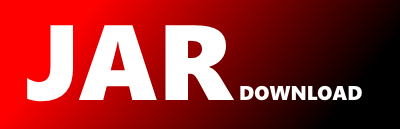
commonMain.aws.sdk.kotlin.services.glacier.model.JobParameters.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides options for defining a job.
*/
public class JobParameters private constructor(builder: Builder) {
/**
* The ID of the archive that you want to retrieve. This field is required only if `Type` is set to `select` or `archive-retrieval`code>. An error occurs if you specify this request parameter for an inventory retrieval job request.
*/
public val archiveId: kotlin.String? = builder.archiveId
/**
* The optional description for the job. The description must be less than or equal to 1,024 bytes. The allowable characters are 7-bit ASCII without control codes-specifically, ASCII values 32-126 decimal or 0x20-0x7E hexadecimal.
*/
public val description: kotlin.String? = builder.description
/**
* When initiating a job to retrieve a vault inventory, you can optionally add this parameter to your request to specify the output format. If you are initiating an inventory job and do not specify a Format field, JSON is the default format. Valid values are "CSV" and "JSON".
*/
public val format: kotlin.String? = builder.format
/**
* Input parameters used for range inventory retrieval.
*/
public val inventoryRetrievalParameters: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobInput? = builder.inventoryRetrievalParameters
/**
* Contains information about the location where the select job results are stored.
*/
public val outputLocation: aws.sdk.kotlin.services.glacier.model.OutputLocation? = builder.outputLocation
/**
* The byte range to retrieve for an archive retrieval. in the form "*StartByteValue*-*EndByteValue*" If not specified, the whole archive is retrieved. If specified, the byte range must be megabyte (1024*1024) aligned which means that *StartByteValue* must be divisible by 1 MB and *EndByteValue* plus 1 must be divisible by 1 MB or be the end of the archive specified as the archive byte size value minus 1. If RetrievalByteRange is not megabyte aligned, this operation returns a 400 response.
*
* An error occurs if you specify this field for an inventory retrieval job request.
*/
public val retrievalByteRange: kotlin.String? = builder.retrievalByteRange
/**
* Contains the parameters that define a job.
*/
public val selectParameters: aws.sdk.kotlin.services.glacier.model.SelectParameters? = builder.selectParameters
/**
* The Amazon SNS topic ARN to which Amazon S3 Glacier sends a notification when the job is completed and the output is ready for you to download. The specified topic publishes the notification to its subscribers. The SNS topic must exist.
*/
public val snsTopic: kotlin.String? = builder.snsTopic
/**
* The tier to use for a select or an archive retrieval job. Valid values are `Expedited`, `Standard`, or `Bulk`. `Standard` is the default.
*/
public val tier: kotlin.String? = builder.tier
/**
* The job type. You can initiate a job to perform a select query on an archive, retrieve an archive, or get an inventory of a vault. Valid values are "select", "archive-retrieval" and "inventory-retrieval".
*/
public val type: kotlin.String? = builder.type
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.JobParameters = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("JobParameters(")
append("archiveId=$archiveId,")
append("description=$description,")
append("format=$format,")
append("inventoryRetrievalParameters=$inventoryRetrievalParameters,")
append("outputLocation=$outputLocation,")
append("retrievalByteRange=$retrievalByteRange,")
append("selectParameters=$selectParameters,")
append("snsTopic=$snsTopic,")
append("tier=$tier,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = archiveId?.hashCode() ?: 0
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (format?.hashCode() ?: 0)
result = 31 * result + (inventoryRetrievalParameters?.hashCode() ?: 0)
result = 31 * result + (outputLocation?.hashCode() ?: 0)
result = 31 * result + (retrievalByteRange?.hashCode() ?: 0)
result = 31 * result + (selectParameters?.hashCode() ?: 0)
result = 31 * result + (snsTopic?.hashCode() ?: 0)
result = 31 * result + (tier?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as JobParameters
if (archiveId != other.archiveId) return false
if (description != other.description) return false
if (format != other.format) return false
if (inventoryRetrievalParameters != other.inventoryRetrievalParameters) return false
if (outputLocation != other.outputLocation) return false
if (retrievalByteRange != other.retrievalByteRange) return false
if (selectParameters != other.selectParameters) return false
if (snsTopic != other.snsTopic) return false
if (tier != other.tier) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.JobParameters = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the archive that you want to retrieve. This field is required only if `Type` is set to `select` or `archive-retrieval`code>. An error occurs if you specify this request parameter for an inventory retrieval job request.
*/
public var archiveId: kotlin.String? = null
/**
* The optional description for the job. The description must be less than or equal to 1,024 bytes. The allowable characters are 7-bit ASCII without control codes-specifically, ASCII values 32-126 decimal or 0x20-0x7E hexadecimal.
*/
public var description: kotlin.String? = null
/**
* When initiating a job to retrieve a vault inventory, you can optionally add this parameter to your request to specify the output format. If you are initiating an inventory job and do not specify a Format field, JSON is the default format. Valid values are "CSV" and "JSON".
*/
public var format: kotlin.String? = null
/**
* Input parameters used for range inventory retrieval.
*/
public var inventoryRetrievalParameters: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobInput? = null
/**
* Contains information about the location where the select job results are stored.
*/
public var outputLocation: aws.sdk.kotlin.services.glacier.model.OutputLocation? = null
/**
* The byte range to retrieve for an archive retrieval. in the form "*StartByteValue*-*EndByteValue*" If not specified, the whole archive is retrieved. If specified, the byte range must be megabyte (1024*1024) aligned which means that *StartByteValue* must be divisible by 1 MB and *EndByteValue* plus 1 must be divisible by 1 MB or be the end of the archive specified as the archive byte size value minus 1. If RetrievalByteRange is not megabyte aligned, this operation returns a 400 response.
*
* An error occurs if you specify this field for an inventory retrieval job request.
*/
public var retrievalByteRange: kotlin.String? = null
/**
* Contains the parameters that define a job.
*/
public var selectParameters: aws.sdk.kotlin.services.glacier.model.SelectParameters? = null
/**
* The Amazon SNS topic ARN to which Amazon S3 Glacier sends a notification when the job is completed and the output is ready for you to download. The specified topic publishes the notification to its subscribers. The SNS topic must exist.
*/
public var snsTopic: kotlin.String? = null
/**
* The tier to use for a select or an archive retrieval job. Valid values are `Expedited`, `Standard`, or `Bulk`. `Standard` is the default.
*/
public var tier: kotlin.String? = null
/**
* The job type. You can initiate a job to perform a select query on an archive, retrieve an archive, or get an inventory of a vault. Valid values are "select", "archive-retrieval" and "inventory-retrieval".
*/
public var type: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.JobParameters) : this() {
this.archiveId = x.archiveId
this.description = x.description
this.format = x.format
this.inventoryRetrievalParameters = x.inventoryRetrievalParameters
this.outputLocation = x.outputLocation
this.retrievalByteRange = x.retrievalByteRange
this.selectParameters = x.selectParameters
this.snsTopic = x.snsTopic
this.tier = x.tier
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.JobParameters = JobParameters(this)
/**
* construct an [aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobInput] inside the given [block]
*/
public fun inventoryRetrievalParameters(block: aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobInput.Builder.() -> kotlin.Unit) {
this.inventoryRetrievalParameters = aws.sdk.kotlin.services.glacier.model.InventoryRetrievalJobInput.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.glacier.model.OutputLocation] inside the given [block]
*/
public fun outputLocation(block: aws.sdk.kotlin.services.glacier.model.OutputLocation.Builder.() -> kotlin.Unit) {
this.outputLocation = aws.sdk.kotlin.services.glacier.model.OutputLocation.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.glacier.model.SelectParameters] inside the given [block]
*/
public fun selectParameters(block: aws.sdk.kotlin.services.glacier.model.SelectParameters.Builder.() -> kotlin.Unit) {
this.selectParameters = aws.sdk.kotlin.services.glacier.model.SelectParameters.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy