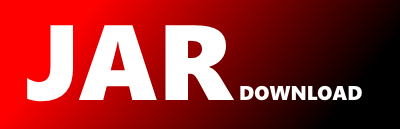
commonMain.aws.sdk.kotlin.services.glue.model.ConnectionInput.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glue-jvm Show documentation
Show all versions of glue-jvm Show documentation
The AWS SDK for Kotlin client for Glue
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.glue.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A structure that is used to specify a connection to create or update.
*/
public class ConnectionInput private constructor(builder: Builder) {
/**
* The authentication properties of the connection. Used for a Salesforce connection.
*/
public val authenticationConfiguration: aws.sdk.kotlin.services.glue.model.AuthenticationConfigurationInput? = builder.authenticationConfiguration
/**
* These key-value pairs define parameters for the connection.
*/
public val connectionProperties: Map = requireNotNull(builder.connectionProperties) { "A non-null value must be provided for connectionProperties" }
/**
* The type of the connection. Currently, these types are supported:
* + `JDBC` - Designates a connection to a database through Java Database Connectivity (JDBC).`JDBC` Connections use the following ConnectionParameters.
* + Required: All of (`HOST`, `PORT`, `JDBC_ENGINE`) or `JDBC_CONNECTION_URL`.
* + Required: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + Optional: `JDBC_ENFORCE_SSL`, `CUSTOM_JDBC_CERT`, `CUSTOM_JDBC_CERT_STRING`, `SKIP_CUSTOM_JDBC_CERT_VALIDATION`. These parameters are used to configure SSL with JDBC.
* + `KAFKA` - Designates a connection to an Apache Kafka streaming platform.`KAFKA` Connections use the following ConnectionParameters.
* + Required: `KAFKA_BOOTSTRAP_SERVERS`.
* + Optional: `KAFKA_SSL_ENABLED`, `KAFKA_CUSTOM_CERT`, `KAFKA_SKIP_CUSTOM_CERT_VALIDATION`. These parameters are used to configure SSL with `KAFKA`.
* + Optional: `KAFKA_CLIENT_KEYSTORE`, `KAFKA_CLIENT_KEYSTORE_PASSWORD`, `KAFKA_CLIENT_KEY_PASSWORD`, `ENCRYPTED_KAFKA_CLIENT_KEYSTORE_PASSWORD`, `ENCRYPTED_KAFKA_CLIENT_KEY_PASSWORD`. These parameters are used to configure TLS client configuration with SSL in `KAFKA`.
* + Optional: `KAFKA_SASL_MECHANISM`. Can be specified as `SCRAM-SHA-512`, `GSSAPI`, or `AWS_MSK_IAM`.
* + Optional: `KAFKA_SASL_SCRAM_USERNAME`, `KAFKA_SASL_SCRAM_PASSWORD`, `ENCRYPTED_KAFKA_SASL_SCRAM_PASSWORD`. These parameters are used to configure SASL/SCRAM-SHA-512 authentication with `KAFKA`.
* + Optional: `KAFKA_SASL_GSSAPI_KEYTAB`, `KAFKA_SASL_GSSAPI_KRB5_CONF`, `KAFKA_SASL_GSSAPI_SERVICE`, `KAFKA_SASL_GSSAPI_PRINCIPAL`. These parameters are used to configure SASL/GSSAPI authentication with `KAFKA`.
* + `MONGODB` - Designates a connection to a MongoDB document database.`MONGODB` Connections use the following ConnectionParameters.
* + Required: `CONNECTION_URL`.
* + Required: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + `SALESFORCE` - Designates a connection to Salesforce using OAuth authencation.
* + Requires the `AuthenticationConfiguration` member to be configured.
* + `VIEW_VALIDATION_REDSHIFT` - Designates a connection used for view validation by Amazon Redshift.
* + `VIEW_VALIDATION_ATHENA` - Designates a connection used for view validation by Amazon Athena.
* + `NETWORK` - Designates a network connection to a data source within an Amazon Virtual Private Cloud environment (Amazon VPC).`NETWORK` Connections do not require ConnectionParameters. Instead, provide a PhysicalConnectionRequirements.
* + `MARKETPLACE` - Uses configuration settings contained in a connector purchased from Amazon Web Services Marketplace to read from and write to data stores that are not natively supported by Glue.`MARKETPLACE` Connections use the following ConnectionParameters.
* + Required: `CONNECTOR_TYPE`, `CONNECTOR_URL`, `CONNECTOR_CLASS_NAME`, `CONNECTION_URL`.
* + Required for `JDBC``CONNECTOR_TYPE` connections: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + `CUSTOM` - Uses configuration settings contained in a custom connector to read from and write to data stores that are not natively supported by Glue.
*
* `SFTP` is not supported.
*
* For more information about how optional ConnectionProperties are used to configure features in Glue, consult [Glue connection properties](https://docs.aws.amazon.com/glue/latest/dg/connection-defining.html).
*
* For more information about how optional ConnectionProperties are used to configure features in Glue Studio, consult [Using connectors and connections](https://docs.aws.amazon.com/glue/latest/ug/connectors-chapter.html).
*/
public val connectionType: aws.sdk.kotlin.services.glue.model.ConnectionType = requireNotNull(builder.connectionType) { "A non-null value must be provided for connectionType" }
/**
* The description of the connection.
*/
public val description: kotlin.String? = builder.description
/**
* A list of criteria that can be used in selecting this connection.
*/
public val matchCriteria: List? = builder.matchCriteria
/**
* The name of the connection.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The physical connection requirements, such as virtual private cloud (VPC) and `SecurityGroup`, that are needed to successfully make this connection.
*/
public val physicalConnectionRequirements: aws.sdk.kotlin.services.glue.model.PhysicalConnectionRequirements? = builder.physicalConnectionRequirements
/**
* A flag to validate the credentials during create connection. Used for a Salesforce connection. Default is true.
*/
public val validateCredentials: kotlin.Boolean = builder.validateCredentials
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.glue.model.ConnectionInput = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConnectionInput(")
append("authenticationConfiguration=$authenticationConfiguration,")
append("connectionProperties=$connectionProperties,")
append("connectionType=$connectionType,")
append("description=$description,")
append("matchCriteria=$matchCriteria,")
append("name=$name,")
append("physicalConnectionRequirements=$physicalConnectionRequirements,")
append("validateCredentials=$validateCredentials")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = authenticationConfiguration?.hashCode() ?: 0
result = 31 * result + (connectionProperties.hashCode())
result = 31 * result + (connectionType.hashCode())
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (matchCriteria?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (physicalConnectionRequirements?.hashCode() ?: 0)
result = 31 * result + (validateCredentials.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConnectionInput
if (authenticationConfiguration != other.authenticationConfiguration) return false
if (connectionProperties != other.connectionProperties) return false
if (connectionType != other.connectionType) return false
if (description != other.description) return false
if (matchCriteria != other.matchCriteria) return false
if (name != other.name) return false
if (physicalConnectionRequirements != other.physicalConnectionRequirements) return false
if (validateCredentials != other.validateCredentials) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.glue.model.ConnectionInput = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The authentication properties of the connection. Used for a Salesforce connection.
*/
public var authenticationConfiguration: aws.sdk.kotlin.services.glue.model.AuthenticationConfigurationInput? = null
/**
* These key-value pairs define parameters for the connection.
*/
public var connectionProperties: Map? = null
/**
* The type of the connection. Currently, these types are supported:
* + `JDBC` - Designates a connection to a database through Java Database Connectivity (JDBC).`JDBC` Connections use the following ConnectionParameters.
* + Required: All of (`HOST`, `PORT`, `JDBC_ENGINE`) or `JDBC_CONNECTION_URL`.
* + Required: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + Optional: `JDBC_ENFORCE_SSL`, `CUSTOM_JDBC_CERT`, `CUSTOM_JDBC_CERT_STRING`, `SKIP_CUSTOM_JDBC_CERT_VALIDATION`. These parameters are used to configure SSL with JDBC.
* + `KAFKA` - Designates a connection to an Apache Kafka streaming platform.`KAFKA` Connections use the following ConnectionParameters.
* + Required: `KAFKA_BOOTSTRAP_SERVERS`.
* + Optional: `KAFKA_SSL_ENABLED`, `KAFKA_CUSTOM_CERT`, `KAFKA_SKIP_CUSTOM_CERT_VALIDATION`. These parameters are used to configure SSL with `KAFKA`.
* + Optional: `KAFKA_CLIENT_KEYSTORE`, `KAFKA_CLIENT_KEYSTORE_PASSWORD`, `KAFKA_CLIENT_KEY_PASSWORD`, `ENCRYPTED_KAFKA_CLIENT_KEYSTORE_PASSWORD`, `ENCRYPTED_KAFKA_CLIENT_KEY_PASSWORD`. These parameters are used to configure TLS client configuration with SSL in `KAFKA`.
* + Optional: `KAFKA_SASL_MECHANISM`. Can be specified as `SCRAM-SHA-512`, `GSSAPI`, or `AWS_MSK_IAM`.
* + Optional: `KAFKA_SASL_SCRAM_USERNAME`, `KAFKA_SASL_SCRAM_PASSWORD`, `ENCRYPTED_KAFKA_SASL_SCRAM_PASSWORD`. These parameters are used to configure SASL/SCRAM-SHA-512 authentication with `KAFKA`.
* + Optional: `KAFKA_SASL_GSSAPI_KEYTAB`, `KAFKA_SASL_GSSAPI_KRB5_CONF`, `KAFKA_SASL_GSSAPI_SERVICE`, `KAFKA_SASL_GSSAPI_PRINCIPAL`. These parameters are used to configure SASL/GSSAPI authentication with `KAFKA`.
* + `MONGODB` - Designates a connection to a MongoDB document database.`MONGODB` Connections use the following ConnectionParameters.
* + Required: `CONNECTION_URL`.
* + Required: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + `SALESFORCE` - Designates a connection to Salesforce using OAuth authencation.
* + Requires the `AuthenticationConfiguration` member to be configured.
* + `VIEW_VALIDATION_REDSHIFT` - Designates a connection used for view validation by Amazon Redshift.
* + `VIEW_VALIDATION_ATHENA` - Designates a connection used for view validation by Amazon Athena.
* + `NETWORK` - Designates a network connection to a data source within an Amazon Virtual Private Cloud environment (Amazon VPC).`NETWORK` Connections do not require ConnectionParameters. Instead, provide a PhysicalConnectionRequirements.
* + `MARKETPLACE` - Uses configuration settings contained in a connector purchased from Amazon Web Services Marketplace to read from and write to data stores that are not natively supported by Glue.`MARKETPLACE` Connections use the following ConnectionParameters.
* + Required: `CONNECTOR_TYPE`, `CONNECTOR_URL`, `CONNECTOR_CLASS_NAME`, `CONNECTION_URL`.
* + Required for `JDBC``CONNECTOR_TYPE` connections: All of (`USERNAME`, `PASSWORD`) or `SECRET_ID`.
* + `CUSTOM` - Uses configuration settings contained in a custom connector to read from and write to data stores that are not natively supported by Glue.
*
* `SFTP` is not supported.
*
* For more information about how optional ConnectionProperties are used to configure features in Glue, consult [Glue connection properties](https://docs.aws.amazon.com/glue/latest/dg/connection-defining.html).
*
* For more information about how optional ConnectionProperties are used to configure features in Glue Studio, consult [Using connectors and connections](https://docs.aws.amazon.com/glue/latest/ug/connectors-chapter.html).
*/
public var connectionType: aws.sdk.kotlin.services.glue.model.ConnectionType? = null
/**
* The description of the connection.
*/
public var description: kotlin.String? = null
/**
* A list of criteria that can be used in selecting this connection.
*/
public var matchCriteria: List? = null
/**
* The name of the connection.
*/
public var name: kotlin.String? = null
/**
* The physical connection requirements, such as virtual private cloud (VPC) and `SecurityGroup`, that are needed to successfully make this connection.
*/
public var physicalConnectionRequirements: aws.sdk.kotlin.services.glue.model.PhysicalConnectionRequirements? = null
/**
* A flag to validate the credentials during create connection. Used for a Salesforce connection. Default is true.
*/
public var validateCredentials: kotlin.Boolean = false
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.glue.model.ConnectionInput) : this() {
this.authenticationConfiguration = x.authenticationConfiguration
this.connectionProperties = x.connectionProperties
this.connectionType = x.connectionType
this.description = x.description
this.matchCriteria = x.matchCriteria
this.name = x.name
this.physicalConnectionRequirements = x.physicalConnectionRequirements
this.validateCredentials = x.validateCredentials
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.glue.model.ConnectionInput = ConnectionInput(this)
/**
* construct an [aws.sdk.kotlin.services.glue.model.AuthenticationConfigurationInput] inside the given [block]
*/
public fun authenticationConfiguration(block: aws.sdk.kotlin.services.glue.model.AuthenticationConfigurationInput.Builder.() -> kotlin.Unit) {
this.authenticationConfiguration = aws.sdk.kotlin.services.glue.model.AuthenticationConfigurationInput.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.glue.model.PhysicalConnectionRequirements] inside the given [block]
*/
public fun physicalConnectionRequirements(block: aws.sdk.kotlin.services.glue.model.PhysicalConnectionRequirements.Builder.() -> kotlin.Unit) {
this.physicalConnectionRequirements = aws.sdk.kotlin.services.glue.model.PhysicalConnectionRequirements.invoke(block)
}
internal fun correctErrors(): Builder {
if (connectionProperties == null) connectionProperties = emptyMap()
if (connectionType == null) connectionType = ConnectionType.SdkUnknown("no value provided")
if (name == null) name = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy