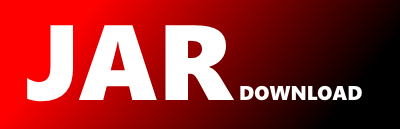
commonMain.aws.sdk.kotlin.services.glacier.model.DescribeVaultResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glacier-jvm Show documentation
Show all versions of glacier-jvm Show documentation
The AWS Kotlin client for Glacier
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Contains the Amazon S3 Glacier response to your request.
*/
public class DescribeVaultResponse private constructor(builder: Builder) {
/**
* The Universal Coordinated Time (UTC) date when the vault was created. This value should be a string in the ISO 8601 date format, for example `2012-03-20T17:03:43.221Z`.
*/
public val creationDate: kotlin.String? = builder.creationDate
/**
* The Universal Coordinated Time (UTC) date when Amazon S3 Glacier completed the last vault inventory. This value should be a string in the ISO 8601 date format, for example `2012-03-20T17:03:43.221Z`.
*/
public val lastInventoryDate: kotlin.String? = builder.lastInventoryDate
/**
* The number of archives in the vault as of the last inventory date. This field will return `null` if an inventory has not yet run on the vault, for example if you just created the vault.
*/
public val numberOfArchives: kotlin.Long = builder.numberOfArchives
/**
* Total size, in bytes, of the archives in the vault as of the last inventory date. This field will return null if an inventory has not yet run on the vault, for example if you just created the vault.
*/
public val sizeInBytes: kotlin.Long = builder.sizeInBytes
/**
* The Amazon Resource Name (ARN) of the vault.
*/
public val vaultArn: kotlin.String? = builder.vaultArn
/**
* The name of the vault.
*/
public val vaultName: kotlin.String? = builder.vaultName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.DescribeVaultResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeVaultResponse(")
append("creationDate=$creationDate,")
append("lastInventoryDate=$lastInventoryDate,")
append("numberOfArchives=$numberOfArchives,")
append("sizeInBytes=$sizeInBytes,")
append("vaultArn=$vaultArn,")
append("vaultName=$vaultName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = creationDate?.hashCode() ?: 0
result = 31 * result + (lastInventoryDate?.hashCode() ?: 0)
result = 31 * result + (numberOfArchives.hashCode())
result = 31 * result + (sizeInBytes.hashCode())
result = 31 * result + (vaultArn?.hashCode() ?: 0)
result = 31 * result + (vaultName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeVaultResponse
if (creationDate != other.creationDate) return false
if (lastInventoryDate != other.lastInventoryDate) return false
if (numberOfArchives != other.numberOfArchives) return false
if (sizeInBytes != other.sizeInBytes) return false
if (vaultArn != other.vaultArn) return false
if (vaultName != other.vaultName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.DescribeVaultResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Universal Coordinated Time (UTC) date when the vault was created. This value should be a string in the ISO 8601 date format, for example `2012-03-20T17:03:43.221Z`.
*/
public var creationDate: kotlin.String? = null
/**
* The Universal Coordinated Time (UTC) date when Amazon S3 Glacier completed the last vault inventory. This value should be a string in the ISO 8601 date format, for example `2012-03-20T17:03:43.221Z`.
*/
public var lastInventoryDate: kotlin.String? = null
/**
* The number of archives in the vault as of the last inventory date. This field will return `null` if an inventory has not yet run on the vault, for example if you just created the vault.
*/
public var numberOfArchives: kotlin.Long = 0L
/**
* Total size, in bytes, of the archives in the vault as of the last inventory date. This field will return null if an inventory has not yet run on the vault, for example if you just created the vault.
*/
public var sizeInBytes: kotlin.Long = 0L
/**
* The Amazon Resource Name (ARN) of the vault.
*/
public var vaultArn: kotlin.String? = null
/**
* The name of the vault.
*/
public var vaultName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.DescribeVaultResponse) : this() {
this.creationDate = x.creationDate
this.lastInventoryDate = x.lastInventoryDate
this.numberOfArchives = x.numberOfArchives
this.sizeInBytes = x.sizeInBytes
this.vaultArn = x.vaultArn
this.vaultName = x.vaultName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.DescribeVaultResponse = DescribeVaultResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy