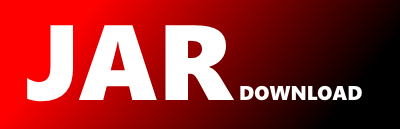
commonMain.aws.sdk.kotlin.services.glacier.model.SetVaultNotificationsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glacier.model
/**
* Provides options to configure notifications that will be sent when specific events happen to a vault.
*/
public class SetVaultNotificationsRequest private constructor(builder: Builder) {
/**
* The `AccountId` value is the AWS account ID of the account that owns the vault. You can either specify an AWS account ID or optionally a single '`-`' (hyphen), in which case Amazon S3 Glacier uses the AWS account ID associated with the credentials used to sign the request. If you use an account ID, do not include any hyphens ('-') in the ID.
*/
public val accountId: kotlin.String? = requireNotNull(builder.accountId) { "A non-null value must be provided for accountId" }
/**
* The name of the vault.
*/
public val vaultName: kotlin.String? = requireNotNull(builder.vaultName) { "A non-null value must be provided for vaultName" }
/**
* Provides options for specifying notification configuration.
*/
public val vaultNotificationConfig: aws.sdk.kotlin.services.glacier.model.VaultNotificationConfig? = builder.vaultNotificationConfig
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glacier.model.SetVaultNotificationsRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SetVaultNotificationsRequest(")
append("accountId=$accountId,")
append("vaultName=$vaultName,")
append("vaultNotificationConfig=$vaultNotificationConfig)")
}
override fun hashCode(): kotlin.Int {
var result = accountId?.hashCode() ?: 0
result = 31 * result + (vaultName?.hashCode() ?: 0)
result = 31 * result + (vaultNotificationConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SetVaultNotificationsRequest
if (accountId != other.accountId) return false
if (vaultName != other.vaultName) return false
if (vaultNotificationConfig != other.vaultNotificationConfig) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glacier.model.SetVaultNotificationsRequest = Builder(this).apply(block).build()
public class Builder {
/**
* The `AccountId` value is the AWS account ID of the account that owns the vault. You can either specify an AWS account ID or optionally a single '`-`' (hyphen), in which case Amazon S3 Glacier uses the AWS account ID associated with the credentials used to sign the request. If you use an account ID, do not include any hyphens ('-') in the ID.
*/
public var accountId: kotlin.String? = null
/**
* The name of the vault.
*/
public var vaultName: kotlin.String? = null
/**
* Provides options for specifying notification configuration.
*/
public var vaultNotificationConfig: aws.sdk.kotlin.services.glacier.model.VaultNotificationConfig? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glacier.model.SetVaultNotificationsRequest) : this() {
this.accountId = x.accountId
this.vaultName = x.vaultName
this.vaultNotificationConfig = x.vaultNotificationConfig
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glacier.model.SetVaultNotificationsRequest = SetVaultNotificationsRequest(this)
/**
* construct an [aws.sdk.kotlin.services.glacier.model.VaultNotificationConfig] inside the given [block]
*/
public fun vaultNotificationConfig(block: aws.sdk.kotlin.services.glacier.model.VaultNotificationConfig.Builder.() -> kotlin.Unit) {
this.vaultNotificationConfig = aws.sdk.kotlin.services.glacier.model.VaultNotificationConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy