glacier.model.OutputLocation? = null
/**
* The retrieved byte range for archive retrieval jobs in the form *StartByteValue*-*EndByteValue*. If no range was specified in the archive retrieval, then the whole archive is retrieved. In this case, *StartByteValue* equals 0 and *EndByteValue* equals the size of the archive minus 1. For inventory retrieval or select jobs, this field is null.
*/
public var retrievalByteRange: kotlin.String? = null
/**
* Contains the parameters used for a select.
*/
public var selectParameters: aws.sdk.kotlin.services.glacier.model.SelectParameters? = null
/**
* For an archive retrieval job, this value is the checksum of the archive. Otherwise, this value is null.
*
* The SHA256 tree hash value for the requested range of an archive. If the **InitiateJob** request for an archive specified a tree-hash aligned range, then this field returns a value.
*
* If the whole archive is retrieved, this value is the same as the ArchiveSHA256TreeHash value.
*
* This field is null for the following:
* + Archive retrieval jobs that specify a range that is not tree-hash aligned
*
* + Archival jobs that specify a range that is equal to the whole archive, when the job status is `InProgress`
*
* + Inventory jobs
* + Select jobs
*/
public var sha256TreeHash: kotlin.String? = null
/**
* An Amazon SNS topic that receives notification.
*/
public var snsTopic: kotlin.String? = null
/**
* The status code can be `InProgress`, `Succeeded`, or `Failed`, and indicates the status of the job.
*/
public var statusCode: aws.sdk.kotlin.services.glacier.model.StatusCode? = null
/**
* A friendly message that describes the job status.
*/
public var statusMessage: kotlin.String? = null
/**
* The tier to use for a select or an archive retrieval. Valid values are `Expedited`, `Standard`, or `Bulk`. `Standard` is the default.
*/
public var tier: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the vault from which an archive retrieval was requested.
*/
public var vaultArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.DescribeJobResponse) : this() {
this.action = x.action
this.archiveId = x.archiveId
this.archiveSha256TreeHash = x.archiveSha256TreeHash
this.archiveSizeInBytes = x.archiveSizeInBytes
this.completed = x.completed
this.completionDate = x.completionDate
this.creationDate = x.creationDate
this.inventoryRetrievalParameters = x.inventoryRetrievalParameters
this.inventorySizeInBytes = x.inventorySizeInBytes
this.jobDescription = x.jobDescription
this.jobId = x.jobId
this.jobOutputPath = x.jobOutputPath
this.outputLocation = x.outputLocation
this.retrievalByteRange = x.retrievalByteRange
this.selectParameters = x.selectParameters
this.sha256TreeHash = x.sha256TreeHash
this.snsTopic = x.snsTopic
this.statusCode = x.statusCode
this.statusMessage = x.statusMessage
this.tier = x.tier
this.vaultArn = x.vaultArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.DescribeJobResponse = DescribeJobResponse(this)
/**
* construct an [aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobDescription] inside the given [block]
*/
public fun inventoryRetrievalParameters(block: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobDescription.Builder.() -> kotlin.Unit) {
this.inventoryRetrievalParameters = aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobDescription.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.glacier.model.OutputLocation] inside the given [block]
*/
public fun outputLocation(block: aws.sdk.kotlin.services.glacier.model.OutputLocation.Builder.() -> kotlin.Unit) {
this.outputLocation = aws.sdk.kotlin.services.glacier.model.OutputLocation.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.glacier.model.SelectParameters] inside the given [block]
*/
public fun selectParameters(block: aws.sdk.kotlin.services.glacier.model.SelectParameters.Builder.() -> kotlin.Unit) {
this.selectParameters = aws.sdk.kotlin.services.glacier.model.SelectParameters.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy